Improving Efficiency of Data Aggregation in Spring Data MongoDB
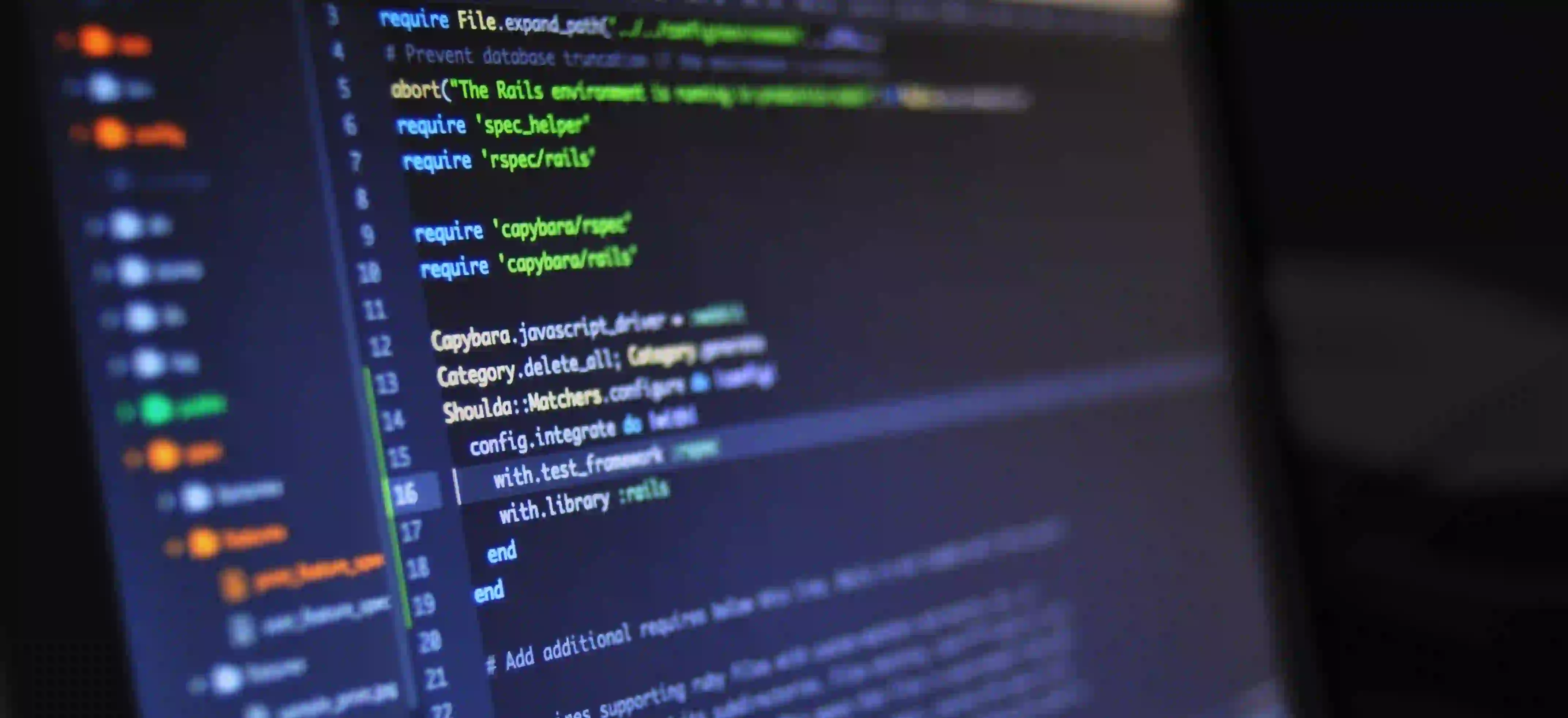
Improving Efficiency of Data Aggregation in Spring Data MongoDB
Introduction
In today's data-driven world, efficient data aggregation is crucial for extracting valuable insights from large datasets. MongoDB, a popular NoSQL database, provides powerful aggregation capabilities that allow us to process and transform data at scale. In this article, we will explore how we can improve the efficiency of data aggregation in Spring Data MongoDB.
Understanding Data Aggregation
Data aggregation in MongoDB involves performing a set of operations on a collection of documents to produce a single result. These operations can include grouping, sorting, filtering, and transforming data. Spring Data MongoDB provides a convenient and intuitive interface for performing data aggregation queries using the MongoDB aggregation pipeline.
The MongoDB aggregation pipeline is a framework for data aggregation that allows us to build multi-stage transformations of data. Each stage in the pipeline performs a specific operation on the input data and passes the result to the next stage. This allows us to compose complex data aggregation queries by chaining multiple stages together.
Improving Efficiency
Efficiency is crucial when dealing with large datasets. Here are some tips and techniques to improve the efficiency of data aggregation in Spring Data MongoDB:
1. Indexing
Indexing plays a crucial role in improving query performance. By creating appropriate indexes on the fields used in the aggregation pipeline stages, we can significantly speed up the data aggregation process. MongoDB provides various types of indexes, such as single-field indexes, compound indexes, and multi-key indexes. Analyze the aggregation query and create indexes to support the query predicates and sort operations.
@Document(collection = "myCollection")
@CompoundIndexes({
@CompoundIndex(name = "index_name", def = "{'field1': 1, 'field2': 1}")
})
public class MyEntity {
// ...
}
2. Projection
In some cases, we may only need a subset of the fields in the documents being aggregated. By using projection, we can include only the required fields in the output document. This reduces the amount of data transferred over the network and improves performance. To specify which fields to include or exclude, we can use the Aggregation.project()
method.
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.project("name", "age")
// ...
);
3. Filtering
To improve efficiency, it's important to filter out unnecessary documents early in the aggregation pipeline. This reduces the number of documents that need to be processed and improves query performance. We can use the $match
stage in the pipeline to filter documents based on specific criteria.
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.match(Criteria.where("age").gte(18))
// ...
);
4. Sorting
Sorting large result sets can be resource-intensive. To improve performance, it's recommended to sort the data as early as possible in the aggregation pipeline. This reduces the amount of data that needs to be sorted and improves query performance. We can use the $sort
stage in the pipeline to sort documents based on one or more fields.
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.sort(Sort.by("name").ascending())
// ...
);
5. Limiting and Skipping Results
In some cases, we may only need a subset of the aggregated results. To improve performance, we can limit the number of documents returned by using the $limit
stage in the pipeline. Additionally, we can skip a certain number of documents using the $skip
stage. These stages can help reduce the amount of data transferred over the network and improve query performance.
Aggregation aggregation = Aggregation.newAggregation(
Aggregation.limit(10),
Aggregation.skip(20)
// ...
);
6. Avoid Unnecessary Stages
Avoid unnecessary stages in the aggregation pipeline to improve query performance. Carefully analyze the aggregation query and remove any stages that are not required. This reduces the processing overhead and improves the overall efficiency of the data aggregation process.
7. Caching
If the aggregation query results don't change frequently, consider caching the results. Caching can significantly improve the performance of subsequent queries by avoiding the expensive data aggregation process. Spring Data MongoDB provides caching support through the use of caching annotations, such as @Cacheable
and @CachePut
.
Conclusion
Efficient data aggregation is essential for extracting valuable insights from large datasets. In this article, we explored various techniques to improve the efficiency of data aggregation in Spring Data MongoDB. By leveraging indexing, projection, filtering, sorting, and other optimization techniques, we can significantly improve the performance of data aggregation queries. Additionally, caching can be used to further enhance query performance. By following these best practices, we can make the most of MongoDB's powerful aggregation capabilities and unlock the full potential of our data.