Preventing Communication Overload: The Power of Mediator Pattern
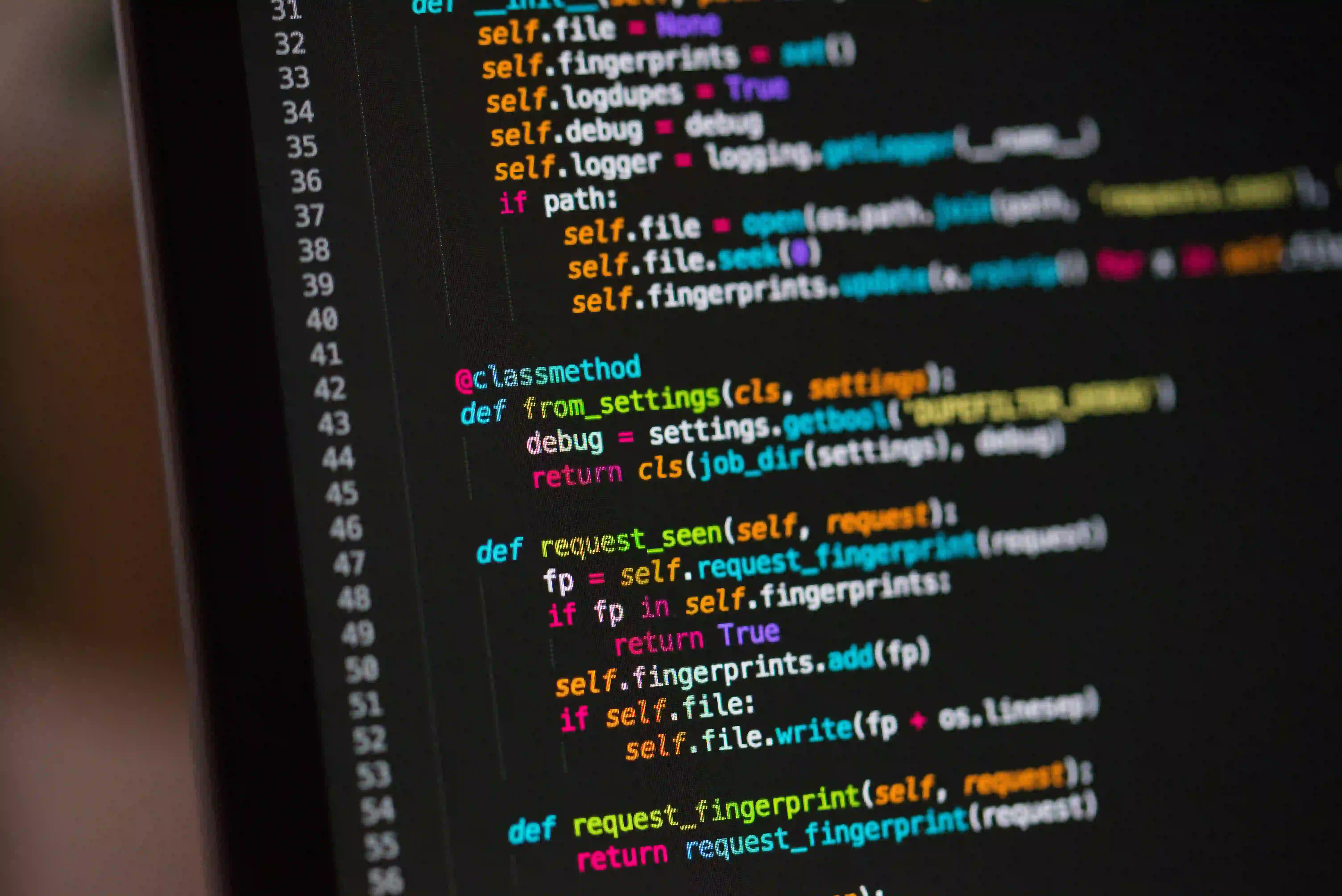
Introduction
In today's fast-paced world of software development, it's not uncommon to encounter complex systems with numerous components that need to communicate with each other. As the number of components grows, ensuring effective communication and avoiding communication overload becomes a challenge. This is where the Mediator pattern comes in handy.
The Mediator pattern is a behavioral design pattern that allows for loose coupling between components by encapsulating communication logic in a mediator object. It enables components to communicate with each other without having to explicitly know about each other.
In this article, we will explore the Mediator pattern in Java and understand how it can help prevent communication overload in software systems.
Understanding the Mediator Pattern
At its core, the Mediator pattern promotes a centralized approach to handling communication between components. Instead of components directly communicating with each other, they communicate through a mediator object.
The mediator object encapsulates the communication logic and knows about the individual components and how to facilitate their communication. It acts as a hub and allows components to communicate with each other without being aware of the specific implementation details of other components.
By introducing a mediator, components can be decoupled from each other, reducing dependencies and improving reusability. This makes the overall system more maintainable and extensible.
When to Use the Mediator Pattern
The Mediator pattern is most effective when:
- There are multiple components in a system that need to communicate with each other.
- The components are tightly coupled and have direct references to each other, resulting in a high degree of dependencies.
- Adding new components or modifying existing components leads to cascading changes in other components.
By introducing a mediator object, these issues can be mitigated, allowing for more flexible and scalable software systems.
Implementing the Mediator Pattern in Java
Let's dive into an example to understand how the Mediator pattern can be implemented in Java. Imagine a chat application where multiple users can send messages to each other. We'll create a simplified version of this application using the Mediator pattern.
First, let's define the components involved in the communication:
- User: Represents a user of the chat application.
- ChatMediator: Acts as a mediator between users and facilitates communication.
- ChatRoom: Implements the communication logic and keeps track of users and their messages.
Here's how the code for these components might look like:
// User.java
public class User {
private String name;
private ChatMediator chatMediator;
public User(String name, ChatMediator chatMediator) {
this.name = name;
this.chatMediator = chatMediator;
}
public void sendMessage(String message) {
chatMediator.sendMessage(message, this);
}
public void receiveMessage(String message) {
System.out.println(name + " received message: " + message);
}
}
// ChatMediator.java
public interface ChatMediator {
void sendMessage(String message, User user);
}
// ChatRoom.java
public class ChatRoom implements ChatMediator {
private List<User> users;
public ChatRoom() {
users = new ArrayList<>();
}
public void addUser(User user) {
users.add(user);
}
@Override
public void sendMessage(String message, User sender) {
for (User user : users) {
if (user != sender) {
user.receiveMessage(message);
}
}
}
}
In the above code, the User
class represents a user of the chat application. Each user has a reference to the ChatMediator
object, which acts as a mediator between users. When a user sends a message, it calls the sendMessage
method on the ChatMediator
, passing the message and a reference to itself.
The ChatMediator
interface defines the contract for the mediator, with a single method sendMessage
that takes a message and a user. The ChatRoom
class implements the ChatMediator
interface and maintains a list of users. When a message is sent, the ChatRoom
iterates over all users (excluding the sender) and calls the receiveMessage
method on each user.
By using the Mediator pattern, the User
class doesn't need to know about the implementation details of other users or the chat room. It simply delegates the responsibility of message delivery to the ChatMediator
.
Advantages of Using the Mediator Pattern
The Mediator pattern offers several advantages when it comes to managing communication between components:
- Decoupling: Components don't have direct dependencies on each other, reducing coupling and improving maintainability.
- Reusability: The mediator object can be reused across different components, promoting code reusability.
- Scalability: Adding new components becomes easier as they only need to communicate through the mediator object, without impacting existing components.
- Centralized Logic: The mediator object encapsulates the communication logic, making it easier to manage and modify if necessary.
Limitations of the Mediator Pattern
While the Mediator pattern has its benefits, it's important to consider some limitations:
- Increased Complexity: Introducing a mediator object adds an extra layer of complexity to the system, which may not be necessary for smaller projects.
- Single Point of Failure: The mediator object becomes a single point of failure. If it fails, the entire communication between components breaks.
- Performance Overhead: The mediator object acts as a middleman, introducing additional method calls and potentially impacting performance in large-scale systems.
It's crucial to evaluate the trade-offs before deciding to use the Mediator pattern in a software system.
Conclusion
The Mediator pattern provides an effective solution for managing communication between components in software systems. By introducing a mediator object, components can communicate without knowing about each other, reducing coupling and improving flexibility.
In this article, we explored the Mediator pattern in Java and saw how it can be used to prevent communication overload. We implemented a simplified chat application to demonstrate the pattern in action and discussed its advantages and limitations.
Next time you find yourself dealing with a complex system with tightly coupled components, consider using the Mediator pattern to simplify communication and improve maintainability.