Handling Large Message Volume in JMS with AWS SQS
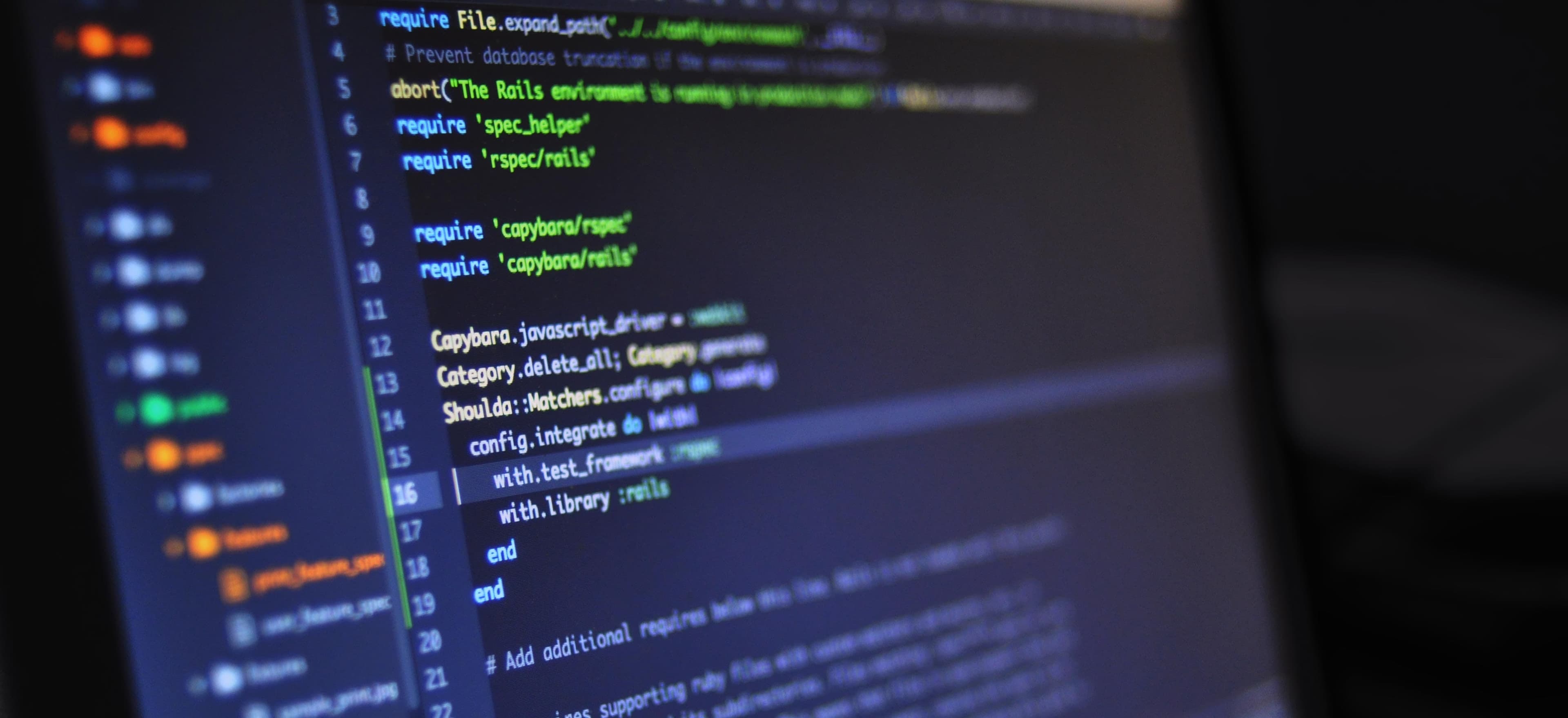
- Published on
Introduction
In today's world, with the increasing demand for real-time data processing, handling large message volumes efficiently has become a crucial aspect of many applications. Java Message Service (JMS) is a popular messaging standard that provides a reliable way to exchange messages between systems. However, when dealing with high message volumes, traditional JMS implementations may face scalability and performance challenges. That's where Amazon Simple Queue Service (SQS) comes to the rescue.
Amazon SQS is a fully managed message queuing service provided by Amazon Web Services (AWS). It offers a highly scalable and reliable infrastructure to decouple the components of a distributed system, allowing applications to communicate asynchronously. In this blog post, we will explore how to handle large message volumes effectively using JMS with Amazon SQS.
Prerequisites
To follow along with the examples and code snippets in this blog post, you will need the following:
- Java Development Kit (JDK) 8 or later
- A valid AWS account
- AWS SDK for Java
Understanding JMS
JMS is a Java API that provides a common interface for sending and receiving messages between applications. It allows applications to send and receive messages in a reliable and asynchronous manner. JMS supports two types of messaging models: point-to-point and publish/subscribe.
In the point-to-point model, messages are sent from a single sender to a single receiver using a queue. The sender puts messages in the queue, and the receiver consumes them from the queue. This model ensures that each message is consumed by only one receiver.
In the publish/subscribe model, messages are sent from a sender to multiple subscribers using a topic. The sender publishes messages to a topic, and all subscribers interested in receiving those messages can subscribe to the topic. This model enables message broadcasting, where multiple receivers can consume the same message.
Why Use Amazon SQS with JMS?
While JMS provides a powerful messaging standard, it may not be suitable for handling large message volumes efficiently, especially when dealing with distributed systems. Here are a few reasons why using Amazon SQS with JMS makes sense:
-
Scalability: Amazon SQS is designed to handle massive message volumes with ease. It automatically scales to accommodate increasing message loads without any configuration changes. This makes it an ideal choice for applications that experience varying message traffic.
-
Reliability: Amazon SQS is a fully managed service that abstracts away the complexities of running and maintaining message queues. It provides a reliable infrastructure that ensures message delivery and durability. Messages are automatically replicated across multiple data centers, providing a high level of fault tolerance.
-
Decoupling Components: Amazon SQS helps decouple the components of a distributed system by introducing queues as intermediaries. This allows individual components to communicate asynchronously, reducing dependencies and increasing system resilience.
-
Back Pressure: Amazon SQS provides back pressure mechanisms to handle high message volumes. When the number of incoming messages exceeds the processing capacity, SQS can automatically throttle the flow of messages to prevent overload and ensure smooth processing.
-
Delay Queues: Amazon SQS supports delay queues, allowing you to specify a delay before a message becomes available for consumption. This feature can be useful in scenarios where you need to control the timing of message processing.
Setting Up Amazon SQS
To get started with Amazon SQS, you need to create a queue in the AWS Management Console. Follow these steps:
- Open the AWS Management Console and navigate to the SQS service.
- Click on "Create Queue" to create a new queue.
- Provide a name for your queue and choose the desired queue type (standard or FIFO). FIFO queues ensure message ordering, while standard queues offer higher throughput.
- Configure additional settings such as message retention period, visibility timeout, etc.
- Click on "Create Queue" to create the queue.
Once your queue is created, you will have access to the queue URL, which you will need to connect to the queue from your Java application.
Integrating JMS with Amazon SQS
To integrate JMS with Amazon SQS, you will need to use the AWS SDK for Java and the JMS API. Here are the steps to configure and use JMS with Amazon SQS:
- Include the necessary dependencies in your project's
pom.xml
orbuild.gradle
file:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>sqs</artifactId>
<version>2.17.25</version>
</dependency>
<dependency>
<groupId>javax.jms</groupId>
<artifactId>javax.jms-api</artifactId>
<version>2.0.1</version>
</dependency>
- Create an instance of the AWS SDK client using your AWS credentials:
AwsCredentials credentials = DefaultCredentialsProvider.create().resolveCredentials();
SqsClient sqsClient = SqsClient.builder()
.region(Region.US_EAST_1)
.credentialsProvider(StaticCredentialsProvider.create(credentials))
.build();
- Create an instance of the SqsConnectionFactory:
SqsConnectionFactory connectionFactory = new SqsConnectionFactory(
new ProviderConfiguration(),
sqsClient);
- Create a JMS connection using the connection factory:
JmsContext jmsContext = connectionFactory.createContext();
- Create a JMS session and specify the destination (queue or topic) you want to send/receive messages from:
JmsSession jmsSession = jmsContext.createSession(false, Session.AUTO_ACKNOWLEDGE);
JmsQueue jmsQueue = jmsSession.createQueue("your-queue-url");
- Create a JMS producer or consumer and start sending/receiving messages:
JmsProducer jmsProducer = jmsSession.createProducer(jmsQueue);
jmsProducer.send(jmsSession.createTextMessage("Hello, world!"));
JmsConsumer jmsConsumer = jmsSession.createConsumer(jmsQueue);
JmsTextMessage message = (JmsTextMessage) jmsConsumer.receive();
System.out.println(message.getText());
Make sure to handle exceptions and close the JMS resources properly to release any held-up resources.
Handling Large Message Volumes
Now, let's take a look at how you can handle large message volumes effectively using JMS with Amazon SQS.
Batch Processing
When dealing with high message volumes, it is often more efficient to process messages in batches rather than individually. Amazon SQS provides a mechanism to receive messages in batches, reducing the overall overhead of sending/receiving requests.
In JMS, you can specify the maximum number of messages to receive in a batch by setting the JMS_IBM_BATCHSIZE
property on the JmsConsumer
object. Here's an example:
jmsConsumer.setIntProperty("JMS_IBM_BATCHSIZE", 10);
In this example, we configure the consumer to receive messages in batches of 10. This can significantly improve the throughput of your application when processing large volumes of messages.
Parallelism
To further increase the processing speed when handling large message volumes, you can introduce parallelism in your application. By using multiple consumers, you can process messages concurrently, leveraging the power of multi-core systems.
In JMS, each consumer runs in its own thread, allowing you to achieve parallelism easily. Here's an example of creating multiple consumers:
JmsConsumer consumer1 = jmsSession.createConsumer(jmsQueue);
JmsConsumer consumer2 = jmsSession.createConsumer(jmsQueue);
You can create as many consumers as you need, depending on the desired level of parallel processing.
Prefetching
Prefetching is a technique that allows consumers to fetch and cache a certain number of messages in advance. This helps to minimize network latency and improve the overall processing time, especially when messages are small in size.
In JMS, you can set the maximum number of messages to prefetch using the JMS_IBM_MQ_PREFETCH_SIZE
property on the JmsConsumer
object. Here's an example:
jmsConsumer.setIntProperty("JMS_IBM_MQ_PREFETCH_SIZE", 100);
In this example, we configure the consumer to prefetch up to 100 messages at a time. Adjusting this value based on your message size and network latency can have a significant impact on the overall performance.
Dead Letter Queues
When handling large volumes of messages, it is common to encounter failures or errors during message processing. To handle such situations gracefully, you can leverage Amazon SQS's dead letter queues feature.
A dead letter queue is a separate queue that receives messages that were not successfully processed after a certain number of retries. By redirecting failed messages to a dead letter queue, you can analyze and handle them separately, without impacting the regular message flow.
To configure a dead letter queue, you need to specify the RedrivePolicy
attribute when creating a queue. The RedrivePolicy
specifies the number of message delivery attempts before a message is considered dead and the Amazon SQS queue URL of the dead letter queue.
Here's an example of creating a queue with a dead letter queue:
CreateQueueRequest request = CreateQueueRequest.builder()
.queueName("your-queue-name")
.attributes(Map.of(
"RedrivePolicy",
"{\"maxReceiveCount\":\"5\",\"deadLetterTargetArn\":\"arn:aws:sqs:us-east-1:123456789012:your-deadletter-queue\"}"))
.build();
sqsClient.createQueue(request);
In this example, the maxReceiveCount
attribute is set to 5, which means that a message will be retried up to 5 times before being considered dead. The deadLetterTargetArn
attribute specifies the ARN (Amazon Resource Name) of the dead letter queue.
By leveraging dead letter queues, you can handle message failures more robustly and ensure that messages are not lost due to processing errors.
Conclusion
In this blog post, we explored how to handle large message volumes efficiently using JMS with Amazon SQS. We discussed the benefits of using Amazon SQS for scalable and reliable messaging, and we learned how to integrate JMS with Amazon SQS. Additionally, we looked at various strategies for handling large message volumes, such as batch processing, parallelism, prefetching, and dead letter queues.
By leveraging the power of JMS with Amazon SQS, you can build robust and scalable messaging systems that can handle high message volumes effectively. Whether you're building real-time data processing pipelines, event-driven architectures, or any other distributed application, JMS with Amazon SQS is a powerful combination worth considering.
Checkout our other articles