Choosing the Right Automation Testing Tool: A Beginner's Dilemma
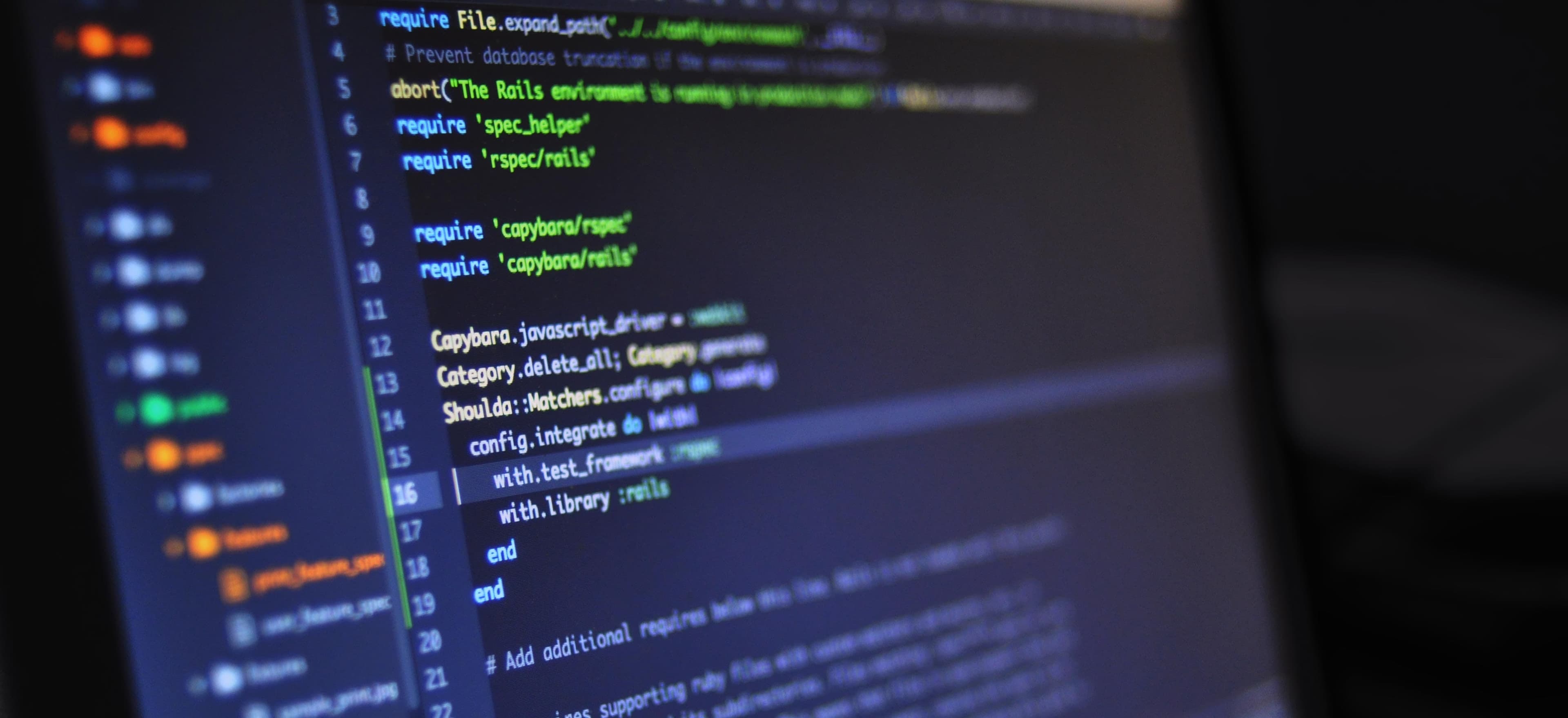
- Published on
Choosing the Right Automation Testing Tool: A Beginner's Dilemma
As a beginner in automation testing, it can be overwhelming to choose the right tool for your testing needs. With so many options available, each claiming to be the best, how do you decide which one is right for you? In this article, we will discuss some important factors to consider when choosing an automation testing tool and highlight a few popular ones in the industry.
Factors to Consider
1. Type of Application Under Test
The first factor to consider is the type of application you will be testing. Different tools are better suited for testing different types of applications. For example, if you are testing a web application, tools like Selenium WebDriver or Cypress might be a good choice. On the other hand, if you are testing a mobile application, you might consider tools like Appium or Espresso.
2. Programming Language
Most automation testing tools support multiple programming languages. It is important to choose a tool that supports a language you are comfortable with or eager to learn. If you are already familiar with Java, you might consider tools like Selenium WebDriver or JUnit, as they have good support for Java.
3. Community Support
Having a strong community support is crucial when choosing an automation testing tool. A vibrant community means there are plenty of resources available such as tutorials, forums, and online communities where you can get help and learn from others. This can greatly speed up your learning process and help you troubleshoot any problems you encounter. Tools like Selenium WebDriver, JUnit, and TestNG have large and active communities.
4. Integration with Other Tools
Consider the integration options the automation testing tool offers. Does it integrate well with your existing tools and technologies? For example, if you are using a Continuous Integration (CI) tool like Jenkins or Bamboo, you might want to choose a testing tool that has built-in support for these CI systems. This will allow you to seamlessly integrate your tests into your CI pipeline.
5. Reporting and Analytics
Good reporting and analytics capabilities are essential for automation testing. Look for a tool that provides detailed and actionable reports. This will help you identify issues and track the progress of your tests. Tools like TestNG and JUnit provide rich reporting features that can be integrated with various reporting plugins.
6. Cost and Licensing
Consider the cost and licensing requirements of the tool. Some tools are free and open source, while others have a commercial license and require a subscription. Make sure you understand the licensing terms and evaluate whether the cost is justified by the features and benefits the tool provides.
Popular Automation Testing Tools
1. Selenium WebDriver
Selenium WebDriver is one of the most popular automation testing tools in the industry. It supports various programming languages, including Java, and is widely used for web application testing. Selenium WebDriver allows you to write tests in a simple and concise way, and it integrates well with other tools and frameworks.
Here's an example of a Selenium WebDriver test written in Java:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyTest {
public static void main(String[] args) {
// Set the path to the chromedriver executable
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
// Create a new instance of the WebDriver
WebDriver driver = new ChromeDriver();
// Navigate to a URL
driver.get("https://www.example.com");
// Perform actions on the page
// ...
// Close the browser
driver.quit();
}
}
2. JUnit
JUnit is a popular unit testing framework for Java. While primarily used for unit testing, it can also be used for automation testing. JUnit provides a simple and intuitive API for writing tests and supports advanced features such as parameterized tests and test suites. It integrates well with build tools like Maven and Gradle, making it easy to incorporate your tests into your development workflow.
Here's an example of a JUnit test written in Java:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyTest {
@Test
public void testAddition() {
int result = 2 + 2;
assertEquals(4, result);
}
}
3. TestNG
TestNG is another popular testing framework for Java. It provides powerful features for test configuration, including support for data-driven testing, parallel execution, and test dependencies. TestNG is known for its rich reporting capabilities and integrates well with other tools and frameworks, such as Selenium WebDriver and Jenkins.
Here's an example of a TestNG test written in Java:
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class MyTest {
@Test
public void testAddition() {
int result = 2 + 2;
assertEquals(4, result);
}
}
Conclusion
Choosing the right automation testing tool can be challenging, especially for beginners. By considering factors such as the type of application, programming language, community support, integration options, reporting capabilities, and cost, you can make an informed decision. Selenium WebDriver, JUnit, and TestNG are popular choices in the industry, but there are many other tools available as well. Take the time to evaluate and experiment with different tools to find the one that best suits your needs and preferences. Happy testing!
Checkout our other articles