Fighting Type Erasure: Unveiling the Limitations of Java Generics
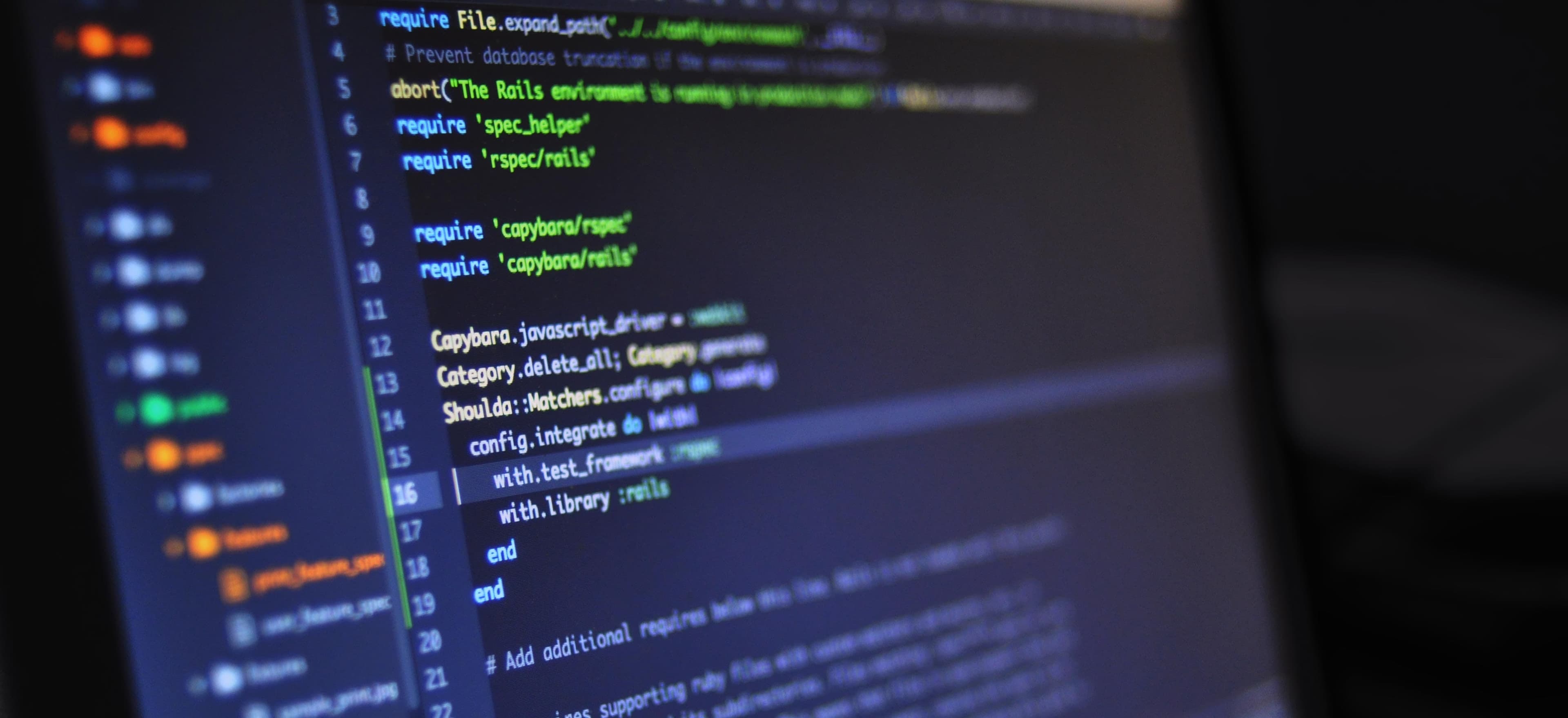
- Published on
Fighting Type Erasure: Unveiling the Limitations of Java Generics
Introduction
Java generics were introduced in Java 5 with the goal of providing type safety and eliminating the need for casting. Generics allow developers to create abstract data types that can work with different types of objects while ensuring type safety and eliminating the risk of runtime errors.
However, despite their many advantages, Java generics have some limitations. One of the major limitations is type erasure, which is a process by which generic type information is erased at compile time, making it unavailable at runtime. This can lead to some unexpected behavior and limitations when using generics in Java.
In this article, we will explore the concept of type erasure in Java generics, understand its limitations, and explore some workarounds to overcome these limitations.
Understanding Type Erasure
Type erasure is a process in Java where the compiler removes the generic type information and replaces it with the bounds or Object type. This means that the generic type information is not available at runtime, and all generic types are effectively treated as Object types.
For example, consider the following generic class:
public class Box<T> {
private T content;
public void add(T item) {
this.content = item;
}
public T get() {
return this.content;
}
}
At runtime, the generic type T
is erased, and the code is effectively compiled as if it were:
public class Box {
private Object content;
public void add(Object item) {
this.content = item;
}
public Object get() {
return this.content;
}
}
This means that any generic type information used within the class, such as method signatures or field types, is lost at runtime.
Limitations of Type Erasure
Due to type erasure, Java generics suffer from some limitations. Let's explore some of these limitations:
Inability to Instantiate Generic Types
One of the major limitations of type erasure is the inability to instantiate generic types directly. Since the generic type information is erased at runtime, it is not possible to create an instance of a generic type using the new
keyword.
For example, consider the following code:
public class MyClass<T> {
public T createInstance() {
return new T(); // Error: Cannot instantiate the type T
}
}
Here, the code will fail with a compilation error because the generic type T
is erased at runtime, and the new T()
expression is not valid.
To work around this limitation, you can pass an instance of Class<T>
as a constructor parameter and use reflection to create a new instance. Here's an example:
public class MyClass<T> {
private Class<T> type;
public MyClass(Class<T> type) {
this.type = type;
}
public T createInstance() throws IllegalAccessException, InstantiationException {
return type.newInstance();
}
}
Inability to Perform Certain Operations on Generic Types
Another limitation of type erasure is the inability to perform certain operations on generic types. Since the generic type information is not available at runtime, it is not possible to directly perform operations that depend on the specific type of the generic.
For example, consider the following code:
public class GenericProperty<T> {
private T value;
public void setValue(T value) {
this.value = value;
}
public T getValue() {
return this.value;
}
public void printType() {
System.out.println(T.class); // Error: Cannot use 'T' here
}
}
Here, the code will fail with a compilation error because the generic type T
is erased at runtime, and the T.class
expression is not valid.
To work around this limitation, you can pass the Class<T>
as a constructor parameter and store it as an instance field. Then, you can use this stored Class<T>
to perform operations that depend on the specific type of the generic. Here's an example:
public class GenericProperty<T> {
private T value;
private Class<T> type;
public GenericProperty(Class<T> type) {
this.type = type;
}
public void setValue(T value) {
this.value = value;
}
public T getValue() {
return this.value;
}
public void printType() {
System.out.println(type);
}
}
Inability to Perform Runtime Type Checks
One more limitation of type erasure is the inability to perform runtime type checks on generic types. Since the generic type information is erased at runtime, it is not possible to check whether an object is an instance of a specific generic type.
For example, consider the following code:
public class MyClass<T> {
public void doSomething(T item) {
if (item instanceof T) { // Error: Cannot perform instanceof check on erased type
System.out.println("It's an instance of T");
}
}
}
Here, the code will fail with a compilation error because the instanceof
operator cannot be used with erased types.
To work around this limitation, you can pass the Class<T>
as a constructor parameter and use the isInstance()
method of the Class
class to perform type checks at runtime. Here's an example:
public class MyClass<T> {
private Class<T> type;
public MyClass(Class<T> type) {
this.type = type;
}
public void doSomething(Object item) {
if (type.isInstance(item)) {
System.out.println("It's an instance of T");
}
}
}
Conclusion
Java generics provide a way to create type-safe and reusable code. However, they have some limitations due to type erasure. Type erasure can make it difficult to work with generic types at runtime.
In this article, we explored the concept of type erasure in Java generics and discussed its limitations. We also explored some workarounds to overcome these limitations, such as using reflection and storing Class<T>
as an instance field.
By understanding the limitations of type erasure, you can write more effective and robust code when working with Java generics.
Checkout our other articles