Overcoming Common Challenges in Android Developer Interviews
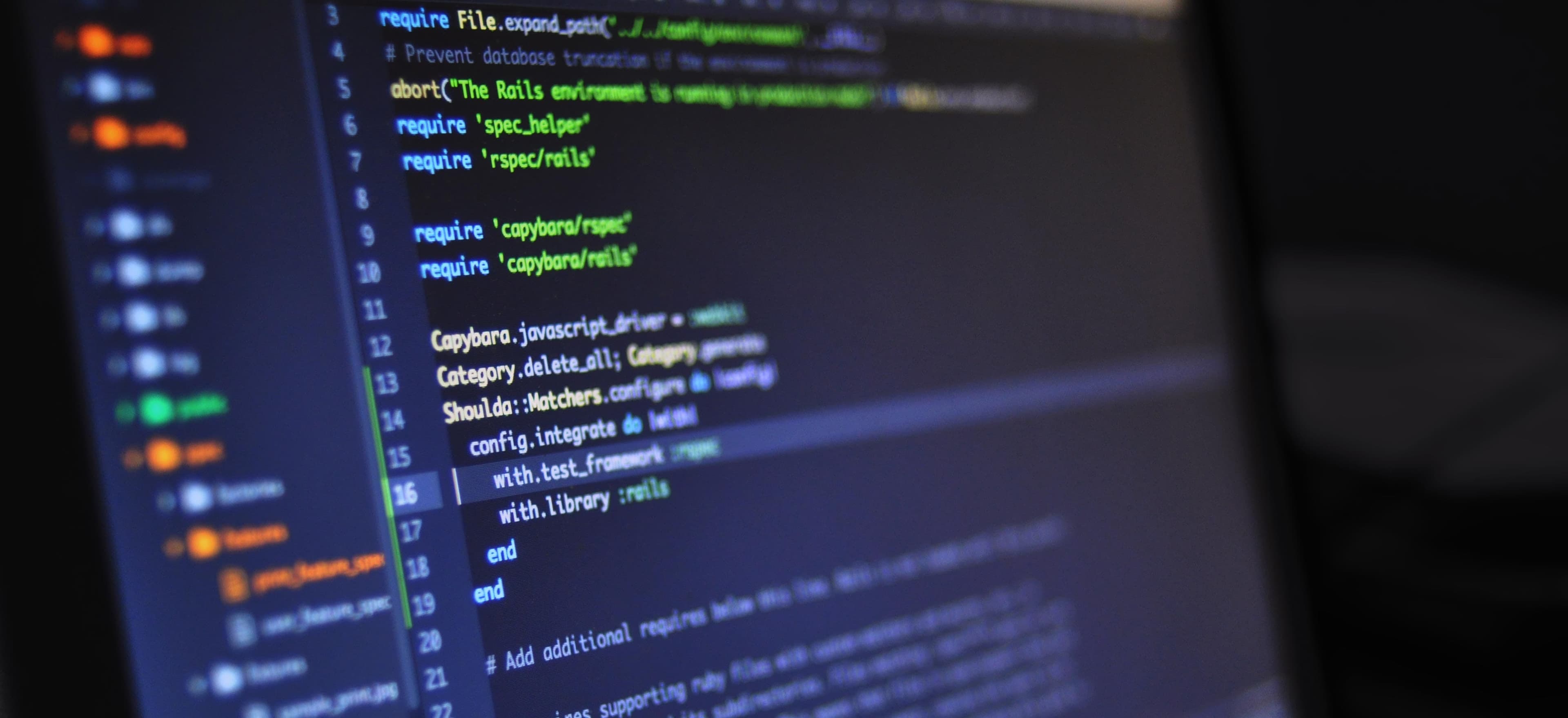
- Published on
Introduction
Interviews can be a nerve-wracking experience for any job seeker, especially in the field of Android development. As an Android developer, you'll likely encounter a variety of challenges during interviews that will test your knowledge and abilities. However, with proper preparation and a clear understanding of common challenges, you can overcome these obstacles and impress potential employers. In this article, we will discuss some of the most common challenges faced by Android developers during interviews and provide tips on how to tackle them.
Understanding Android Architecture
One of the most important aspects of being an Android developer is having a solid understanding of the Android architecture. Interviewers often test candidates on their knowledge of Android components, such as activities, services, content providers, and broadcast receivers. They may also ask about the Activity lifecycle and how to handle configuration changes.
To demonstrate your expertise in this area, it is essential to have hands-on experience working with these components. Be prepared to explain how they interact with each other and how they fit into the overall architecture of an Android application.
Here's an example of how you can explain the Activity lifecycle:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onStart() {
super.onStart();
// Called when the activity is about to become visible
}
@Override
protected void onResume() {
super.onResume();
// Called when the activity has become visible (it is now "resumed")
}
@Override
protected void onPause() {
super.onPause();
// Called when another activity is taking focus
}
@Override
protected void onStop() {
super.onStop();
// Called when the activity is no longer visible
}
@Override
protected void onDestroy() {
super.onDestroy();
// Called before the activity is destroyed
}
}
In the code snippet above, we have implemented the Activity
class and overridden several lifecycle methods. Use code comments to explain the purpose of each method and when it is called. This shows that you not only understand the code but also know the reasons behind it.
Knowledge of Design Patterns
Design patterns play a significant role in Android development. Interviewers often ask questions about design patterns to assess candidates' software engineering skills and their ability to write clean, maintainable code.
Some common design patterns used in Android development include:
1. Model-View-Controller (MVC)
The MVC pattern separates an application into three main components: the Model, the View, and the Controller. The Model represents the data and business logic, the View handles the user interface, and the Controller acts as an intermediary between the Model and the View.
2. Model-View-ViewModel (MVVM)
MVVM is another popular design pattern in Android development. It separates the UI code (View) from the business logic (Model) using a ViewModel as an intermediary. MVVM is especially useful when working with data binding frameworks like Android Data Binding or Jetpack Compose.
3. Singleton
Singleton is a creational design pattern that ensures only one instance of a class is created throughout the application. It is often used for creating global objects or managing shared resources.
When discussing design patterns in an interview, be sure to provide examples of how you have implemented them in real-world projects. This demonstrates your practical experience and shows that you understand how design patterns can improve code maintainability and scalability.
Handling Memory Leaks
Memory leaks can be a common problem in Android applications, and interviewers may want to gauge your understanding of how to detect and fix them.
One of the most common causes of memory leaks in Android is when a reference to a context is retained beyond its intended lifetime. To avoid this, it is essential to understand how the Android garbage collector works and how to release resources properly.
Here's an example of a potential memory leak:
public class MainActivity extends AppCompatActivity {
private static MySingleton singleton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
singleton = MySingleton.getInstance(this);
}
// ...
}
public class MySingleton {
private Context context;
private static MySingleton instance;
private MySingleton(Context context) {
this.context = context;
}
public static MySingleton getInstance(Context context) {
if (instance == null) {
instance = new MySingleton(context);
}
return instance;
}
// ...
}
In the code above, the MySingleton
class retains a reference to the Context
object passed during initialization. If the MainActivity
gets destroyed, the reference to the Context
will still exist, leading to a memory leak.
To avoid this, it is recommended to use ApplicationContext
instead of Context
in long-lived objects like singletons. The application context is not tied to the lifecycle of an activity and can help prevent memory leaks.
Version Control Systems
When working in a team, version control systems are essential for managing code changes and collaborating efficiently. Interviewers may ask about your experience with version control systems, such as Git, and how you handle branching, merging, and resolving conflicts.
Having practical knowledge of Git and understanding common Git commands will show your ability to work effectively in a team environment. Be prepared to explain concepts such as repositories, branches, commits, and pull requests.
Here are some common Git commands you should be familiar with:
git init
: Initializes a new Git repository.git clone <repository>
: Clones a repository from a remote server to your local machine.git checkout -b <branch_name>
: Creates a new branch and switches to it.git add <file>
: Adds a file to the staging area.git commit -m "<commit_message>"
: Commits changes with a descriptive message.git push origin <branch_name>
: Pushes changes from your local branch to the remote repository.
Demonstrating your understanding of version control systems and your ability to manage code changes effectively will give interviewers confidence in your ability to work collaboratively.
Writing Efficient Code and Performance Optimization
Efficiency and performance are crucial aspects of Android development. Interviewers may ask questions related to writing efficient code and optimizing application performance.
Here are some tips to keep in mind:
- Use appropriate data structures and algorithms to minimize time and space complexity.
- Avoid redundant code and optimize resource usage.
- Utilize background threads or asynchronous programming for time-consuming operations.
- Profile your application using tools like the Android Profiler to identify performance bottlenecks.
Be prepared to discuss real-life examples where you have optimized code or improved application performance. This demonstrates your ability to write efficient, high-quality code.
Conclusion
Preparing for Android developer interviews requires a deep understanding of Android architecture, design patterns, memory management, version control systems, and performance optimization. By addressing these common challenges and being well-prepared, you can increase your chances of success in an interview. Remember to practice your coding skills, review key concepts, and showcase your passion for Android development. Good luck!