Maximizing JVM Memory Usage: Essential JVM Arguments for Avoiding OutOfMemoryError
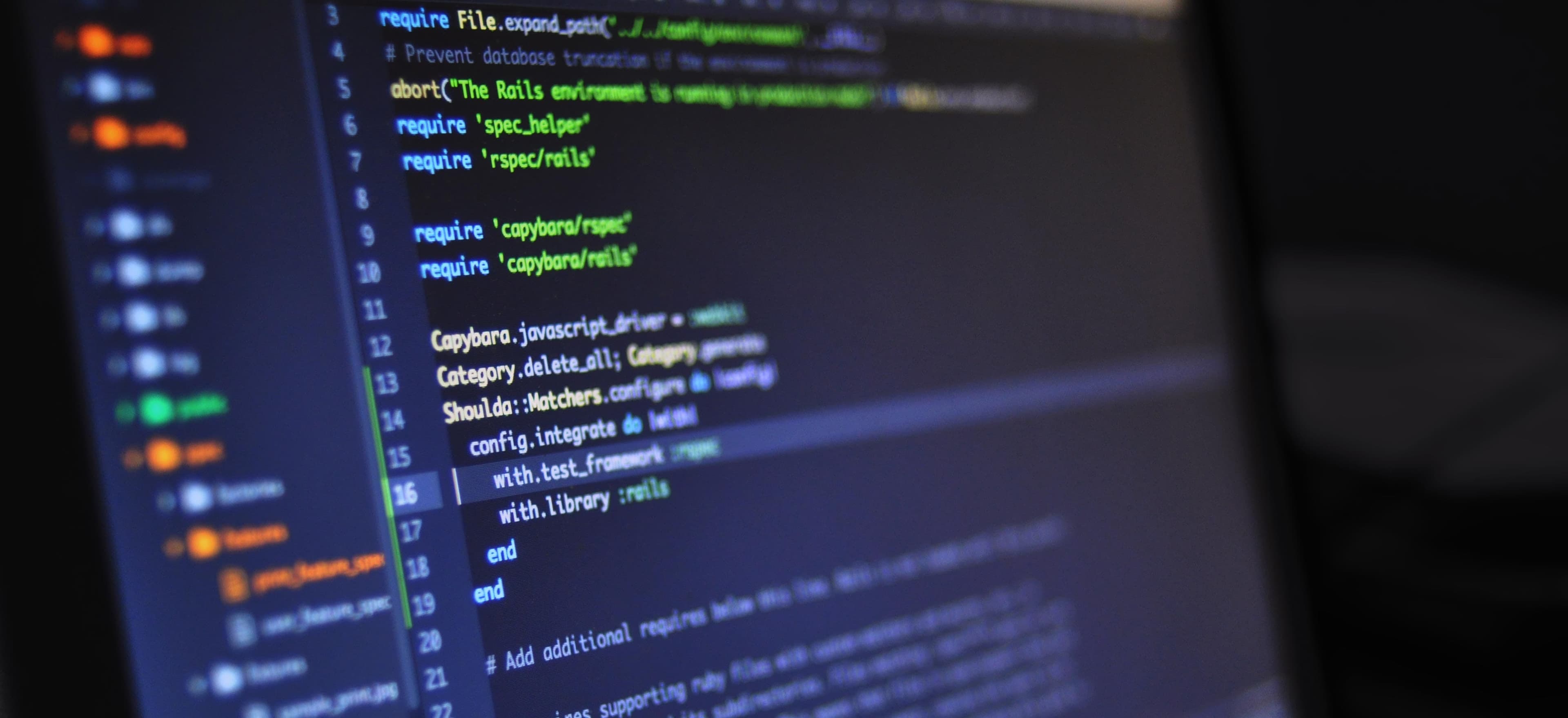
- Published on
Introduction
One common challenge in developing Java applications is managing JVM memory. It's important to understand and utilize the right JVM arguments to optimize memory usage and prevent OutOfMemoryError. In this article, we will explore some essential JVM arguments that can help maximize JVM memory usage and avoid memory-related issues.
Table of Contents
- Understanding JVM Memory
- Heap Memory
- Garbage Collection
- JVM Arguments for Memory Management
- Conclusion
Understanding JVM Memory
Before diving into JVM arguments, let's briefly discuss JVM memory and its components.
JVM memory consists of the heap and non-heap memory. The heap memory is where objects are allocated and deallocated during runtime. It is further divided into three regions: young generation, old generation, and metaspace.
The young generation holds newly created objects, while the old generation contains long-lived objects. The metaspace stores class metadata and is a replacement for the classic permanent generation (PermGen).
On the other hand, non-heap memory includes the stack, which holds method-specific data, including local variables and method pointers, and the direct memory, used by NIO operations.
Managing JVM memory efficiently is crucial for application performance and stability, as improper memory configuration can lead to OutOfMemoryError or excessive garbage collection pauses.
Heap Memory
Heap memory is the most critical part of JVM memory and requires careful management for optimal performance. JVM arguments can be used to control the size of the heap memory.
The heap memory size can be set using the -Xmx
and -Xms
JVM arguments. -Xmx
defines the maximum heap size, while -Xms
sets the initial heap size during JVM startup.
For example, to set the maximum heap size to 2GB and the initial heap size to 512MB, we can use the following arguments:
java -Xmx2G -Xms512M MyApp
By appropriately configuring the -Xmx
and -Xms
arguments, you can avoid OutOfMemoryError caused by insufficient heap memory and reduce unnecessary memory allocation.
Garbage Collection
Garbage Collection (GC) is an essential process in JVM memory management. It automatically frees up the memory occupied by objects that are no longer reachable.
There are different garbage collection algorithms available, such as Parallel GC, CMS (Concurrent Mark Sweep) GC, and G1 (Garbage-First) GC. Each algorithm has its characteristics and is suitable for different scenarios.
Properly selecting the right garbage collection algorithm can significantly impact memory usage and application performance.
JVM Arguments for Memory Management
Let's explore some essential JVM arguments that can help manage JVM memory efficiently.
XX:MaxMetaspaceSize
The XX:MaxMetaspaceSize
argument specifies the maximum size of the metaspace. The metaspace stores class metadata, including bytecode, constant pools, and symbol tables.
By default, a JVM instance automatically adjusts the metaspace size based on application requirements. However, in some cases, it's useful to explicitly set an upper limit.
For example, to limit the metaspace size to 256MB, we can use the following argument:
java -XX:MaxMetaspaceSize=256M MyApp
Setting an appropriate size for the metaspace avoids excessive memory consumption and potential out-of-memory errors caused by uncontrolled class metadata growth.
XX:NewSize and XX:MaxNewSize
The XX:NewSize
and XX:MaxNewSize
arguments control the size of the young generation, which is the area where new objects are allocated.
By default, JVM sets XX:NewSize
to a fraction of the young generation size. However, we can explicitly set the values to optimize memory.
For example, to set the young generation size to 256MB, we can use the following arguments:
java -XX:NewSize=256M -XX:MaxNewSize=256M MyApp
Balancing the young generation size with the overall heap size is crucial to minimize garbage collection pauses and achieve better performance.
XX:SurvivorRatio
The XX:SurvivorRatio
argument configures the ratio between Eden space and survivor space in the young generation.
By default, the survivor space is divided into two equally-sized areas (S0 and S1). Adjusting the survivor ratio may improve memory usage for applications with specific object allocation patterns.
For example, to set the survivor ratio to 4 (4:1 ratio of Eden space to survivor space), we can use the following argument:
java -XX:SurvivorRatio=4 MyApp
Experimenting with different survivor ratios can have a significant impact on garbage collection behavior and memory utilization.
XX:G1HeapRegionSize
The XX:G1HeapRegionSize
argument is specific to the G1 garbage collector. It defines the size of individual regions that make up the heap.
Smaller region sizes may reduce memory waste but increase memory fragmentation. Conversely, larger region sizes may increase memory waste but reduce fragmentation.
For example, to set the G1 heap region size to 1MB, we can use the following argument:
java -XX:G1HeapRegionSize=1M MyApp
Choosing an appropriate G1 heap region size depends on the specific workload and memory requirements of the application.
XX:+UseCompressedOops
The XX:+UseCompressedOops
argument enables compressed object pointers in 64-bit JVMs.
By default, JVM uses 64-bit pointers to address objects, which can lead to increased memory usage. Compressed Oops allows the JVM to use 32-bit pointers for objects within the heap, resulting in memory savings.
To enable compressed object pointers, we can use the following argument:
java -XX:+UseCompressedOops MyApp
Enabling compressed Oops can significantly reduce memory footprint, especially for applications that require a large heap.
Conclusion
In this article, we explored essential JVM arguments that can help maximize JVM memory usage and avoid OutOfMemoryError. Properly configuring JVM memory is crucial for application performance and stability.
By understanding and utilizing the right JVM arguments, such as -Xmx
, -Xms
, XX:MaxMetaspaceSize
, XX:NewSize
, XX:MaxNewSize
, XX:SurvivorRatio
, XX:G1HeapRegionSize
, and XX:+UseCompressedOops
, you can optimize memory usage and achieve better application performance.
Remember to experiment with different configurations and parameter values to find the optimal memory settings for your specific application workload.
Checkout our other articles