Troubleshooting: Eclipse Deeplearning4j Neural Network Training Issues
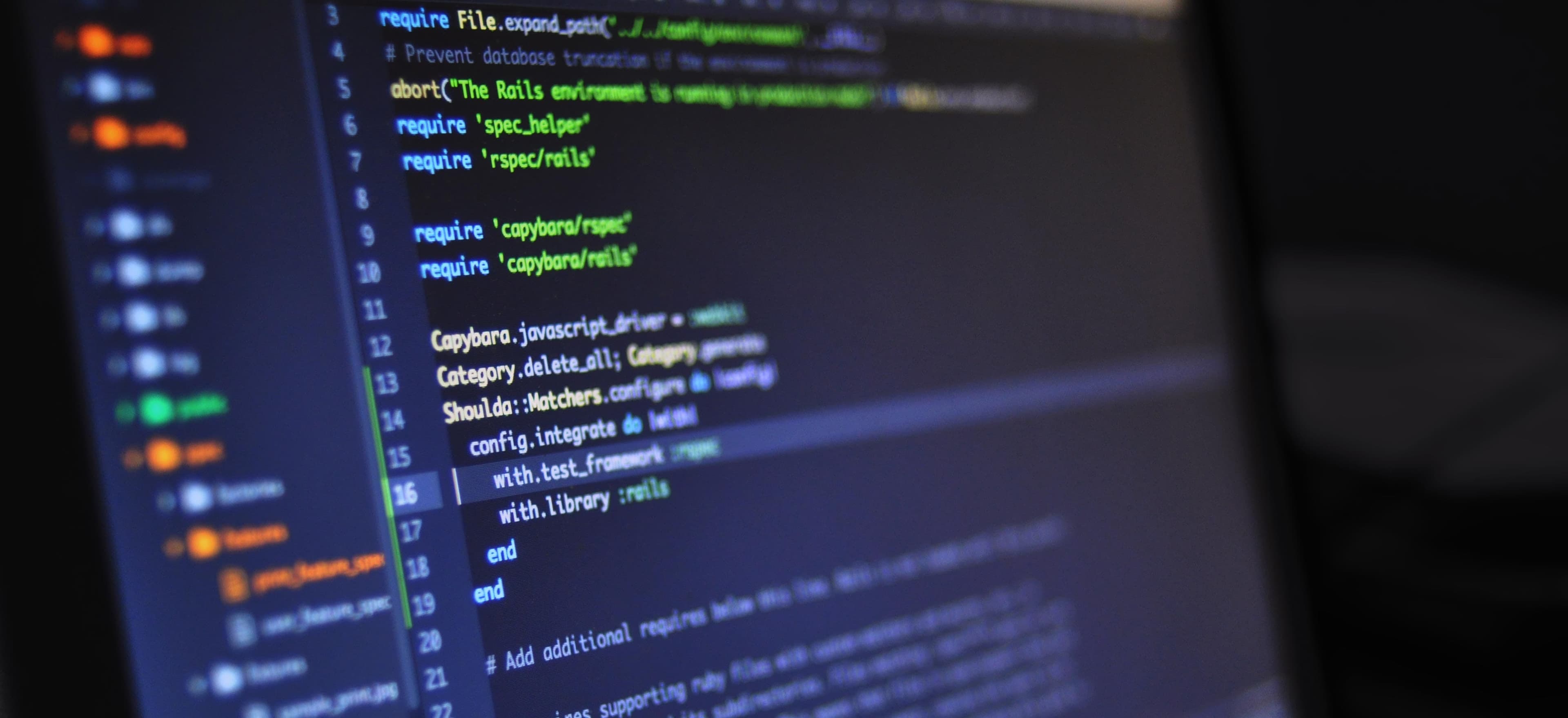
- Published on
Introduction
Eclipse Deeplearning4j (DL4J) is a powerful open-source deep learning library for the Java programming language. It provides tools for building, training, and deploying neural networks. However, like any software, DL4J can encounter issues during training. In this article, we will explore some common troubleshooting techniques for resolving neural network training issues in DL4J.
Common Issues
1. Vanishing/Exploding Gradients
Vanishing or exploding gradients are a common issue in deep learning, where the gradients in the neural network become very small or very large. This can make the training process unstable or prevent convergence altogether. One possible cause of this issue is improper weight initialization.
To resolve this, try using different weight initialization strategies. For example, the Xavier or He initialization methods are often recommended for deep neural networks. These initialization methods ensure that the weights are initialized in a way that prevents vanishing or exploding gradients.
Here is an example of using He initialization in DL4J:
NeuralNetConfiguration.ListBuilder builder = new NeuralNetConfiguration.Builder()
.weightInit(WeightInit.HE)
// other configuration options
.build();
2. Overfitting
Overfitting occurs when a model performs well on the training data but fails to generalize to new, unseen data. This can happen if the model becomes too complex or if there is not enough training data. Overfitting can lead to poor performance and low accuracy.
To address overfitting, you can try the following techniques:
- Increase the amount of training data or use data augmentation techniques to artificially expand the dataset.
- Reduce the complexity of the model by removing unnecessary layers or using regularization techniques such as L1 or L2 regularization.
- Use techniques like dropout or batch normalization to improve generalization.
3. Underfitting
Underfitting is the opposite of overfitting. It occurs when the model fails to capture the underlying patterns in the data. This can happen if the model is too simple or if there is not enough training data.
To address underfitting, you can try the following techniques:
- Increase the complexity of the model by adding more layers or increasing the number of units in each layer.
- Use a more powerful model architecture, such as a convolutional neural network (CNN) for image data or a recurrent neural network (RNN) for sequential data.
- Ensure that the model has enough capacity to learn the patterns in the data by checking the model's capacity and adjusting it accordingly.
4. Slow Training
Training a deep neural network can be computationally expensive and time-consuming. If you are experiencing slow training, there are several possible causes.
One common cause of slow training is inefficient data loading. Make sure that you are using efficient data loading techniques, such as using batching and parallelization. DL4J provides tools for efficient data loading, such as the RecordReaderDataSetIterator
class.
Another possible cause of slow training is improper hardware utilization. Ensure that you are using a GPU if available, as it can significantly speed up the training process for deep neural networks.
Lastly, check your implementation for any unnecessary computation or redundant operations. Optimizing the code can greatly improve training speed.
5. NaN Loss/Cost Function
If you encounter a NaN (Not-a-Number) loss or cost function during training, it usually indicates a problem with the model's configuration or the data being used. Here are a few possible causes and solutions:
- NaN values in the input data: Check the input data for any NaN values and handle them appropriately, such as replacing them with a default value or removing the corresponding samples.
- Incorrect loss function: Ensure that you are using the correct loss function for your task. For example, if you are working on a classification problem, you should be using a softmax cross-entropy loss.
- Incorrect data preprocessing: Double-check your data preprocessing steps to make sure that they are performed correctly. Normalize or standardize your input data if necessary.
Conclusion
Troubleshooting neural network training issues in Eclipse Deeplearning4j can be challenging, but with the right knowledge and techniques, it is possible to resolve common issues and achieve successful training. By understanding the underlying problems and applying appropriate solutions, you can improve the stability, performance, and efficiency of your deep learning models. Happy training!
Checkout our other articles