Inefficient Handling of Asynchronous Flow Invocations in Corda Services
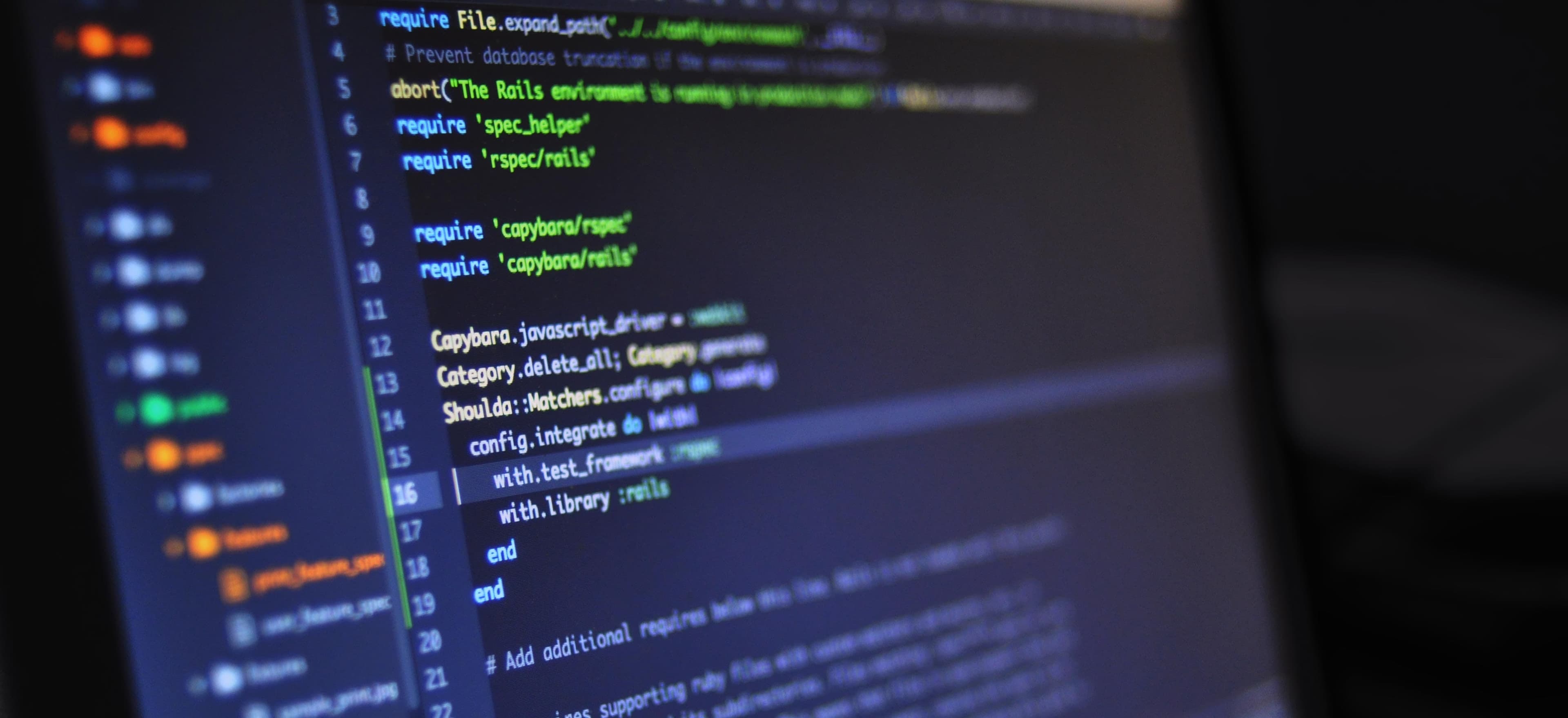
- Published on
Introduction
In Corda, a blockchain platform, services are used to handle asynchronous flow invocations. These services play a crucial role in ensuring the reliable execution of flows and managing the communication between nodes. However, inefficient handling of asynchronous flow invocations can lead to performance issues and hinder the scalability of the system.
In this article, we will explore the common pitfalls in handling asynchronous flow invocations in Corda services and discuss strategies to improve their efficiency and performance.
Table of Contents
- Understanding Asynchronous Flow Invocations
- Common Pitfalls in Handling Asynchronous Flow Invocations
- Strategies to Improve Efficiency in Handling Asynchronous Flow Invocations
- Conclusion
Understanding Asynchronous Flow Invocations
In Corda, flows are the building blocks of business processes. They represent a sequence of steps that are executed asynchronously. Flows can interact with each other and with external systems through services.
Services in Corda act as intermediaries between flows and external systems. They help in encapsulating business logic and managing the communication between different components of the system. Services can be invoked from within a flow using the serviceHub
object.
In an ideal scenario, a service should be lightweight and respond quickly to flow invocations. However, in reality, inefficient handling of asynchronous flow invocations can lead to delays and impact the overall performance of the system.
Common Pitfalls in Handling Asynchronous Flow Invocations
Let's take a look at some common pitfalls in handling asynchronous flow invocations in Corda services:
1. Blocking Calls
One of the main reasons for inefficient handling of flow invocations is blocking calls within services. Blocking calls can result in the suspension of the flow and lead to delays in the execution of subsequent steps.
To avoid blocking calls, it is advisable to use non-blocking I/O operations and asynchronous programming techniques. For example, you can use Kotlin coroutines to handle asynchronous operations in a non-blocking way.
Here's an example of how you can use coroutines to handle asynchronous operations:
// Perform a non-blocking I/O operation
val result = withContext(Dispatchers.IO) {
// Perform an I/O operation
}
// Continue with the flow execution
2. Lack of Parallelism
Another issue that can impact the efficiency of flow invocations is the lack of parallelism. If a service performs time-consuming operations sequentially, it can result in delays and decreased performance.
To improve parallelism, you can divide the workload into smaller tasks and execute them concurrently. This can be achieved using parallel streams or Kotlin coroutines.
Here's an example of how you can use parallel streams to execute tasks concurrently:
val tasks = listOf<Task>()
tasks.parallelStream().forEach { task ->
// Perform the task
}
3. Inefficient Resource Management
Inefficient resource management can also lead to performance issues in Corda services. For example, failing to close database connections or releasing other resources properly can result in resource leaks and decreased performance.
To ensure efficient resource management, it is important to properly handle exceptions and release resources in a timely manner. You can use Kotlin's use
function to automatically close resources once they are no longer needed.
Here's an example of how you can use the use
function to handle database connections:
databaseConnection.use { connection ->
// Use the database connection
}
4. Lack of Caching
Another pitfall in handling flow invocations is the lack of caching. If a service repeatedly performs the same operation without caching the results, it can result in unnecessary computations and decreased performance.
To improve performance, you can cache the results of time-consuming operations. You can use libraries like Guava or Caffeine to implement caching in your Corda services.
Here's an example of how you can implement caching using Guava:
val cache = CacheBuilder.newBuilder()
.maximumSize(100)
.expireAfterAccess(10, TimeUnit.MINUTES)
.build<String, Any>()
val result = cache.get("key") {
// Perform a time-consuming operation
}
Strategies to Improve Efficiency in Handling Asynchronous Flow Invocations
Now that we understand the common pitfalls, let's discuss strategies to improve the efficiency of handling asynchronous flow invocations in Corda services.
1. Use Non-Blocking I/O
To avoid blocking calls, it is recommended to use non-blocking I/O operations. Non-blocking I/O allows multiple operations to be performed concurrently, resulting in improved performance and scalability.
You can use Kotlin coroutines to handle asynchronous operations in a non-blocking way. Coroutines provide a lightweight way to perform asynchronous operations without blocking the execution of the flow.
2. Parallelize Time-Consuming Operations
To improve parallelism and decrease the execution time of time-consuming operations, you can parallelize them. Divide the workload into smaller tasks and execute them concurrently using parallel streams or Kotlin coroutines.
Parallelizing operations can significantly improve the performance of Corda services, especially when dealing with large datasets or complex computations.
3. Optimize Resource Management
Efficient resource management is crucial for the performance of Corda services. Ensure that resources like database connections are properly closed and released when they are no longer needed.
You can use Kotlin's use
function to handle resources and ensure their proper release. Additionally, consider using connection pooling to optimize the usage of database connections and minimize the overhead of creating new connections.
4. Implement Caching
Caching can greatly improve the performance of Corda services by reducing the need for redundant computations. Cache the results of time-consuming operations to avoid recomputing them unnecessarily.
You can use libraries like Guava or Caffeine to implement caching in your Corda services. Configure the cache to suit your specific requirements, considering factors like cache size and expiry time.
Conclusion
Efficient handling of asynchronous flow invocations is crucial for the performance and scalability of Corda services. By avoiding blocking calls, improving parallelism, optimizing resource management, and implementing caching, you can enhance the efficiency of your Corda services and ensure reliable execution of flows.
Take the time to analyze your services and identify potential bottlenecks. Implement the strategies discussed in this article to improve the efficiency and performance of your Corda services, ultimately leading to a better user experience and increased customer satisfaction.