Avoiding Object Resurrection: A Prevention Guide
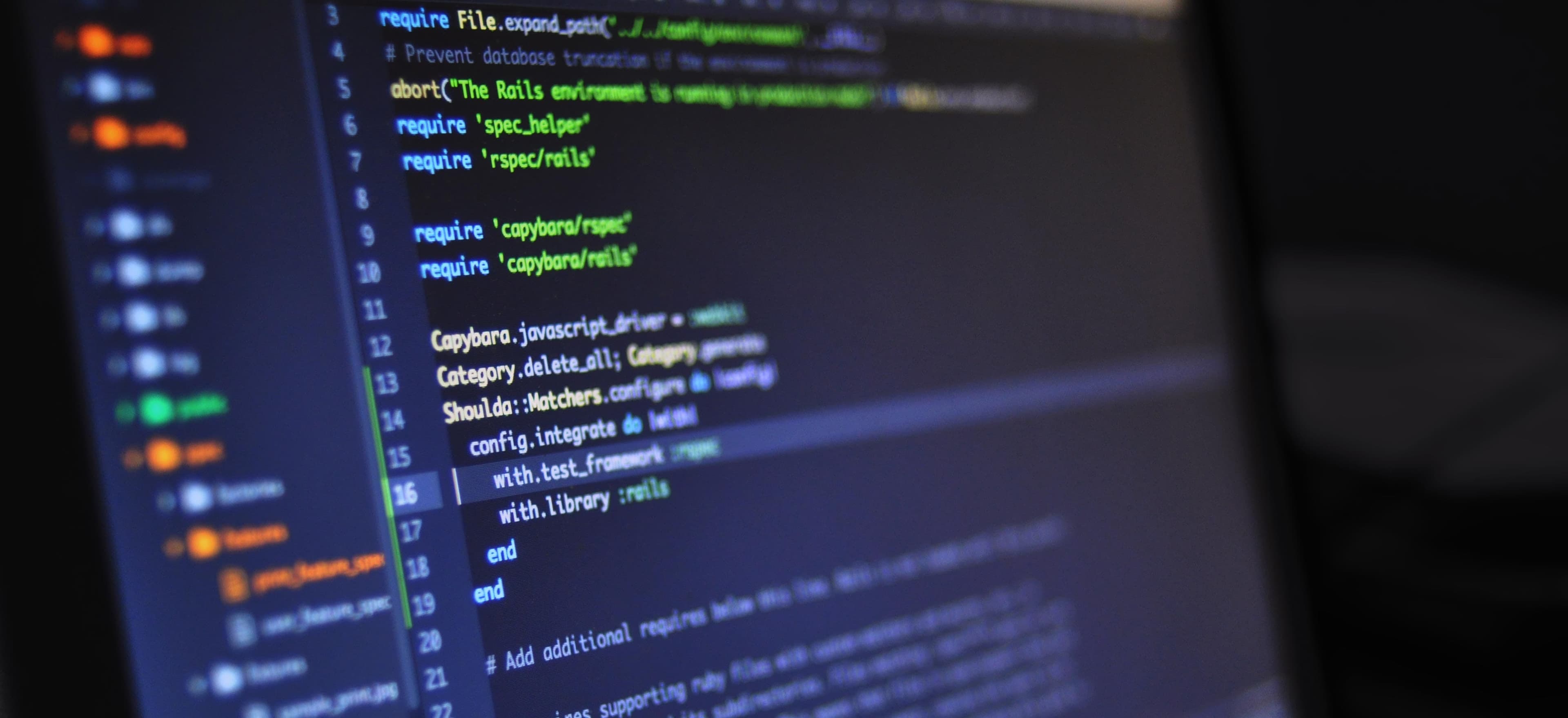
- Published on
Introduction
In Java programming, "object resurrection" refers to the practice of reviving an object that has been garbage collected. While it may seem like a harmless action, resurrecting objects can lead to serious memory leaks and performance issues. In this blog post, we will explore what object resurrection is, why it should be avoided, and provide a guide on how to prevent it in your Java applications.
What is Object Resurrection?
Object resurrection occurs when an object is resurrected from its finalizer. The finalizer is a method in the Object
class that is called by the garbage collector before an object is garbage collected. Resurrecting an object involves assigning a non-null reference to a finalized object, keeping it alive beyond its intended lifetime.
Here is an example to illustrate object resurrection:
public class MyClass {
private static MyClass instance;
public MyClass() {
instance = this;
}
@Override
protected void finalize() throws Throwable {
instance = this;
}
}
In the example above, the finalize
method resurrects the object by assigning the this
reference back to the instance
variable. This prevents the object from being garbage collected.
Why Should Object Resurrection be Avoided?
Memory Leaks
Resurrecting objects can lead to memory leaks. When an object is resurrected, it is added back to the memory heap, but its references are most likely no longer valid. This means that the resurrected object becomes unreachable and cannot be garbage collected in future garbage collection cycles. Over time, these resurrected objects can accumulate and consume a significant amount of memory, leading to out-of-memory errors.
Performance Issues
Object resurrection can also cause performance issues in your Java application. When an object is resurrected, it goes through the entire process of object initialization, including executing constructors and initializing fields. This can be a costly operation, especially if the object requires complex initialization logic. As more objects are resurrected, the performance of your application will degrade, impacting the user experience.
Unpredictable Behavior
Resurrecting objects can introduce unpredictable behavior into your application. By reviving an object that was meant to be garbage collected, you are disrupting the normal lifecycle of the object. This can lead to subtle bugs and difficult-to-debug issues. It is generally best to let objects go through their natural lifecycle, allowing the garbage collector to clean up unused objects.
How to Prevent Object Resurrection
Avoiding object resurrection is crucial for maintaining the stability and performance of your Java application. Here are some guidelines to prevent object resurrection:
1. Avoid Finalizers
The first and most important step in preventing object resurrection is to avoid using finalizers. Finalizers should be used sparingly, if at all, as they are generally considered to be an unreliable and unpredictable mechanism for resource cleanup. Instead, consider using try-with-resources or close()
methods to correctly deallocate resources.
2. Use Weak References
In cases where you need to keep track of objects without preventing them from being garbage collected, consider using weak references. Weak references allow an object to be garbage collected if there are no strong references to it. This provides a more reliable and efficient way to manage object lifecycle without the risk of object resurrection.
Here is an example of using weak references:
import java.lang.ref.WeakReference;
public class MyCache {
private Map<String, WeakReference<MyObject>> cache = new HashMap<>();
public void put(String key, MyObject value) {
cache.put(key, new WeakReference<>(value));
}
public MyObject get(String key) {
WeakReference<MyObject> reference = cache.get(key);
return reference != null ? reference.get() : null;
}
}
In the example above, the MyCache
class uses WeakReference
to store values. If an object stored in the cache is no longer referenced elsewhere, it will be garbage collected automatically.
3. Use Clean-up Actions
Instead of relying on finalizers, you can use clean-up actions to explicitly release resources when they are no longer needed. Clean-up actions can be implemented using the AutoCloseable
interface and the try-with-resources
statement.
Here is an example of using clean-up actions:
public class MyResource implements AutoCloseable {
public MyResource() {
// Resource initialization
}
public void doSomething() {
// Perform operations
}
@Override
public void close() {
// Release resources
}
}
public class MyApplication {
public static void main(String[] args) {
try (MyResource resource = new MyResource()) {
// Use the resource
resource.doSomething();
} catch (Exception e) {
// Handle exception
}
}
}
In the example above, the MyResource
class implements the AutoCloseable
interface, which allows it to be used within a try-with-resources
statement. The close
method is then called automatically at the end of the block, ensuring that resources are properly released.
4. Use Object Pooling
Object pooling is a technique where a pool of pre-initialized objects is used instead of creating and destroying objects on-demand. By reusing objects from the pool, you can avoid the need for object resurrection and improve performance by reducing object allocation and garbage collection overhead.
Here is an example of object pooling:
public class MyObjectPool {
private List<MyObject> pool = new ArrayList<>();
public MyObject acquire() {
if (pool.isEmpty()) {
return new MyObject();
} else {
return pool.remove(0);
}
}
public void release(MyObject object) {
// Reset object state if necessary
pool.add(object);
}
}
In the example above, the MyObjectPool
class maintains a pool of MyObject
instances. The acquire
method retrieves an object from the pool, either by creating a new instance or reusing an existing instance. The release
method returns an object to the pool for future use.
Conclusion
Object resurrection should be avoided in Java applications due to the potential for memory leaks, performance issues, and unpredictable behavior. By following the prevention guide outlined in this blog post, you can ensure that your Java applications are robust, efficient, and maintainable. Remember to avoid finalizers, use weak references when needed, implement clean-up actions, and consider object pooling as a performance optimization.
Checkout our other articles