Maven Publication Error: Unable to Publish AAR to Maven Using Gradle
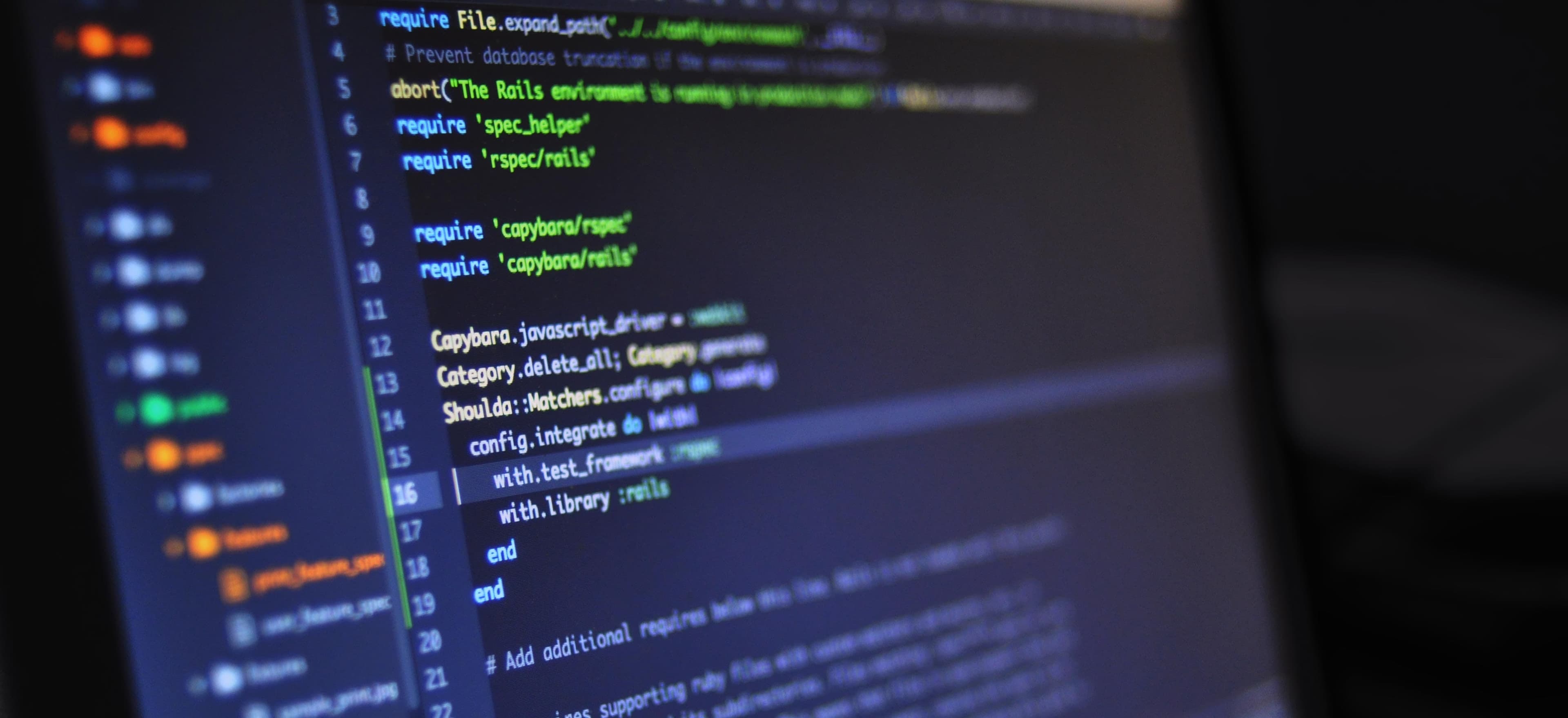
- Published on
Introduction
In the world of Java development, build automation tools like Maven and Gradle have become indispensable. They make it easier for developers to manage dependencies, build and package their projects, and publish them to remote repositories. However, sometimes you may encounter issues while publishing your AAR (Android Archive) file to Maven using Gradle. One common error that developers face is the "Unable to publish AAR to Maven" error. In this article, we will explore the possible causes of this error and provide solutions to help you resolve it.
Understanding the Error
When you try to publish your AAR file to Maven using Gradle, you may encounter an error message similar to the following:
Could not publish configuration 'archives'
Cannot publish artifacts configured with 'uploadArchives' task, please use 'maven-publish' plugin.
This error occurs when you are using the legacy uploadArchives
task in your Gradle build script instead of the modern maven-publish
plugin.
Causes of the Error
The error message suggests that you have configured your build script to use the uploadArchives
task, which is deprecated. The uploadArchives
task is part of the older maven
plugin, which has been replaced by the new maven-publish
plugin.
The maven-publish
plugin provides a more flexible and powerful way to publish artifacts to Maven repositories. It allows you to define and configure publications that describe how your artifacts should be published, including their group, name, version, and other properties.
Resolving the Error
To resolve the "Unable to publish AAR to Maven" error, you need to migrate your build script from using the deprecated uploadArchives
task to using the maven-publish
plugin. Here's how you can do it:
Step 1: Apply the maven-publish
Plugin
In your Gradle build script, locate the plugins
block and add the following line:
plugins {
// Other plugins...
id 'maven-publish'
}
This applies the maven-publish
plugin to your project, making its functionality available to you.
Step 2: Define Your Publications
Next, you need to define the publications that describe how your artifacts should be published. For an AAR file, you can create a publication like this:
publishing {
publications {
aar(MavenPublication) {
// Configure the publication attributes
groupId = 'com.example'
artifactId = 'my-library'
version = '1.0.0'
// Specify the AAR file to be published
artifact(file('path/to/your-library.aar'))
// Optionally, if you have other files to publish, you can include them as well
// artifact(file('path/to/another-file.txt'))
}
}
}
In the publications
block, we defined a publication with the name aar
, using the MavenPublication
type. Inside this publication, we can specify the attributes like groupId
, artifactId
, and version
, which are required by Maven. We also use the artifact
method to specify the AAR file to be published.
Step 3: Configure the Maven Repository
Next, you need to configure the Maven repository where you want to publish your artifacts. You can do this by adding the following block to your build script:
repositories {
maven {
url 'https://repo.example.com'
credentials {
username 'your-username'
password 'your-password'
}
}
}
In the repositories
block, we configure a Maven repository with the url
pointing to the repository's URL. You can also specify your credentials if required.
Step 4: Publish the Artifacts
Finally, you can publish your artifacts by running the publish
task. In your terminal or command prompt, navigate to your project directory and run the following command:
gradle publish
This will execute the publish
task, which will publish your artifacts to the configured Maven repository.
Conclusion
In this article, we've discussed how to resolve the "Unable to publish AAR to Maven" error when using Gradle. By migrating from the deprecated uploadArchives
task to the maven-publish
plugin, you can successfully publish your AAR file to a Maven repository. Remember to update your Gradle build script accordingly and configure the necessary publications and repositories.
Checkout our other articles