Maximizing Error Handling Efficiency with Smaller Try Blocks
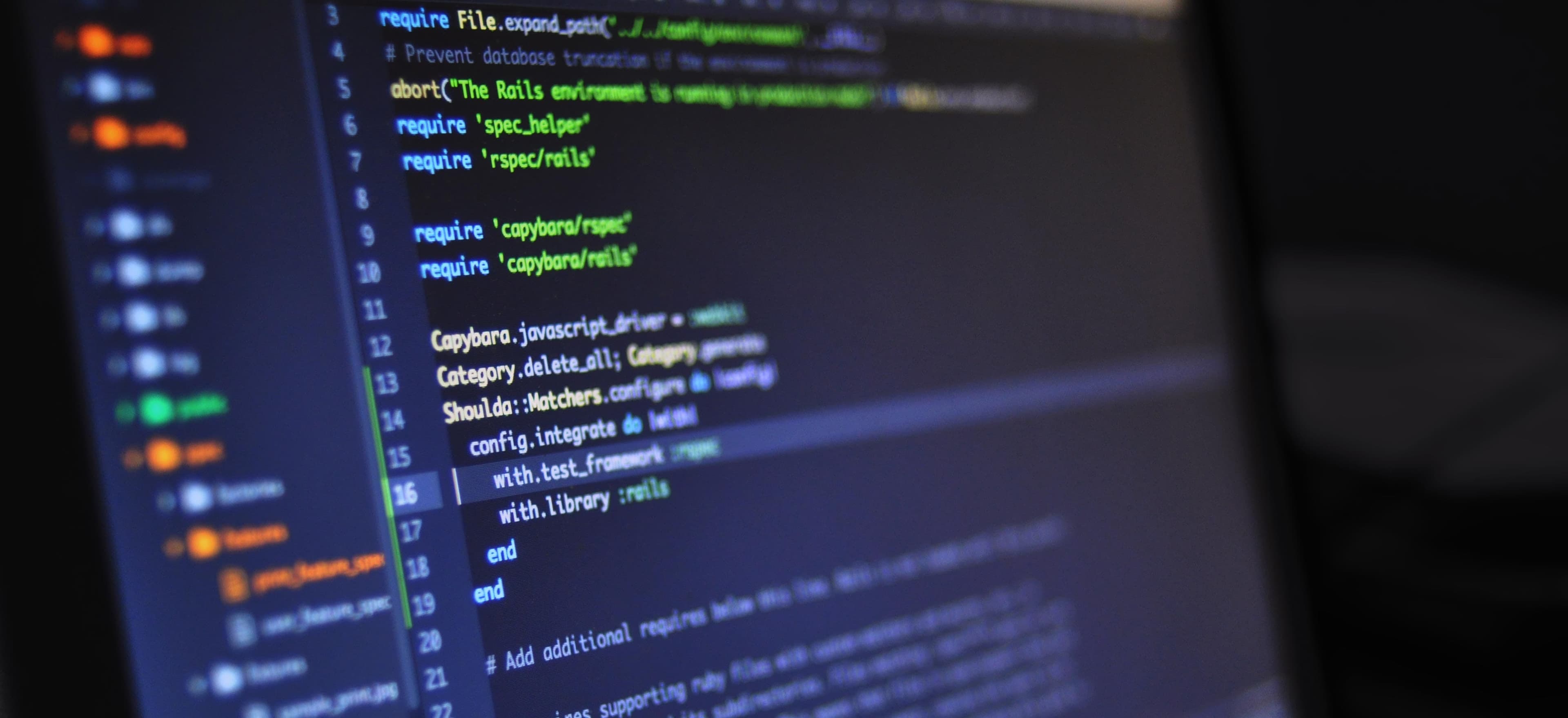
- Published on
Introduction
Error handling is an essential part of any well-written Java code. It ensures that your application can gracefully handle unexpected or exceptional situations, preventing crashes and providing a better user experience.
In Java, the try-catch
block is used to handle exceptions. However, using large try-catch
blocks can make your code harder to read and maintain. In this article, we'll explore the benefits of smaller try
blocks and how they can maximize error handling efficiency in your Java code.
The Problem with Large Try Blocks
Typically, when an exception is thrown within a try
block, the flow of control jumps directly to the corresponding catch
block. This can result in a significant amount of code being skipped, making it difficult to identify the exact location of the error.
Consider the following code snippet:
try {
// Several lines of code
// ...
// Code that may throw an exception
// ...
// Several more lines of code
// ...
} catch (Exception e) {
// Exception handling code
// ...
}
In this example, if an exception is thrown within the try
block, the flow of control jumps directly to the catch
block, skipping the remaining lines of code. While this can be useful in some cases, it can also hide potential issues and make debugging more difficult.
Benefits of Smaller Try Blocks
By dividing your code into smaller try
blocks, you can isolate potential issues and make it easier to identify the exact location of an error. This approach offers several benefits:
1. Improved Code Readability
Smaller try
blocks make your code more readable by making it clear which specific sections of code can throw exceptions. This can help other developers easily understand the error handling logic without having to analyze large blocks of code.
2. Easier Debugging
With smaller try
blocks, you can pinpoint the exact location of an error more quickly. When an exception is thrown, the stack trace provided by Java will indicate the specific try
block where the exception occurred, narrowing down the possible problem areas.
3. Granular Exception Handling
Smaller try
blocks allow for more fine-grained exception handling. You can catch specific types of exceptions and handle them differently depending on the context. This flexibility can lead to more customized and targeted error handling strategies in your application.
4. Minimized Error Cascading
Error cascading occurs when an exception is thrown, caught, and re-thrown within the same try
block or its corresponding catch
block. With smaller try
blocks, you can avoid error cascading and ensure that exceptions are handled appropriately without unnecessary duplication of code.
Best Practices for Smaller Try Blocks
To maximize error handling efficiency with smaller try
blocks, follow these best practices:
1. Identify Sections of Code That Can Throw Exceptions
Review your code and identify sections that are likely to throw exceptions. This can include I/O operations, network requests, database queries, and external API calls. By identifying these sections, you can isolate them within separate try
blocks.
2. Encapsulate Related Code
Group related code together within a single try
block. For example, if you have multiple database queries that are related to a specific feature or operation, consider putting them within the same try
block. This helps maintain code cohesion and makes it easier to understand the purpose of the code within the try
block.
3. Use Multiple Catch Blocks
When using smaller try
blocks, it's important to use multiple catch
blocks to handle different types of exceptions. This allows you to handle each type of exception differently or provide more specific error messages to the user.
For example:
try {
// Code that may throw IOException
} catch (IOException e) {
// Exception handling for IOException
} catch (Exception e) {
// Generic exception handling
}
4. Utilize Finally Blocks
Finally blocks are executed regardless of whether an exception is thrown or not. They are useful for cleaning up resources, such as closing database connections, releasing file handles, or freeing up memory. By including a finally block within each try
block, you ensure that the necessary cleanup is performed, enhancing the overall reliability of your code.
try {
// Code that may throw exceptions
} catch (Exception e) {
// Exception handling
} finally {
// Cleanup code
}
5. Wrap Critical Code in Try Blocks
Wrap critical code that could potentially cause a system failure or impact data integrity within separate try
blocks. This includes operations such as modifying database records, performing file operations, or executing transactions. By isolating this critical code, you can handle exceptions appropriately and prevent catastrophic failures.
Conclusion
By using smaller try
blocks, you can maximize error handling efficiency in your Java code. Not only does it improve code readability and maintainability, but it also makes debugging easier and allows for more fine-grained exception handling. By following the best practices outlined in this article, you can ensure that your code is robust and resilient, providing a better user experience and increasing the overall reliability of your application.
Checkout our other articles