Reducing Cacheable Overhead in Spring
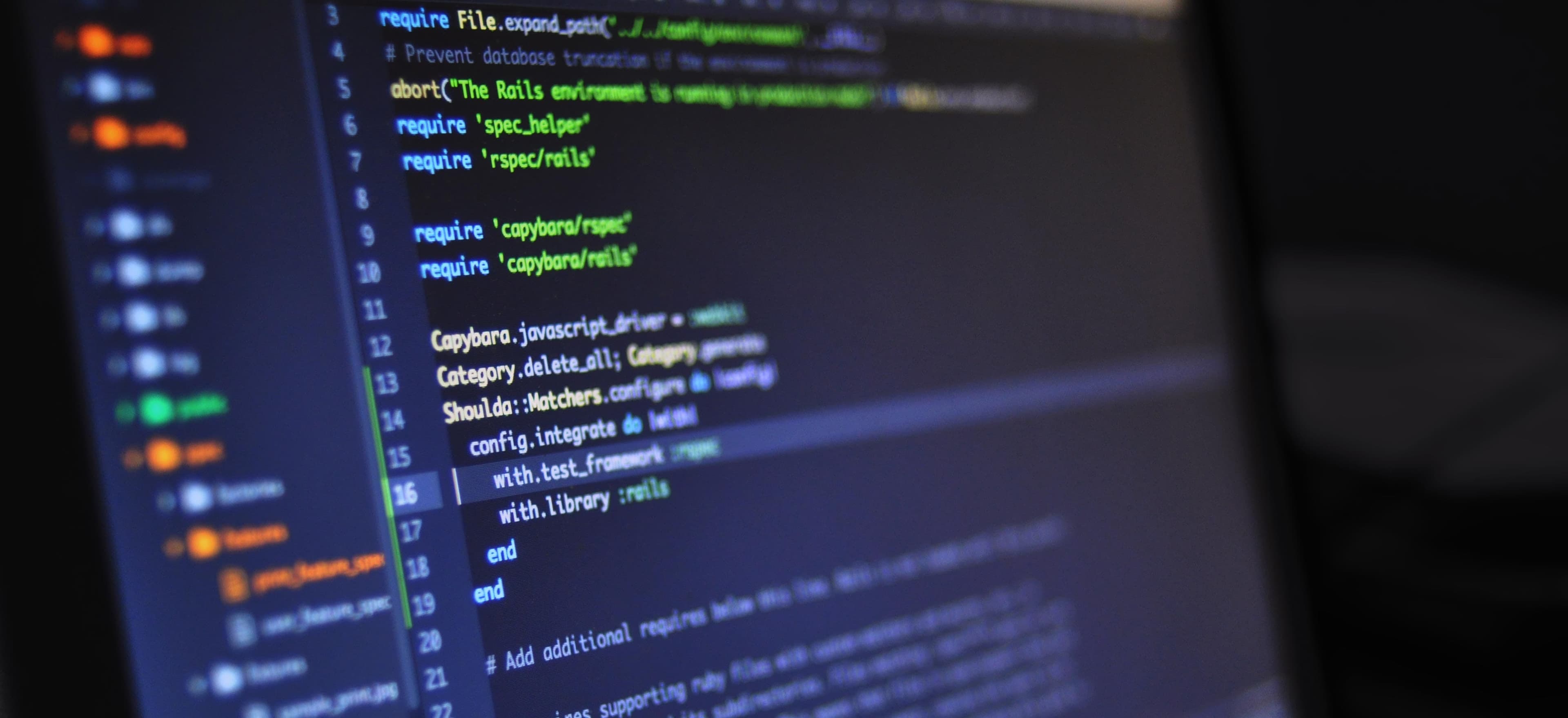
- Published on
Introduction
Caching is a powerful technique that can greatly improve the performance and scalability of your Java applications. By caching frequently accessed data or expensive computations, you can reduce the overhead of repeated calculations and database queries. However, using caching in the wrong way or in the wrong places can lead to unnecessary overhead and potential performance issues. In this article, we will discuss some strategies for reducing cacheable overhead in Spring applications.
Understanding Cacheable Overhead
To understand how to reduce cacheable overhead, we first need to understand what it is. Cacheable overhead refers to the additional processing and memory usage incurred when using caching mechanisms. While caching can provide significant performance benefits, it's important to be aware of the potential overhead that it introduces.
When using caching in a Spring application, the cache framework intercepts method calls and checks if the requested data is already present in the cache. If it is, the cached result is returned instead of executing the method again. This can save significant processing time and reduce the load on the database or other expensive resources. However, this interception and cache lookup process does add some overhead.
The key to reducing cacheable overhead is to minimize the amount of unnecessary data being cached and optimize the cache lookup process. In the following sections, we will discuss some strategies to achieve this.
1. Understand Your Application's Caching Needs
Before implementing caching in your Spring application, it's important to understand the needs of your application and carefully decide which data or computations should be cached. Caching everything may seem like a good idea, but it can lead to excessive memory usage and increased cache lookup overhead.
Start by identifying the parts of your application that can benefit the most from caching. Look for frequently executed methods or expensive computations that are used across multiple requests. By focusing on these areas, you can target the caching efforts where they will have the most impact.
Consider the following factors when deciding what to cache:
- Frequency of access: Cache data or computations that are accessed frequently. If something is rarely accessed, caching it may not provide much benefit.
- Cost of computation or retrieval: If a computation or retrieval of data is expensive, it's a good candidate for caching. Avoid caching lightweight operations that don't have a significant impact on performance.
By carefully selecting what to cache, you can minimize unnecessary cacheable overhead and maximize the benefits of caching.
2. Use Cache Eviction Policies
Cache eviction policies determine when and how items are removed from the cache. By using an appropriate eviction policy, you can avoid caching unnecessary data and reduce the cacheable overhead.
Different eviction policies are available, depending on the caching mechanism you're using. Some common eviction policies include:
- LRU (Least Recently Used): Removes the least recently used items from the cache when it reaches its maximum size.
- LFU (Least Frequently Used): Removes the least frequently used items from the cache when it reaches its maximum size.
- FIFO (First In, First Out): Removes the oldest items from the cache when it reaches its maximum size.
Choose an eviction policy that best suits your application's needs. If you have a high turnover of data, for example, a FIFO policy may be more appropriate. If you have data that is accessed frequently but not recently, an LRU policy may be more effective.
Spring provides support for different caching implementations, such as Ehcache and Caffeine, which offer various eviction policies. Choose the caching implementation and eviction policy that align with your application's requirements.
3. Optimize Cache Key Generation
The cache key is used to identify a specific entry in the cache. It's important to generate an efficient and unique cache key to minimize cacheable overhead and avoid potential clashes.
When generating a cache key, consider the following:
- Use immutable and unique identifiers: If the data being cached has an identifier, such as an ID or a unique combination of fields, use it as part of the cache key. Immutable identifiers are ideal because they won't change over time and won't lead to cache inconsistencies.
- Avoid complex or expensive key generation: Generating the cache key should be fast and lightweight. Avoid using expensive operations or complex logic to generate the key. Simple concatenations or hash calculations are usually sufficient.
- Consider including method arguments: If the cacheable method has arguments that affect the result, include them in the cache key. This ensures that different invocations with different arguments result in separate cache entries.
By optimizing the cache key generation process, you can ensure efficient cache lookup and minimize cacheable overhead.
4. Use Conditional Caching
Conditional caching allows you to cache data or computations only under certain conditions. This can be useful when caching data that might change frequently or when caching expensive computations that are not always needed.
Spring provides support for conditional caching through the @CachePut
and @Cacheable
annotations. By adding additional parameters or expressions to these annotations, you can control when the caching should occur.
For example, consider a method that retrieves user details based on their username. If the details are expensive to compute and the user's data can change frequently, you can use conditional caching to only cache the result for a limited period or if the data has not changed.
@Cacheable(value = "userDetails", key = "#username", condition = "!T(java.time.LocalDateTime).now().minusMinutes(5).isBefore(#result.lastUpdated)")
public UserDetails getUserDetails(String username) {
// Compute user details and return
}
In the above example, the getUserDetails
method is only cached if the result's last updated timestamp is not within the last 5 minutes. This ensures that if the user's data changes frequently, the cache is not stale and unnecessary cacheable overhead is avoided.
By using conditional caching, you can fine-tune the caching behavior and minimize cacheable overhead.
Conclusion
Caching is a powerful technique for improving the performance and scalability of your Spring applications. However, it's important to be aware of the potential cacheable overhead that caching introduces. By understanding your application's caching needs, using appropriate cache eviction policies, optimizing cache key generation, and using conditional caching, you can reduce unnecessary cacheable overhead and maximize the benefits of caching.
Remember to consistently monitor and analyze the performance of your caching implementation to ensure that it's delivering the expected benefits and not causing any performance issues.
By following these strategies, you can effectively use caching in your Spring applications and achieve optimal performance and scalability.
Additional Resources
Checkout our other articles