Eliminate cascading failures with Hystrix.
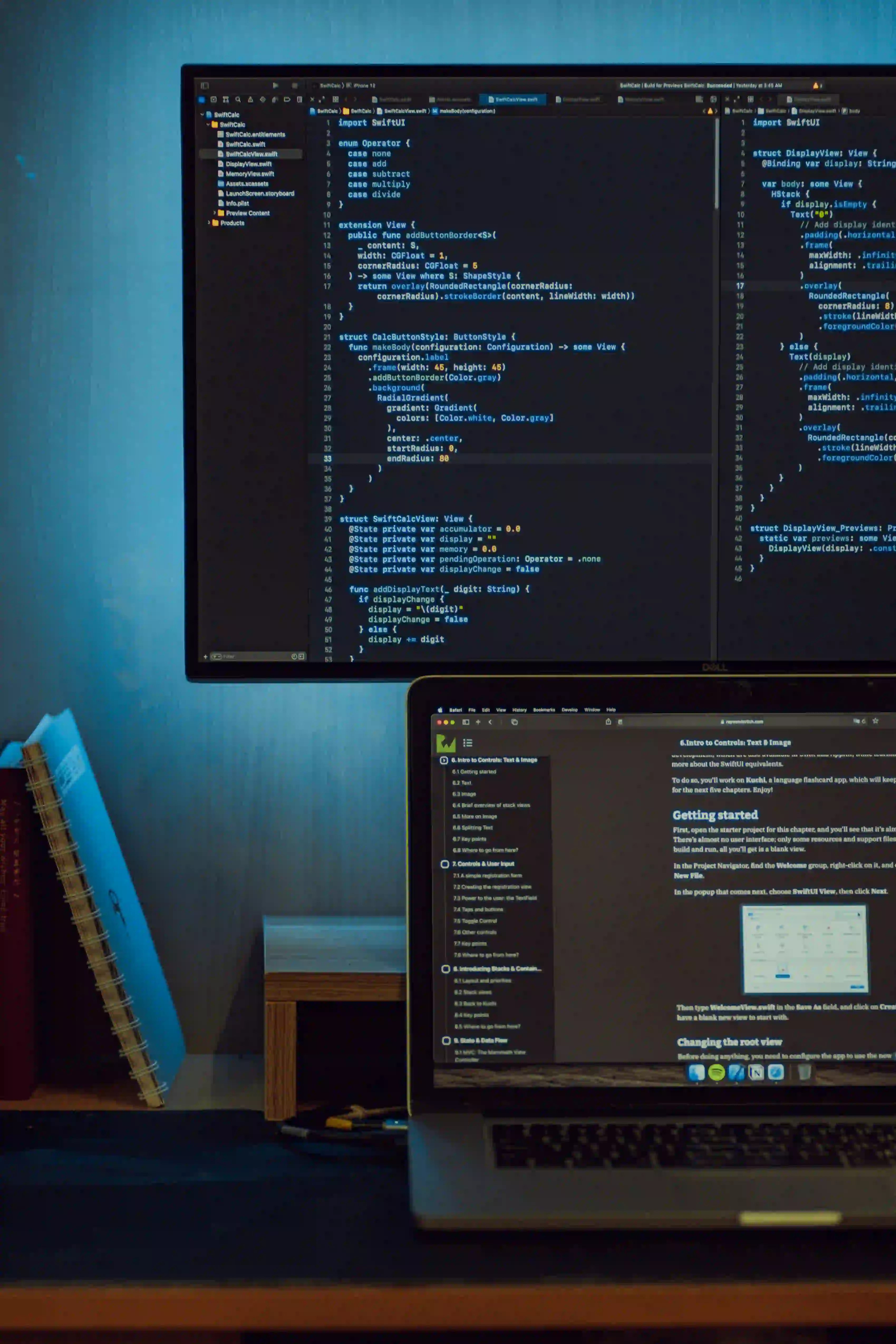
Introduction
In a distributed system, failures are inevitable. When one component fails, it can have a cascading effect, causing all other dependent components to fail as well. This can lead to a complete system outage and result in a poor user experience.
To address the issue of cascading failures, a library called Hystrix was developed by Netflix. Hystrix is a fault tolerance library designed to handle and recover from failures in distributed systems. It provides a solution to eliminate cascading failures and improve the resilience of a system.
In this article, we will explore how Hystrix works and how it can be used to eliminate cascading failures in Java applications.
What is Hystrix?
Hystrix is an open-source library that provides a way to isolate and control interactions between multiple service dependencies. It helps in preventing failures from propagating and causing a complete system outage. Hystrix is designed to handle network latency, failures, and timeouts.
Hystrix is built around the Command pattern, where each interaction with an external service is encapsulated within a HystrixCommand object. This allows for fine-grained control and isolation of each service call.
How does Hystrix work?
Hystrix works by adding a layer between the calling code and the external service. When a service call is made, Hystrix intercepts the request and checks if a circuit breaker is open for that particular service.
A circuit breaker is a design pattern that helps in preventing further requests to a service that is already experiencing failures. When a circuit breaker is open, subsequent requests are not made to the service, and instead, a fallback response or a cached response is returned.
Hystrix monitors the success and failure rate of each service call. If the failure rate exceeds a predefined threshold, the circuit breaker is opened. This prevents further requests from being made to the service, reducing the load on the failing service and allowing it to recover.
When the circuit breaker is open, Hystrix can also execute a fallback function or return a fallback response. This ensures that the calling code receives a response, even if the service is down.
Implementing Hystrix in Java
To implement Hystrix in a Java application, you need to add the Hystrix dependency to your project. You can use a build tool like Maven or Gradle to include the dependency.
Once you have included the Hystrix dependency, you can start using Hystrix in your application. To make a service call using Hystrix, you need to create a class that extends the HystrixCommand class and implement the run()
method. The run()
method contains the code that makes the actual service call.
Here's an example of how to use Hystrix in Java:
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
public class MyServiceCommand extends HystrixCommand<String> {
private final String serviceUrl;
public MyServiceCommand(String serviceUrl) {
super(HystrixCommandGroupKey.Factory.asKey("MyServiceGroup"));
this.serviceUrl = serviceUrl;
}
@Override
protected String run() throws Exception {
// Code to make the service call
// ...
return "Service response";
}
@Override
protected String getFallback() {
// Fallback logic
// ...
return "Fallback response";
}
}
In this example, MyServiceCommand
extends the HystrixCommand
class and overrides the run()
and getFallback()
methods.
To execute the service call, you need to create an instance of MyServiceCommand
and call the execute()
method. Here's an example:
MyServiceCommand command = new MyServiceCommand("http://example.com");
String response = command.execute();
In this example, the execute()
method will make the service call defined in the run()
method of MyServiceCommand
. If the service call fails or times out, the getFallback()
method will be called, and the fallback response will be returned.
Benefits of using Hystrix
Using Hystrix in your Java application provides several benefits:
1. Resilience
Hystrix helps in building resilient systems by isolating and controlling interactions with external services. It prevents failures from propagating and causing a complete system outage. By using circuit breakers and fallback mechanisms, Hystrix ensures that the system remains operational even when services are not available.
2. Performance
Hystrix provides performance optimizations by using techniques like request caching, request collapsing, and thread pooling. It reduces the number of costly and time-consuming service calls and improves the overall performance of the system.
3. Monitoring and Metrics
Hystrix provides built-in monitoring and metrics capabilities. It collects and exposes metrics like success rate, failure rate, and response times for each service call. This helps in identifying and diagnosing issues with external services and allows for proactive monitoring and alerting.
4. Fault Tolerance
Hystrix helps in building fault-tolerant systems by providing fault tolerance patterns like circuit breakers and fallback mechanisms. It ensures that the system remains operational even in the face of failures and reduces the impact of failures on the overall system.
Conclusion
Cascading failures can have a detrimental impact on the performance and availability of a distributed system. Hystrix provides a solution to eliminate cascading failures by isolating interactions with external services and providing fault tolerance mechanisms.
By using Hystrix in your Java applications, you can improve the resilience, performance, and fault tolerance of your system. It helps in preventing failures from propagating and ensures that the system remains operational even in the face of failures.
So, if you want to eliminate cascading failures and build a more robust and resilient system, give Hystrix a try in your Java applications!