How to Handle Circuit Breaker Patterns with Spring Cloud HystrixCommands
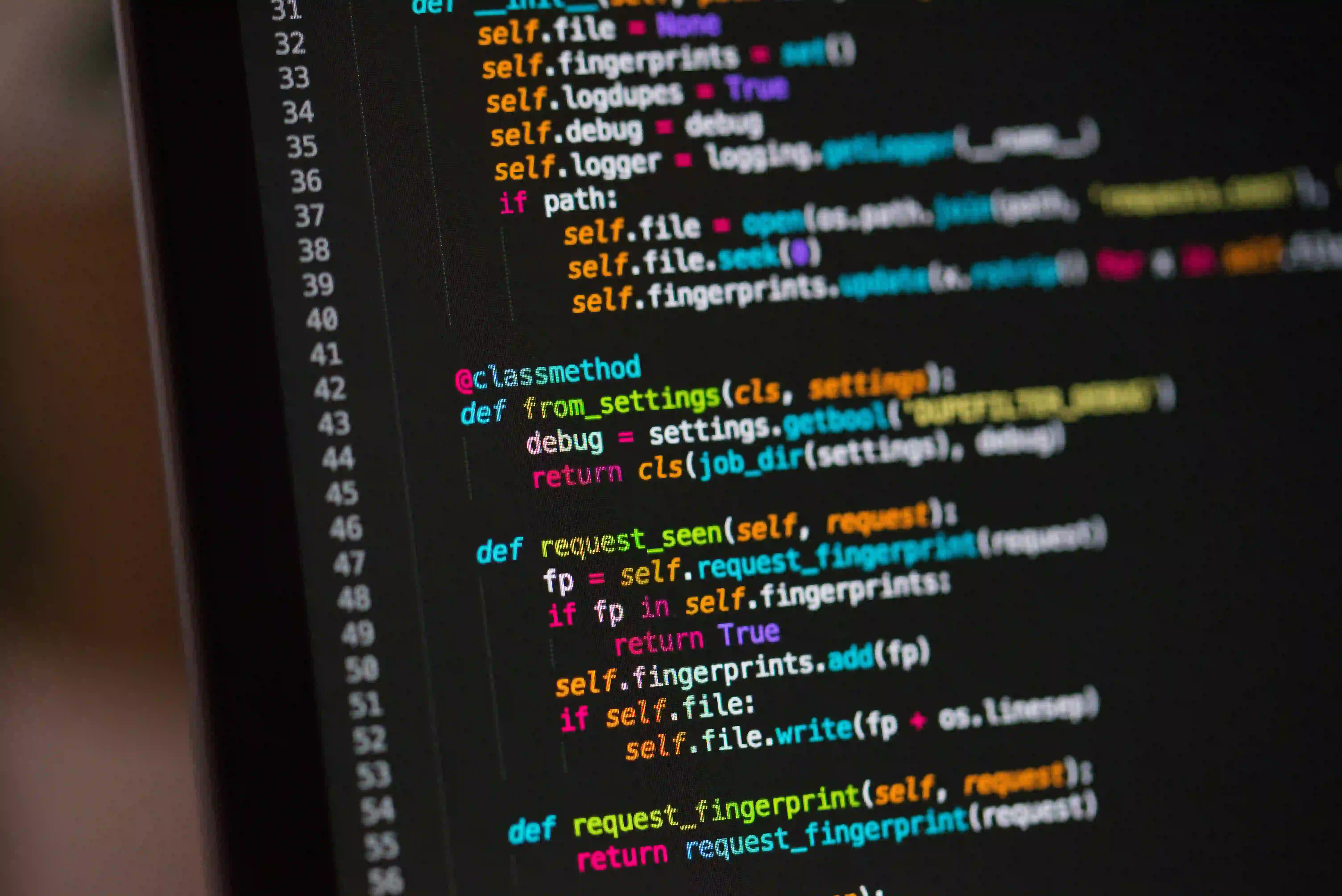
Introduction
In modern distributed systems, service dependencies are common. However, when a dependency becomes slow or unresponsive, it can have a cascading effect on other services in the system. The Circuit Breaker pattern is a great way to handle such scenarios and prevent further damage.
Spring Cloud provides a powerful library called Hystrix, which allows you to implement the Circuit Breaker pattern in your Java applications. In this blog post, we will explore how to use Spring Cloud HystrixCommands to handle Circuit Breaker patterns effectively.
What is the Circuit Breaker Pattern?
The Circuit Breaker pattern is a design pattern used in distributed systems to handle failure scenarios when interacting with remote services. It's based on the same principle as an electrical circuit breaker. When a fault is detected, the circuit breaker interrupts the flow of electricity to prevent further damage.
Similarly, in a distributed system, the Circuit Breaker pattern monitors the interactions with remote services. If the remote service becomes slow or unresponsive, the Circuit Breaker trips and further requests are not sent to the remote service. Instead, fallback mechanisms are used to provide alternative responses or handle the failure gracefully.
Implementing Circuit Breaker with Spring Cloud HystrixCommands
Spring Cloud provides an integration with Hystrix, which is a mature and battle-tested library for implementing the Circuit Breaker pattern in your Java applications. Hystrix allows you to define commands, which are encapsulated business operations that can be protected by a circuit breaker.
To get started with Spring Cloud HystrixCommands, you need to include the necessary dependencies in your project's pom.xml
file:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
Once you have the dependencies in place, you can start using HystrixCommands in your code. Here's a simple example:
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String getRemoteResponse() {
// Call the remote service here
// ...
}
public String fallbackMethod() {
// Fallback logic goes here
// ...
}
In this example, getRemoteResponse
is a business operation that you want to protect with a circuit breaker. The @HystrixCommand
annotation indicates that the method should be wrapped in a Hystrix command, and the fallbackMethod
parameter specifies the method to be called in case of failure.
When a request is made to getRemoteResponse
, Hystrix will execute the business operation. If the operation fails or takes too long, Hystrix will trip the circuit breaker and call the fallback method.
Configuring Hystrix Command Properties
By default, Hystrix uses sensible defaults for configuring command properties such as timeout, circuit breaker threshold, and error thresholds. However, you can customize these properties based on your specific needs.
To configure Hystrix command properties, you can use the @HystrixProperty
annotation. For example, to set the timeout value to 5 seconds, you can do the following:
@HystrixCommand(fallbackMethod = "fallbackMethod", commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "5000")
})
public String getRemoteResponse() {
// Call the remote service here
// ...
}
In addition to execution.isolation.thread.timeoutInMilliseconds
, there are many other properties that you can configure using @HystrixProperty
. You can refer to the Hystrix documentation for a complete list of available properties.
Bulkheading with Hystrix Thread Pool Configuration
In a distributed system, it's important to isolate different services and prevent cascading failures. Bulkheading is a technique that allows you to achieve this isolation by using separate thread pools for different functionalities or services.
Hystrix provides the ability to configure thread pools for each command group. By default, Hystrix uses a single thread pool for all command groups. However, you can configure separate thread pools for different command groups using the @HystrixCommand
annotation.
Here's an example:
@HystrixCommand(fallbackMethod = "fallbackMethod", threadPoolKey = "remoteServiceThreadPool")
public String getRemoteResponse() {
// Call the remote service here
// ...
}
In this example, the remoteServiceThreadPool
thread pool will be used for executing the getRemoteResponse
command. You can configure the properties of this thread pool in the Spring configuration file.
Monitoring and Metrics with Hystrix Dashboard
Hystrix provides a built-in dashboard that allows you to monitor the health and performance of your Hystrix commands. The Hystrix dashboard provides real-time metrics such as request volume, error percentage, thread pool usage, and circuit breaker status.
To enable the Hystrix dashboard, you need to include the following dependency in your pom.xml
file:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-hystrix-dashboard</artifactId>
</dependency>
Once the dependency is included, you can access the Hystrix dashboard at http://localhost:8080/hystrix
(assuming your application is running on port 8080).
Conclusion
Handling Circuit Breaker patterns is essential for building robust and resilient distributed systems. Spring Cloud HystrixCommands provides a powerful and easy-to-use mechanism for implementing the Circuit Breaker pattern in your Java applications.
In this blog post, we explored how to use Spring Cloud HystrixCommands to handle Circuit Breaker patterns effectively. We discussed the basics of the Circuit Breaker pattern, how to implement it with Spring Cloud HystrixCommands, and how to configure command properties and thread pools. We also touched on monitoring and metrics using the Hystrix dashboard.
By leveraging the power of Spring Cloud HystrixCommands, you can build more reliable and fault-tolerant applications that can gracefully handle failures and prevent them from propagating across your system.