NullPointerException: The Most Common Java Exception and How to Handle It
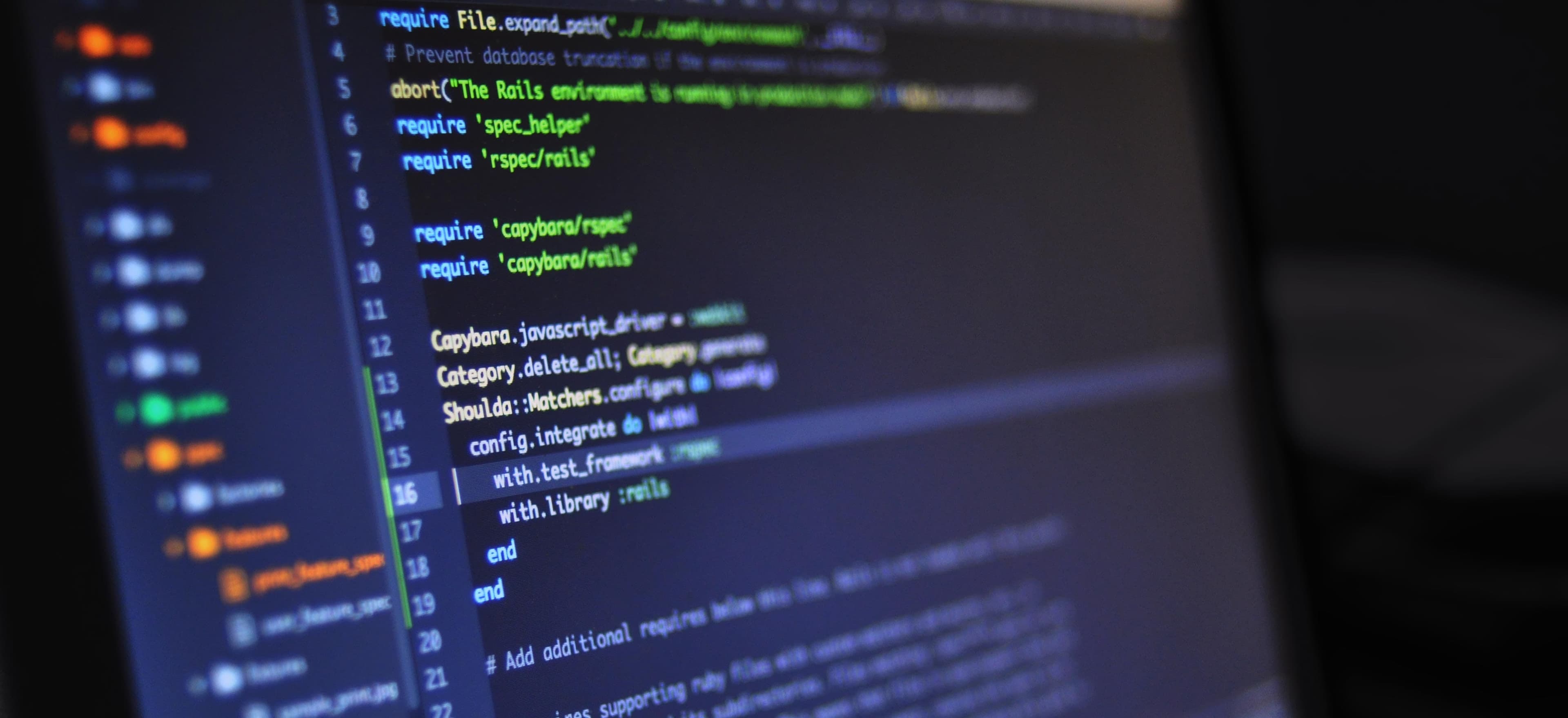
- Published on
Introduction
NullPointerException is one of the most common exceptions in the Java programming language. It occurs when a program attempts to use an object reference that has not been initialized, resulting in a null value. This exception can be frustrating for developers, but understanding why it occurs and how to handle it can help prevent bugs and improve the overall quality of your code.
In this article, we will explore the causes, consequences, and strategies to handle NullPointerException in Java.
Table of Contents
- What is a NullPointerException?
- Common Causes of NullPointerException
- Handling NullPointerException
- Best Practices to Avoid NullPointerException
- Conclusion
What is a NullPointerException?
A NullPointerException, also known as NPE, is a runtime exception that is thrown when a piece of code tries to access or manipulate an object reference that is currently assigned the value of 'null'. In other words, it occurs when you try to call a method or access a field on an object that hasn't been created or properly initialized.
For example, consider the following code snippet:
String message = null;
System.out.println(message.length());
In this case, we are trying to access the length()
method of the message
String, but since message
is null
, a NullPointerException will be thrown at runtime.
Common Causes of NullPointerException
NullPointerExceptions are often caused by simple programming mistakes, such as:
Forgetting to initialize an object reference
String message;
System.out.println(message.length());
In this example, the message
String is declared but not initialized. Therefore, when we try to call the length()
method on it, a NullPointerException will be thrown.
Accessing an object reference that has been set to null
String[] names = new String[3];
names[0] = "John";
names[1] = "Jane";
names[2] = "Mark";
String message = names[3];
System.out.println(message.length());
In this case, we are trying to access the element at index 3 of the names
array, which does not exist. This will result in a NullPointerException when we try to call the length()
method on the message
String.
Passing null as an argument to a method
public void printLength(String str) {
System.out.println(str.length());
}
String message = null;
printLength(message);
In this example, a printLength()
method is defined to print the length of a given string. When we pass a null reference to this method, a NullPointerException will be thrown within the method when it tries to access the length of the string.
Returning null from a method
public String getMessage() {
return null;
}
String message = getMessage();
System.out.println(message.length());
In this case, the getMessage()
method returns null instead of a valid string. Hence, when we try to call the length()
method on the result, a NullPointerException will occur.
External Links
- Check out this article on Null Pointer Exception in Java from Baeldung.
Handling NullPointerException
While it is impossible to completely eliminate the possibility of encountering a NullPointerException, there are several strategies you can employ to handle it and minimize the impact it has on your code.
Checking for null before accessing an object reference
The most straightforward way to prevent a NullPointerException is to check if an object reference is null before using it. This can be done using an if statement or the ternary operator.
String message = null;
if (message != null) {
System.out.println(message.length());
}
Alternatively, you can use the ternary operator to handle the null case explicitly:
String message = null;
int length = (message != null) ? message.length() : 0;
System.out.println(length);
By performing this null check, you can avoid calling methods on null references and handle the situation proactively.
Using the NullPointerException catch block
Another way to handle a NullPointerException is by using a try-catch block and catching the exception. This approach is useful when you want to perform specific actions or provide alternative behavior when a null reference is encountered.
String message = null;
try {
System.out.println(message.length());
} catch (NullPointerException e) {
System.out.println("Error: Null reference encountered");
}
By catching the NullPointerException, we can display a custom error message or perform any necessary error handling.
Preemptively initializing object references
To avoid NullPointerExceptions altogether, you can initialize object references when they are declared or ensure they are properly initialized before being used.
String message = ""; // initialize with an empty string
System.out.println(message.length());
By initializing message
with an empty string, we prevent a NullPointerException from occurring when we call the length()
method.
Using the Optional class
Java 8 introduced the Optional
class, which provides a convenient way to handle null values. Instead of returning a null reference, you can use the Optional
class to wrap the result and indicate the absence of a value.
public Optional<String> getMessage() {
// Some logic to determine the message
return Optional.ofNullable(message);
}
Optional<String> messageOptional = getMessage();
if (messageOptional.isPresent()) {
System.out.println(messageOptional.get().length());
} else {
System.out.println("Error: Null reference encountered");
}
By using Optional
, you can explicitly handle the case when a value is not present, reducing the likelihood of encountering NullPointerExceptions.
Best Practices to Avoid NullPointerException
In addition to the strategies mentioned above, following these best practices can help you avoid frequent encounters with NullPointerException:
Always initialize object references
When declaring object references, make it a habit to initialize them immediately. If you are unsure of the value, initiate them with default values or empty states to avoid null references.
Check method contract documentation
Always read and understand the documentation for methods you are using or overriding. Make sure to know if a method can return null values or accept null arguments. Handling these cases appropriately can prevent unexpected NullPointerExceptions.
External Links
- Here is the official Java documentation on NullPointerException from Oracle.
Avoid returning null from methods
Avoid returning null from your methods whenever possible. Instead, consider using the Optional
type or return meaningful default values to indicate the absence of a value.
Use null-safe operators and libraries
Consider using third-party libraries like Guava or Apache Commons which provide utility methods for working with null values. These libraries offer null-safe operators, methods, and classes that can simplify null handling in your code.
External Links
- Learn more about Guava's Null Explained from the official Guava GitHub repository.
- Apache Commons also provides Null-safe Utilities to handle null values effectively.
Conclusion
NullPointerException is a common and often frustrating exception in Java. Understanding its causes and implementing effective strategies to handle and prevent it will improve the robustness and reliability of your code. By following best practices, checking for null references, and using appropriate handling techniques, you can minimize the occurrences of NullPointerException and write more stable and maintainable Java applications.
Checkout our other articles