Bean Configuration Not Found: Resolving ClasspathXMLApplicationContext Issue
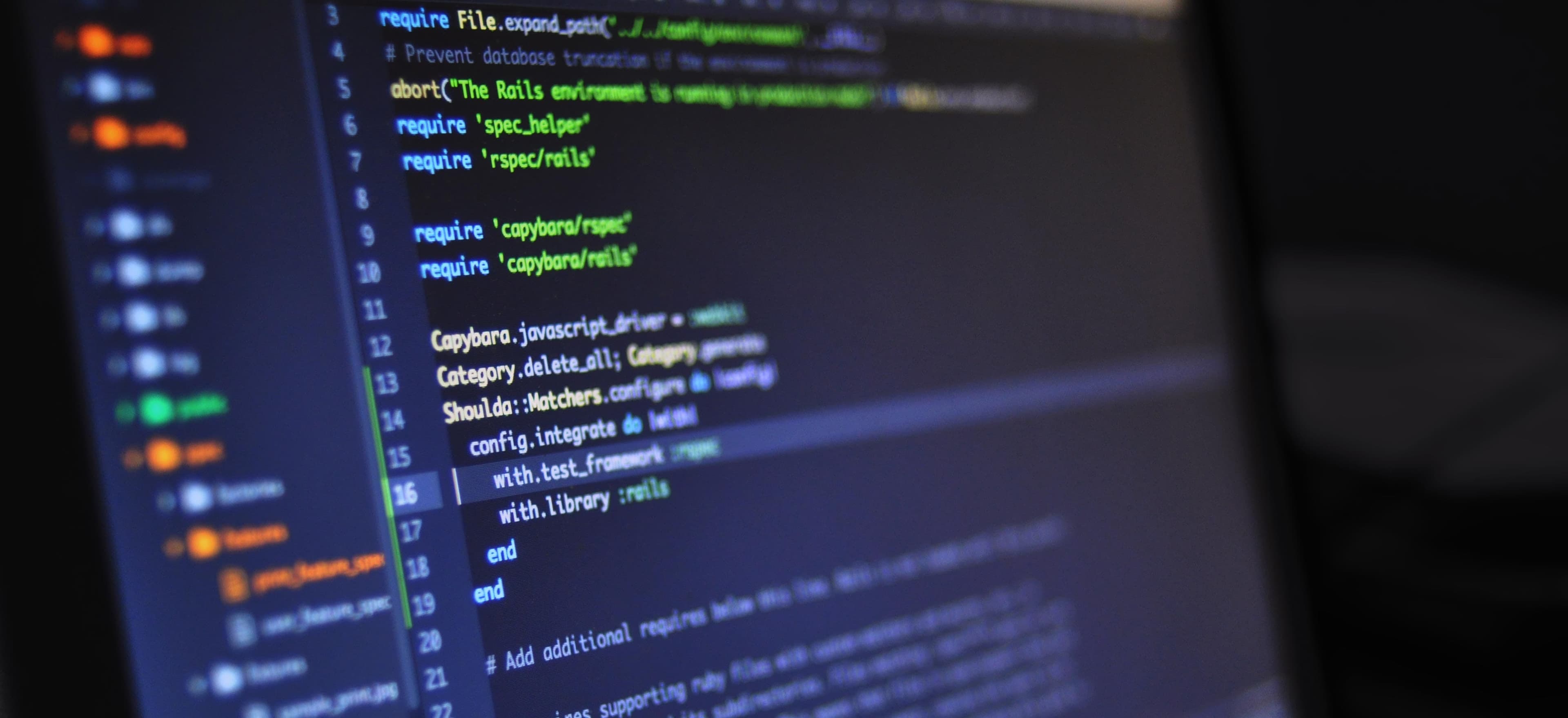
- Published on
Bean Configuration Not Found: Resolving ClasspathXMLApplicationContext Issue
Introduction
In Java development, it is common to use the Spring framework for dependency injection and managing application contexts. One of the ways to configure the Spring application context is through XML configuration files. However, there may be cases where the ClasspathXMLApplicationContext is unable to find the bean configuration file, resulting in an error. In this article, we will explore the possible causes of this issue and discuss ways to resolve it.
Understanding the ClasspathXMLApplicationContext
Before diving into the issue, let's first understand what the ClasspathXMLApplicationContext is. This class is part of the Spring framework and is used to load the bean definitions from an XML file located in the classpath. It creates and manages the Spring application context based on the configuration provided in the XML file.
Possible Causes of the Issue
There can be several reasons why the ClasspathXMLApplicationContext is unable to find the bean configuration file. Let's explore some of the common causes:
1. Incorrect File Path
The most common cause is an incorrect file path specified in the ClasspathXMLApplicationContext constructor. Make sure that the file path is correct and that the XML file is located in the classpath. Remember that the classpath includes all the directories and JAR files available to the application at runtime.
2. File Name Mismatch
Another possible cause is a mismatch between the file name specified in the ClasspathXMLApplicationContext constructor and the actual file name of the XML configuration. Ensure that the file name specified is exactly the same as the XML file name, including the file extension.
3. File Not Included in Classpath
If the XML configuration file is not included in the classpath, the ClasspathXMLApplicationContext will not be able to find it. Check that the file is included in the classpath either by placing it in the correct directory or by adding it to the classpath explicitly.
4. File Encoding Issue
Sometimes, the bean configuration file may have an encoding issue, resulting in the ClasspathXMLApplicationContext not being able to read it. Ensure that the XML file is encoded correctly, especially if it contains special characters or symbols.
5. File Loading Order
The order in which files are loaded by the ClasspathXMLApplicationContext can also cause issues. If multiple XML configuration files are present in the classpath, ensure that the required file is loaded first. This can be controlled by specifying the order of the files in the classpath.
Resolving the Issue
Now that we have identified some of the possible causes of the issue, let's discuss how to resolve it. Here are some steps you can take to troubleshoot and fix the problem:
1. Verify the File Path
Double-check the file path specified in the ClasspathXMLApplicationContext constructor and ensure that it is correct. If needed, provide the absolute path to the file to eliminate any confusion.
2. Confirm the File Name
Compare the file name specified in the constructor with the actual file name of the XML configuration file. Make sure that it is an exact match, including any file extensions.
3. Check Classpath Inclusion
If the XML configuration file is not included in the classpath, add it to the classpath explicitly. This can be done by using the correct directory structure or by configuring the classpath in your build tool (e.g. Maven or Gradle).
4. Verify File Encoding
Ensure that the XML configuration file is encoded correctly. If you suspect an encoding issue, you can try opening and saving the file with a different encoding. UTF-8 is usually a safe choice for XML files.
5. Control File Loading Order
If there are multiple XML configuration files in the classpath, consider specifying the order in which they should be loaded. This can be done by either renaming the files to control the alphabetical order or by explicitly specifying the order in your application configuration.
Example Code Snippet
To illustrate the usage of ClasspathXMLApplicationContext and address the issue, here is a code snippet:
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyApp {
public static void main(String[] args) {
// Create the application context
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
// Retrieve a bean from the context
MyBean myBean = context.getBean("myBean", MyBean.class);
// Use the bean
myBean.doSomething();
// Close the application context
context.close();
}
}
In the above code, we create an instance of ClassPathXmlApplicationContext and specify the file name of the XML configuration ("applicationContext.xml"). Make sure that the file is present in the classpath and contains the appropriate bean definitions.
Conclusion
When encountering the "Bean Configuration Not Found" issue with the ClasspathXMLApplicationContext, it is important to verify the file path, file name, classpath inclusion, file encoding, and file loading order. By following the steps outlined in this article, you should be able to resolve the issue and successfully load the bean configuration file. Remember to carefully review your code and configuration to ensure their correctness.
Checkout our other articles