Creating a thread without implementing Runnable interface in Java
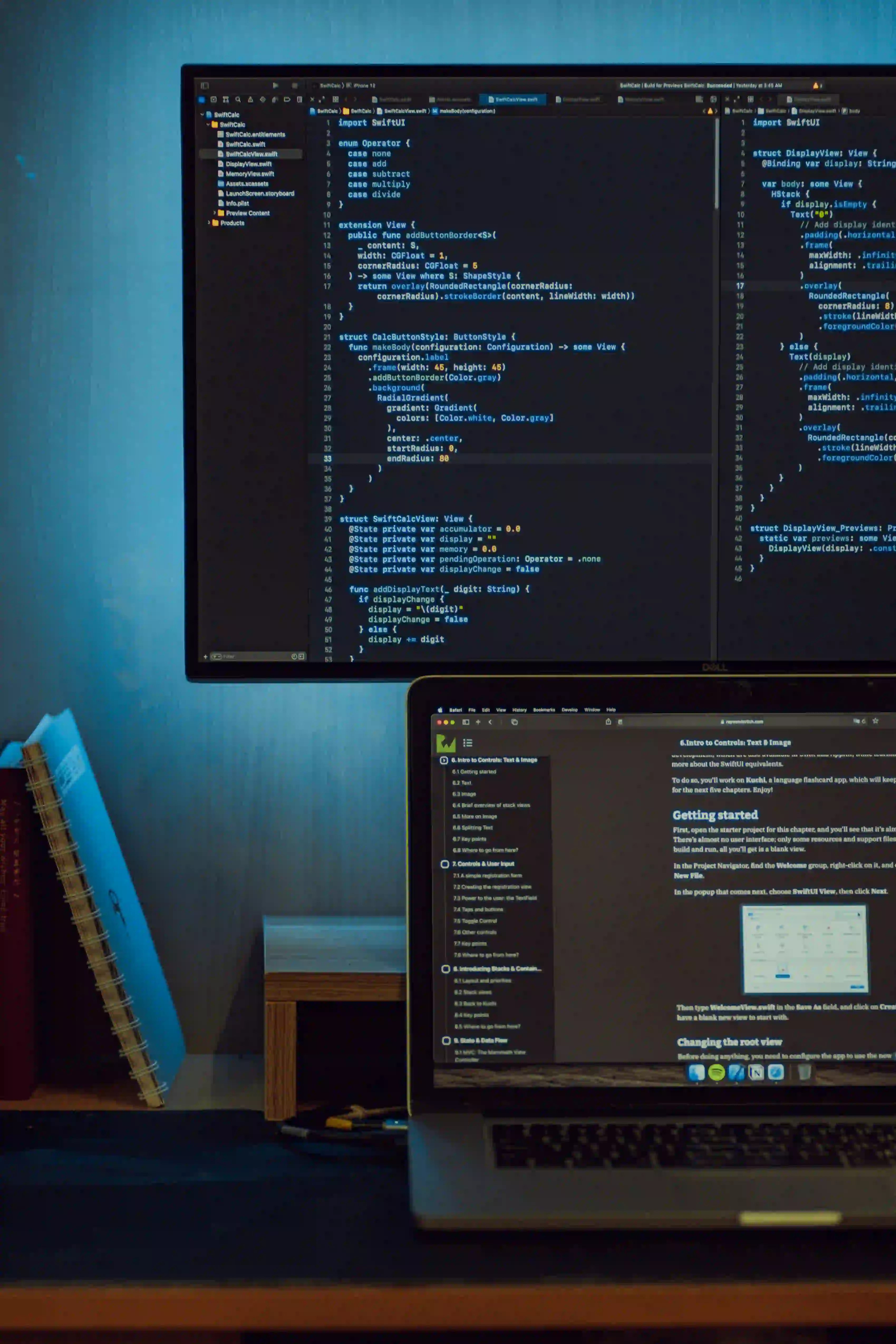
Introduction
In Java, multithreading is an essential concept for building concurrent and parallel applications. Traditionally, to create a new thread, developers would implement the Runnable
interface and override the run()
method. However, there is an alternative way to create a thread without implementing the Runnable
interface in Java.
In this article, we will explore how to create a thread without implementing the Runnable
interface and discuss the advantages and disadvantages of this approach.
The Thread class
In addition to implementing the Runnable
interface, Java provides another way to create a thread by extending the Thread
class. The Thread
class is a built-in class in Java that represents a thread of execution. By extending this class, we can directly create threads without having to implement the Runnable
interface.
To create a thread by extending the Thread
class, we need to follow these steps:
- Create a new class that extends the
Thread
class. - Override the
run()
method, which is the entry point for the thread's execution.
Here's an example that demonstrates how to create a thread by extending the Thread
class:
public class MyThread extends Thread {
@Override
public void run() {
// The code to be executed in the new thread
System.out.println("Running in a new thread");
}
}
In the above example, we have created a new class MyThread
that extends the Thread
class. We have overridden the run()
method to define the code that will be executed in the new thread.
To start the thread, we need to create an instance of the MyThread
class and invoke its start()
method:
public class Main {
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start();
// The code executing in the main thread
System.out.println("Running in the main thread");
}
}
When we invoke the start()
method, it creates a new thread and invokes the run()
method in the new thread concurrently with the main thread.
Advantages of extending the Thread class
Using the Thread
class to create a thread has certain advantages:
-
Simplicity: Extending the
Thread
class allows you to define the code to be executed in the new thread directly within the class itself. This eliminates the need to separately implement theRunnable
interface and provide an instance of it to theThread
constructor. -
Object-oriented approach: By extending the
Thread
class, you can encapsulate the behavior of the thread within a class, making it easier to manage and maintain. -
Inheritance: Extending the
Thread
class allows you to override other methods and add additional functionalities as needed.
Disadvantages of extending the Thread class
While extending the Thread
class to create a thread has its advantages, it also has some disadvantages:
-
Limited inheritance: Java does not support multiple inheritance, so by extending the
Thread
class, you are prohibiting your class from inheriting any other class. This can be a limitation if your class needs to inherit from another class. -
Lack of flexibility: The
Thread
class defines a fixed interface for creating and managing a thread. If you need more control over the thread's execution or want to implement a different concurrency mechanism, theRunnable
interface provides more flexibility. -
Performance impact: Each new thread created by extending the
Thread
class consumes system resources, such as memory. Creating a large number of threads can affect the overall performance of the application, especially in resource-constrained environments.
Conclusion
In Java, creating a thread without implementing the Runnable
interface is possible by extending the Thread
class. While this approach offers simplicity and an object-oriented approach, it also has limitations and may not be suitable for all scenarios.
When deciding whether to implement Runnable
or extend Thread
, consider the specific requirements of your application. If you need more flexibility, better code organization, and the ability to use multiple inheritance, implementing Runnable
is a better choice. On the other hand, if simplicity and encapsulation are more important, extending the Thread
class can be a viable option.
Understanding the pros and cons of both approaches will help you make an informed decision while designing concurrent and parallel applications in Java.