Converting DateTime with Different Formats in Spring 4
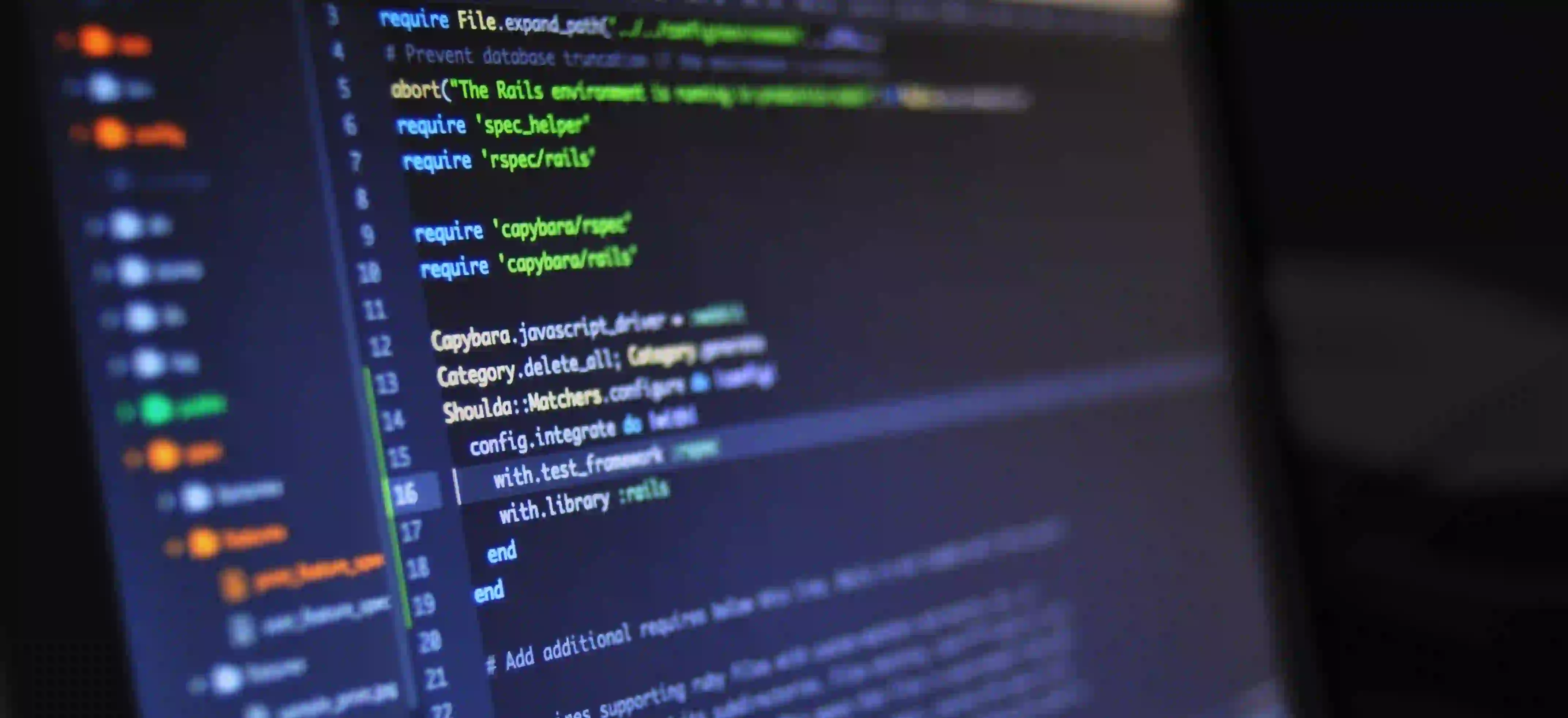
In today's fast-paced digital world, handling date and time is an essential part of any software development project. Whether you are building a web application, a mobile app, or any other software, there will always be a need to work with date and time values.
In this article, we will explore how to convert DateTime values with different formats in a Spring 4 application. Spring 4 provides built-in support for handling date and time, making it easy and convenient to work with DateTime values.
DateTime Conversion in Java
Before we dive into the specifics of datetime conversion in Spring 4, let's quickly review datetime conversion in Java. In Java, the core API for handling date and time is the java.util.Date
class, which represents a specific point in time. However, working directly with java.util.Date
can be cumbersome and error-prone, especially when dealing with different formats.
To overcome the limitations of java.util.Date
, Java 8 introduced the new date and time API. This new API, commonly known as the Java Time API, provides classes like LocalDate
, LocalTime
, and LocalDateTime
that make it easier to handle date and time values. The Java Time API also provides a powerful DateTimeFormatter
class, which allows for easy conversion between different date and time formats.
Spring 4 DateTime Conversion
Spring 4 builds upon the Java Time API and provides additional support for datetime conversion. The org.springframework.format.datetime.DateTimeFormatterFactoryBean
class is a utility class in Spring 4 that allows you to create custom DateTimeFormatter
instances. These custom formatters can then be used to convert DateTime values with different formats.
To use the DateTimeFormatterFactoryBean
, you need to configure it as a bean in your Spring application context. Here's an example configuration:
<bean id="myDateFormat" class="org.springframework.format.datetime.DateTimeFormatterFactoryBean">
<property name="pattern" value="dd-MM-yyyy" />
</bean>
In this example, we have defined a bean named myDateFormat
of type DateTimeFormatterFactoryBean
. We have also configured the pattern
property of the bean to specify the desired date format (in this case, "dd-MM-yyyy").
Once you have defined the DateTimeFormatter
bean, you can use it to convert DateTime values in your Spring 4 application. To do this, you can make use of the @DateTimeFormat
annotation provided by Spring.
public class MyController {
@RequestMapping("/date")
public String handleDate(@RequestParam("date") @DateTimeFormat(pattern="dd-MM-yyyy") LocalDate date) {
// Handle the date
// ...
}
}
In this example, we have a Spring MVC controller method that handles a GET request to "/date". The @RequestParam
annotation is used to bind the value of the "date" request parameter to a LocalDate
parameter in the method. The @DateTimeFormat
annotation is used to specify the expected date format.
When a request is made to this controller method with a valid date value in the specified format, Spring will automatically convert the string value to a LocalDate
object using the configured DateTimeFormatter
. If the date value is not in the expected format, Spring will throw a MismatchedInputException
exception.
In addition to the @DateTimeFormat
annotation, Spring 4 also provides the @JsonFormat
annotation for handling JSON serialization and deserialization of DateTime values. You can use this annotation to specify the date format in which the DateTime value should be serialized or deserialized.
public class MyObject {
@JsonFormat(pattern="yyyy-MM-dd")
private LocalDate date;
// Getters and setters
}
In this example, the @JsonFormat
annotation is applied to a LocalDate
field in a POJO class. This annotation tells the Jackson JSON library to serialize and deserialize the date
field in the specified format.
Conclusion
In this article, we have explored how to convert DateTime values with different formats in a Spring 4 application. We have learned how to configure a DateTimeFormatter
bean using the DateTimeFormatterFactoryBean
class, and how to use this bean for datetime conversion in Spring MVC controller methods.
By leveraging the power of the Java Time API and Spring 4's datetime conversion support, you can easily handle date and time values in your Spring applications. This not only simplifies your code but also helps in ensuring consistent and accurate handling of date and time across your application.
To learn more about DateTime conversion in Spring 4, you can refer to the official Spring documentation: