Handling Backpressure in Spring Reactor
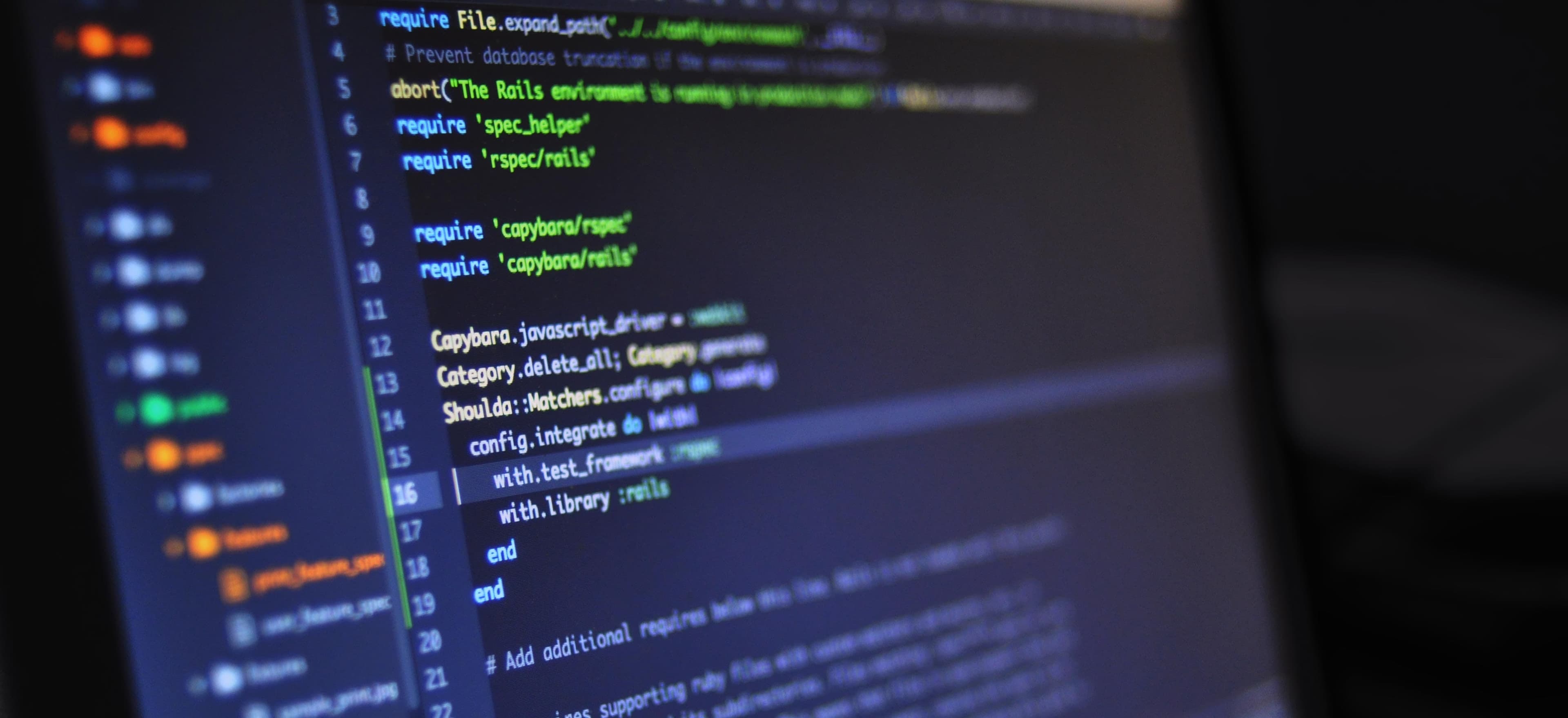
- Published on
Table of Contents
Introduction
Asynchronous programming has become a popular approach in modern application development. It allows developers to write code that can efficiently handle a large number of concurrent requests. However, when dealing with streams of data, it's important to handle backpressure. Backpressure is the mechanism by which the consumer of a stream can signal the producer to slow down or stop producing data when it's unable to keep up with the rate of consumption.
Understanding Backpressure
Before we dive into handling backpressure in Spring Reactor, let's briefly understand what backpressure is and why it's important.
Backpressure is a way for the consumer to tell the producer to slow down or stop producing data when it's unable to keep up with the rate of consumption. In other words, backpressure is a mechanism that allows the consumer to control the flow of data from the producer.
Without backpressure, if the producer is producing data at a faster rate than the consumer can process it, the consumer may end up being overwhelmed with a large amount of data. This can lead to out-of-memory errors, increased latency, and poor overall system performance.
Backpressure in Spring Reactor
Spring Reactor provides several mechanisms to handle backpressure effectively. Let's explore some of the main strategies and techniques:
Using Flux and Mono
In Spring Reactor, Flux and Mono are the two main types for handling reactive streams. Both Flux and Mono are designed to handle backpressure by default.
Flux represents a stream of 0 to N elements, while Mono represents a stream of 0 or 1 element. When a consumer subscribes to a Flux or Mono, it can request an arbitrary number of elements or signal that it wants to cancel the subscription altogether.
Here's an example of a basic Flux declaration:
Flux<Integer> numbersFlux = Flux.range(1, 1000);
In this example, we create a Flux that emits numbers from 1 to 1000. The consumer can then subscribe to this Flux and handle the elements as they arrive. The consumer can control the rate of consumption by requesting a specific number of elements at a time.
Buffering
Buffering is a common technique to handle backpressure in reactive streams. It allows the consumer to specify how many elements it wants to consume at a time.
The buffer()
operator in Spring Reactor can be used to buffer elements emitted by a Flux or Mono. Here's an example:
Flux<Integer> numbersFlux = Flux.range(1, 1000).buffer(100);
In this example, we buffer the elements emitted by the Flux in chunks of 100. This means that the consumer will receive batches of 100 elements at a time, which can help in handling backpressure effectively.
Dropping or Filtering
Sometimes, the consumer may not be able to handle all the elements produced by the producer. In such cases, it's important to have a strategy to handle overflow gracefully.
Spring Reactor provides operators like onBackpressureDrop()
and filter()
that can be used to drop or filter elements when backpressure occurs.
The onBackpressureDrop()
operator drops elements when the consumer is unable to keep up with the rate of production.
Here's an example: [^1^]
Flux<Integer> numbersFlux = Flux.range(1, 1000).onBackpressureDrop();
In this example, if the consumer is unable to keep up with the rate of production, any excess elements emitted by the Flux will be dropped.
The filter()
operator can be used to filter elements based on certain conditions.
Here's an example: [^2^]
Flux<Integer> numbersFlux = Flux.range(1, 1000).filter(num -> num % 2 == 0);
In this example, only the even numbers emitted by the Flux will be passed to the consumer. This can help in reducing the rate of production and handling backpressure effectively.
Backpressure Strategies
Spring Reactor provides different backpressure strategies that can be used to control how the producer reacts when the consumer can't keep up with the rate of consumption.
The default backpressure strategy in Spring Reactor is BUFFER
, which buffers elements emitted by the producer until the consumer is ready to consume them. However, there are other strategies available, such as DROP
, LATEST
, and ERROR
.
BUFFER
: Buffers elements until the consumer can consume them.DROP
: Drops elements when the consumer can't keep up with the rate of production.LATEST
: Keeps only the latest element emitted by the producer and drops the rest.ERROR
: Throws aBackpressureException
when the consumer can't keep up with the rate of production.
Here's an example of using a different backpressure strategy:
Flux<Integer> numbersFlux = Flux.range(1, 1000).onBackpressureBuffer(100, BufferOverflowStrategy.DROP_OLDEST);
In this example, we use the onBackpressureBuffer()
operator with DROP_OLDEST
strategy to drop the oldest elements when backpressure occurs.
Conclusion
Handling backpressure is crucial when dealing with streams of data in reactive applications. In this blog post, we explored how to handle backpressure in Spring Reactor, a popular reactive programming framework for Java.
We discussed different strategies and techniques, such as using Flux and Mono, buffering, dropping or filtering elements, and choosing appropriate backpressure strategies. These techniques can help you ensure that your stream processing pipelines can handle backpressure effectively and prevent issues like out-of-memory errors or poor system performance.
By understanding and implementing these strategies, you can build robust and scalable reactive applications with Spring Reactor. So go ahead and give it a try in your next project!
External Links: