Monitoring OpenJDK Performance from the Command Line
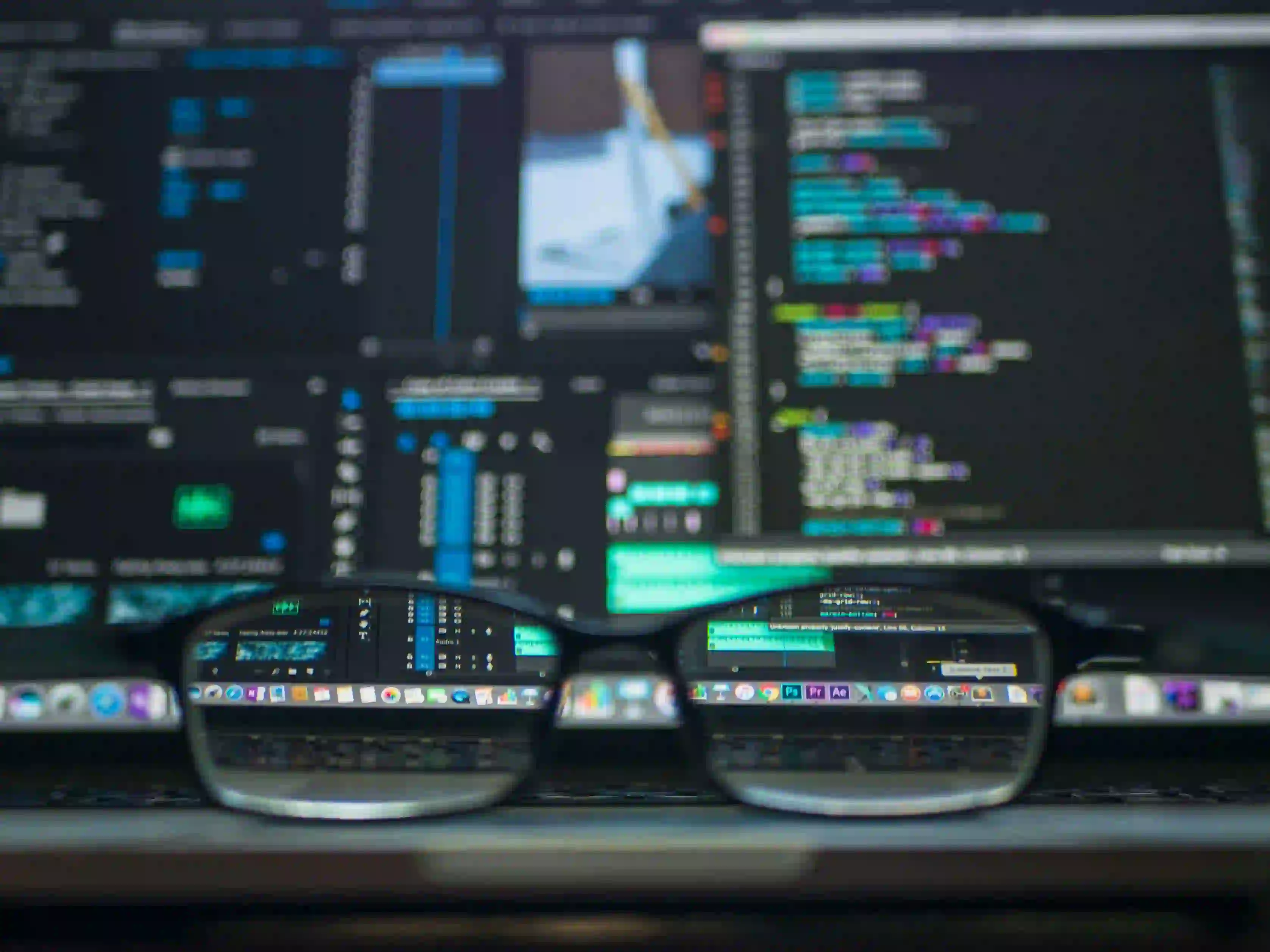
Monitoring OpenJDK Performance from the Command Line
As a Java developer, it's crucial to have an understanding of the performance characteristics of your application running on the OpenJDK. Monitoring the performance of your Java application allows you to identify bottlenecks, optimize code, and improve the overall experience for your users. In this article, we will explore how to monitor OpenJDK performance from the command line using several useful tools.
1. The jstat
Tool
The jstat
tool is a command-line utility that allows you to monitor various statistics about a running Java application. It provides real-time monitoring of Java Virtual Machine (JVM) memory, garbage collection, and thread usage. Here's how you can use it:
-
Open a terminal or command prompt.
-
Navigate to the directory where the Java application is running.
-
Run the following command:
jstat -<option> <pid> <interval> <count>
<option>
: The option specifies the statistics you want to monitor. Some commonly used options are:gc
: Garbage collection statistics.gcutil
: Garbage collection utilization statistics.gccapacity
: Garbage collection heap capacity statistics.gcnew
: New generation garbage collection statistics.
<pid>
: The process ID of the running Java application. You can find this using tools likejps
orps
.<interval>
: The interval in milliseconds between each data collection.<count>
: The number of data collections to perform.
For example, to monitor garbage collection statistics of a Java application with PID 12345 every 1 second for 10 collections, you would run the following command:
jstat -gc 12345 1000 10
The output will display information about the garbage collection, including the time spent in different garbage collection phases and the number of objects collected.
2. The jcmd
Tool
The jcmd
tool is a versatile command-line utility that allows you to interact with a running Java application. It provides a wide range of diagnostic and troubleshooting commands, including performance monitoring. Here's how you can use it:
-
Open a terminal or command prompt.
-
Navigate to the directory where the Java application is running.
-
Run the following command:
jcmd <pid> <command> [options]
<pid>
: The process ID of the running Java application.<command>
: The command you want to execute. Some commonly used commands for performance monitoring are:VM.flags
: Prints VM flags for the running Java application.VM.system_properties
: Prints system properties for the running Java application.GC.class_histogram
: Prints a histogram of the number of instances of each class.Thread.print
: Prints a stack trace of all threads.
[options]
: Additional options specific to the command.
For example, to print the system properties of a Java application with PID 12345, you would run the following command:
jcmd 12345 VM.system_properties
The output will display the system properties, including information about the Java version, operating system, and JVM settings.
3. The jstack
Tool
The jstack
tool allows you to capture the stack trace of a Java application's threads. It is useful for diagnosing performance issues related to thread synchronization, deadlock, or high CPU usage. Here's how you can use it:
-
Open a terminal or command prompt.
-
Navigate to the directory where the Java application is running.
-
Run the following command:
jstack <pid>
<pid>
: The process ID of the running Java application.
For example, to capture the stack trace of a Java application with PID 12345, you would run the following command:
jstack 12345
The output will display the stack trace of all threads in the Java application, including information about the classes and methods being executed.
4. The jconsole
Tool
The jconsole
tool is a graphical user interface (GUI) application that allows you to monitor and manage a running Java application. It provides real-time performance monitoring, including CPU usage, memory usage, and thread activity. Here's how you can use it:
-
Open a terminal or command prompt.
-
Run the following command:
jconsole
This will launch the
jconsole
application. -
In the
jconsole
application, select the process ID of the running Java application from the list of available processes. -
Click on the "Connect" button to connect to the selected Java application.
Once connected, you can navigate through the different tabs in the jconsole
application to view various performance statistics of the Java application, such as memory usage, CPU usage, and thread activity.
Conclusion
Monitoring the performance of your Java application running on the OpenJDK is essential for optimizing its performance and ensuring a smooth user experience. In this article, we explored several command-line tools that allow you to monitor various aspects of your Java application, including memory usage, garbage collection, thread activity, and more. By using these tools, you can identify and address performance issues in your Java applications effectively.