Understanding Entity State Transitions in JPA/Hibernate
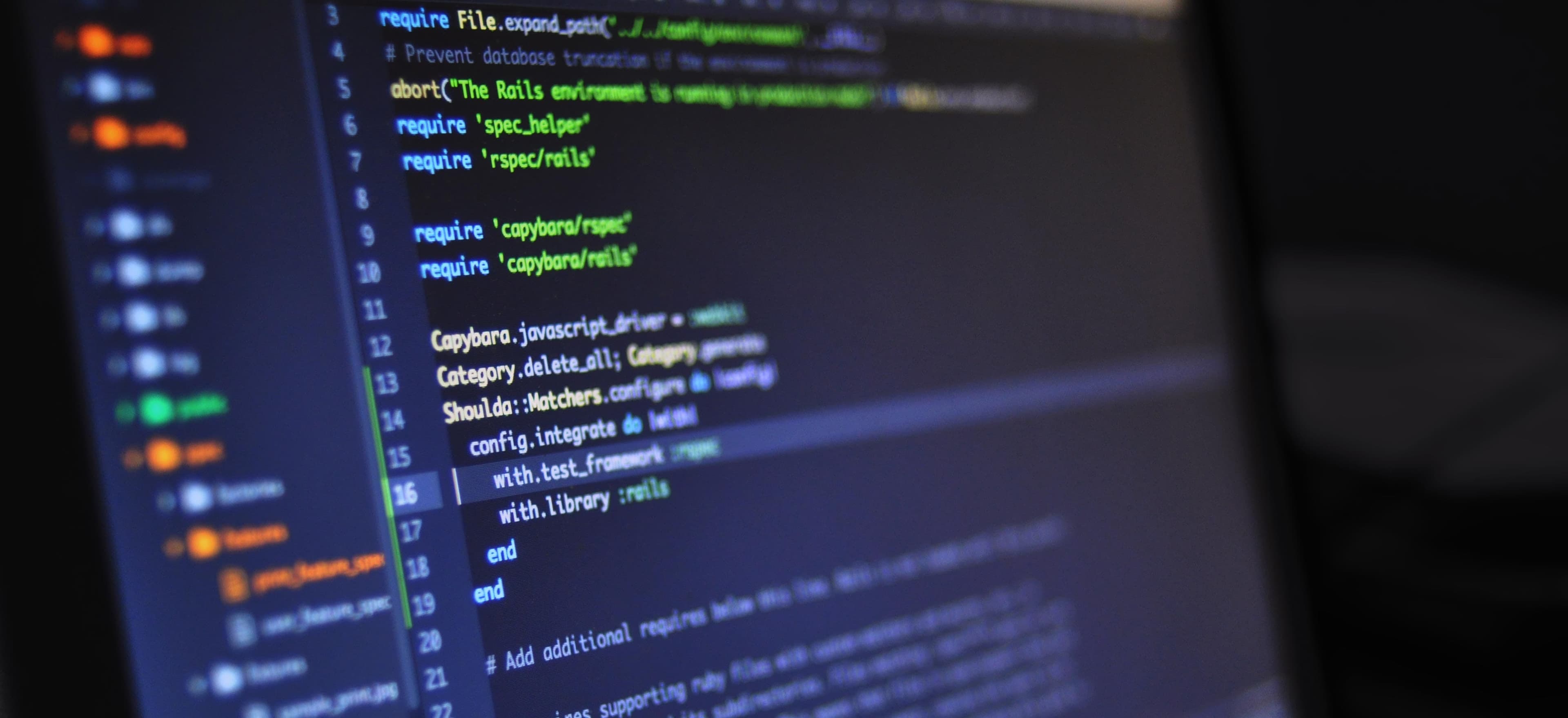
- Published on
Introduction
In Java Persistence API (JPA) and Hibernate, entity state transitions play a crucial role in the persistence and management of objects. Understanding how these transitions occur is essential to ensure the correct behavior of your application.
In this article, we will explore the different entity states in JPA/Hibernate and the various ways to transition between them. We will also discuss the implications of these state transitions and how to handle them effectively in your application.
Entity States in JPA/Hibernate
In JPA/Hibernate, entities can exist in several different states, depending on their lifecycle. The three main states that an entity can be in are:
-
Transient State: An entity is said to be in a transient state when it is not associated with any persistence context. In this state, changes made to the entity are not persisted to the database.
-
Persistent State: An entity is in a persistent state when it is associated with a persistence context. Any changes made to the entity in this state will be automatically synchronized with the database when the persistence context is flushed.
-
Detached State: An entity is in a detached state when it was previously associated with a persistence context but is not currently associated with any. In this state, changes made to the entity must be explicitly managed by reattaching it to a persistence context or merging the changes back to a new persistence context.
Transitions Between Entity States
Entities can transition between different states through various operations and methods provided by JPA/Hibernate. Let's discuss the common ways in which these transitions occur:
Creating New Entities
When you create a new entity instance using the new
keyword or any other means, the entity is in a transient state. It is not associated with any persistence context, and no actions are taken to persist the entity until you explicitly call the persist
method.
// Creating a new transient entity
Person person = new Person();
person.setName("John Doe");
// Persisting the entity to transition to the persistent state
entityManager.persist(person);
Retrieving Entities
When you retrieve an entity from the database using the find
or getReference
method, the entity transitions to the persistent state. The retrieved entity is associated with the current persistence context and any subsequent changes made to the entity will be persisted.
// Retrieving an entity from the database
Person person = entityManager.find(Person.class, 1L);
person.setName("Updated Name"); // The changes will be persisted
Modifying Entities
Entities in the persistent state can be modified by simply changing their properties. These modifications are automatically tracked by the persistence context, and the changes are synchronized with the database when the persistence context is flushed.
// Modifying a persistent entity
Person person = entityManager.find(Person.class, 1L);
person.setName("Updated Name"); // The changes will be persisted on flush
Removing Entities
Entities can be removed from the persistence context and the database using the remove
method. After removal, the entity transitions to a removed state and will no longer be associated with any persistence context.
// Removing a persistent entity
Person person = entityManager.find(Person.class, 1L);
entityManager.remove(person); // The entity is now in a removed state
Detaching Entities
Entities can be detached from a persistence context using the detach
or clear
methods. Once detached, the entity can be modified without the changes being automatically synchronized with the database.
// Detaching a persistent entity
Person person = entityManager.find(Person.class, 1L);
entityManager.detach(person); // The entity is now in a detached state
Merging Detached Entities
When you have a detached entity and want to apply the changes made to it back to the database, you can use the merge
method. This method creates a new managed entity from the detached one and updates the corresponding entity in the database.
// Merging a detached entity
Person detachedPerson = getDetachedPerson();
Person managedPerson = entityManager.merge(detachedPerson); // The changes are synchronized with the database
Handling Entity State Transitions
Handling entity state transitions effectively is crucial to ensure the consistency and integrity of your data. Here are some best practices to consider:
-
Use Appropriate Methods: Make sure to use the appropriate methods provided by JPA/Hibernate to transition between entity states. For example, use
persist
to transition from transient to persistent state andremove
to transition from persistent to removed state. -
Be Mindful of Entity Associations: When modifying or removing entities, be aware of their associations to prevent data inconsistencies. Ensure that you consider cascading options and handle associations accordingly.
-
Understand Persistence Contexts: Understand how persistence contexts work and manage them appropriately. Be mindful of the scope and lifespan of persistence contexts to avoid unexpected behavior.
-
Optimize Entity Fetching: Minimize the number of entities fetched from the database to reduce the overhead on the persistence context and improve performance.
-
Handle Detached Entities: When dealing with detached entities, consider the impact of merging changes back to the database. Be cautious about potential conflicts and handle them appropriately.
-
Use Transactions: Wrap state transitions within transactions to ensure atomicity and consistency. This helps in managing concurrency and maintaining database integrity.
Conclusion
Understanding entity state transitions is crucial when working with JPA/Hibernate. By grasping the different states an entity can be in and how they transition, you can effectively manage the persistence and synchronization of your data.
In this article, we discussed the transient, persistent, and detached states in JPA/Hibernate. We explored various ways to transition between these states and provided best practices for handling entity state transitions in your application. By following these guidelines, you can ensure the reliability and correctness of your data persistence operations.