The Limitations of Spring Boot Autoconfiguration
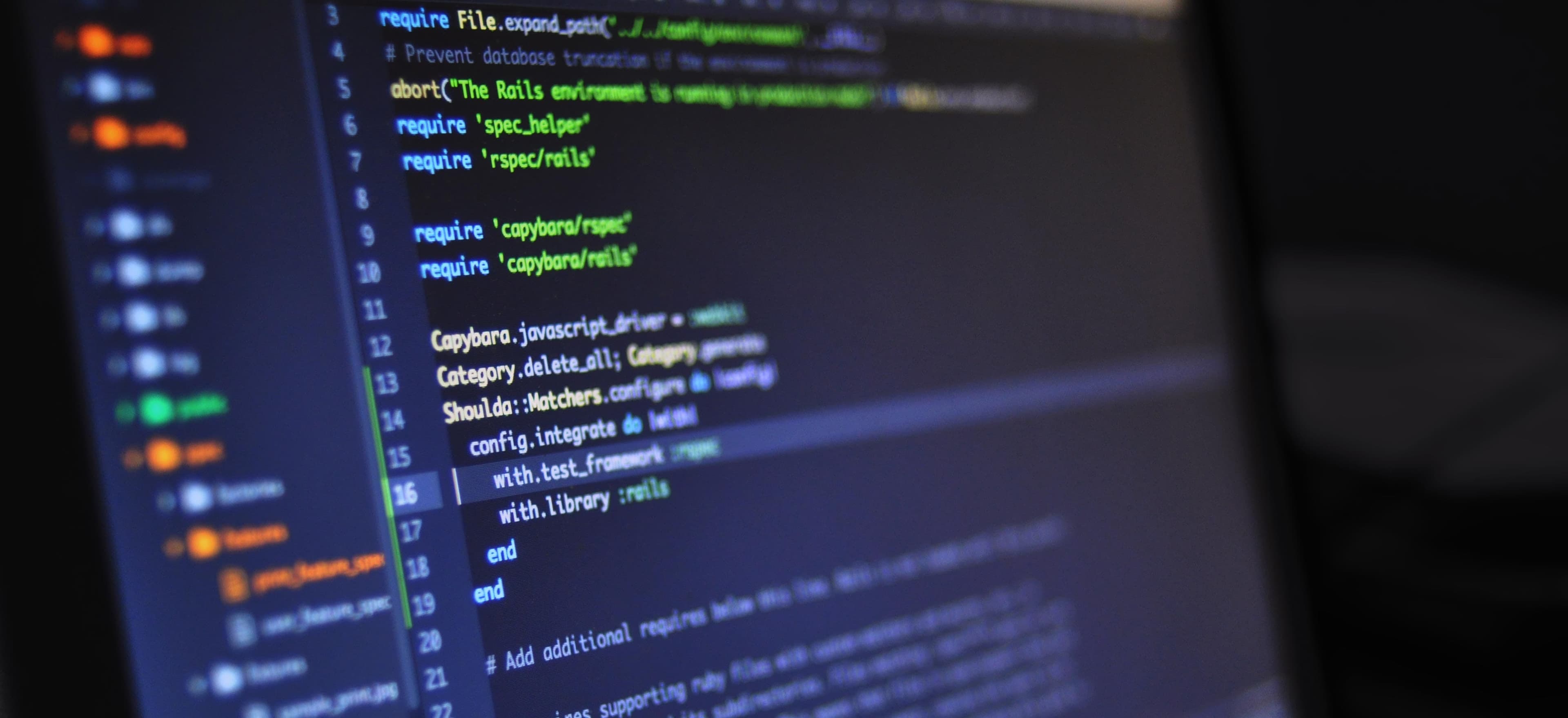
- Published on
Introduction
Spring Boot has gained immense popularity over the years for its ease of use and convention-over-configuration approach. One of the key features that makes Spring Boot so appealing is its autoconfiguration capability. Autoconfiguration allows Spring Boot to automatically configure various components and dependencies based on the user's classpath and properties files. While this feature provides significant convenience, it also has its limitations. In this article, we will explore some of the limitations of Spring Boot's autoconfiguration and how to overcome them.
Understanding Spring Boot Autoconfiguration
Before diving into the limitations, let's briefly understand how Spring Boot's autoconfiguration works. When you include a starter dependency in your Spring Boot application, it brings in a set of pre-configured dependencies and sets up sensible defaults for them. These starters are nothing but collections of dependencies that are commonly used together in specific types of applications.
For example, if you include the spring-boot-starter-web
dependency, Spring Boot sets up the necessary configurations for building a web application, such as configuring an embedded servlet container, registering the necessary servlets and filters, and setting up default error handling.
This automated configuration is achieved using Spring Boot's autoconfiguration mechanism. Spring Boot scans the classpath for various configuration classes, known as @Configuration
classes, and applies the configurations defined in these classes if the corresponding dependencies are present.
Limitations of Spring Boot Autoconfiguration
While Spring Boot's autoconfiguration is a powerful feature that can save you a lot of time and effort, it does have its limitations. Let's take a look at some of the common limitations:
Limited Control Over Configuration
With autoconfiguration, Spring Boot tries to provide sensible defaults and automatic configurations without requiring explicit coding from the developer. While this is convenient in most cases, it can limit your control over the configuration. In some scenarios, you may need to customize certain configurations to fit your specific needs.
For example, if you need to configure a specific behavior of an embedded servlet container, the autoconfiguration may not provide enough options. In such cases, you may have to resort to manually configuring the container, bypassing the autoconfiguration.
Conflicting Configurations
Autoconfiguration relies on the presence of certain dependencies on the classpath to trigger the corresponding configurations. However, if you have conflicting configurations due to multiple dependencies providing similar features, it can lead to unpredictable behavior.
For instance, if you include both the spring-boot-starter-webflux
and spring-boot-starter-web
dependencies, both of which provide web-related configurations, Spring Boot may struggle to determine which configuration to apply. This can result in unexpected behavior and difficult-to-diagnose issues.
To avoid conflicting configurations, it is important to be aware of the dependencies you are including in your project and their overlapping features. You may need to manually exclude certain configurations or use conditional annotations to enforce specific configurations explicitly.
Customized Conditional Configuration
Spring Boot's autoconfiguration provides a set of predefined conditions that can be used to enable or disable the automatic configuration for certain dependencies. However, these predefined conditions may not always fulfill your specific requirements.
In such cases, you may need to define your own custom conditions to control the autoconfiguration process. This requires a deeper understanding of the Spring Boot internals and can be quite complex for beginners.
Limited Extensibility
While Spring Boot's autoconfiguration covers a wide range of popular technologies and libraries, it may not include configurations for less common or niche dependencies. If you are working with such dependencies, you may have to manually configure them, as there might not be an autoconfiguration available out-of-the-box.
This limitation can be challenging if you are working with a complex application that heavily relies on niche technologies. You may need to invest more time and effort into understanding and configuring these dependencies manually.
Overcoming the Limitations
Although Spring Boot's autoconfiguration has its limitations, there are ways to overcome them. Here are a few approaches:
Explicit Configuration
If you find that the autoconfiguration is not meeting your specific requirements, you can opt for explicit configuration. This involves manually defining the necessary configurations in your own @Configuration
classes.
By creating your own configuration classes, you have full control over the configuration process. You can override the default configurations provided by Spring Boot's autoconfiguration or define entirely new configurations to fit your needs.
Conditional Configuration
Using conditional annotations, such as @ConditionalOnProperty
or @ConditionalOnClass
, you can selectively enable or disable autoconfiguration based on specific conditions. This allows you to fine-tune the autoconfiguration process and avoid conflicting configurations.
Conditional annotations provide flexibility in controlling which configurations should be applied based on the presence or absence of certain properties, classes, or other conditions. By leveraging these annotations effectively, you can ensure that only the desired configurations are applied.
Custom Autoconfiguration
In some cases, you may need to create your own custom autoconfiguration classes to handle specific dependencies or configurations that are not covered by Spring Boot's autoconfiguration. Custom autoconfiguration allows you to extend the existing autoconfiguration mechanism and provide your own configurations tailored to your application's needs.
By implementing the AutoConfigurationImportFilter
interface or extending the AutoConfigureAfter
or AutoConfigureBefore
classes, you can define the order in which your custom autoconfiguration is applied and override or complement the existing autoconfiguration.
Conclusion
Spring Boot's autoconfiguration is a powerful feature that simplifies the setup and configuration of your applications. However, it has its limitations, which can be addressed with explicit configuration, conditional configuration, or custom autoconfiguration. By understanding these limitations and applying the appropriate strategies, you can overcome the limitations and achieve greater control over the configuration process in your Spring Boot applications.
Checkout our other articles