Optimizing Performance in Spring RESTful APIs with Spring Data JPA and H2
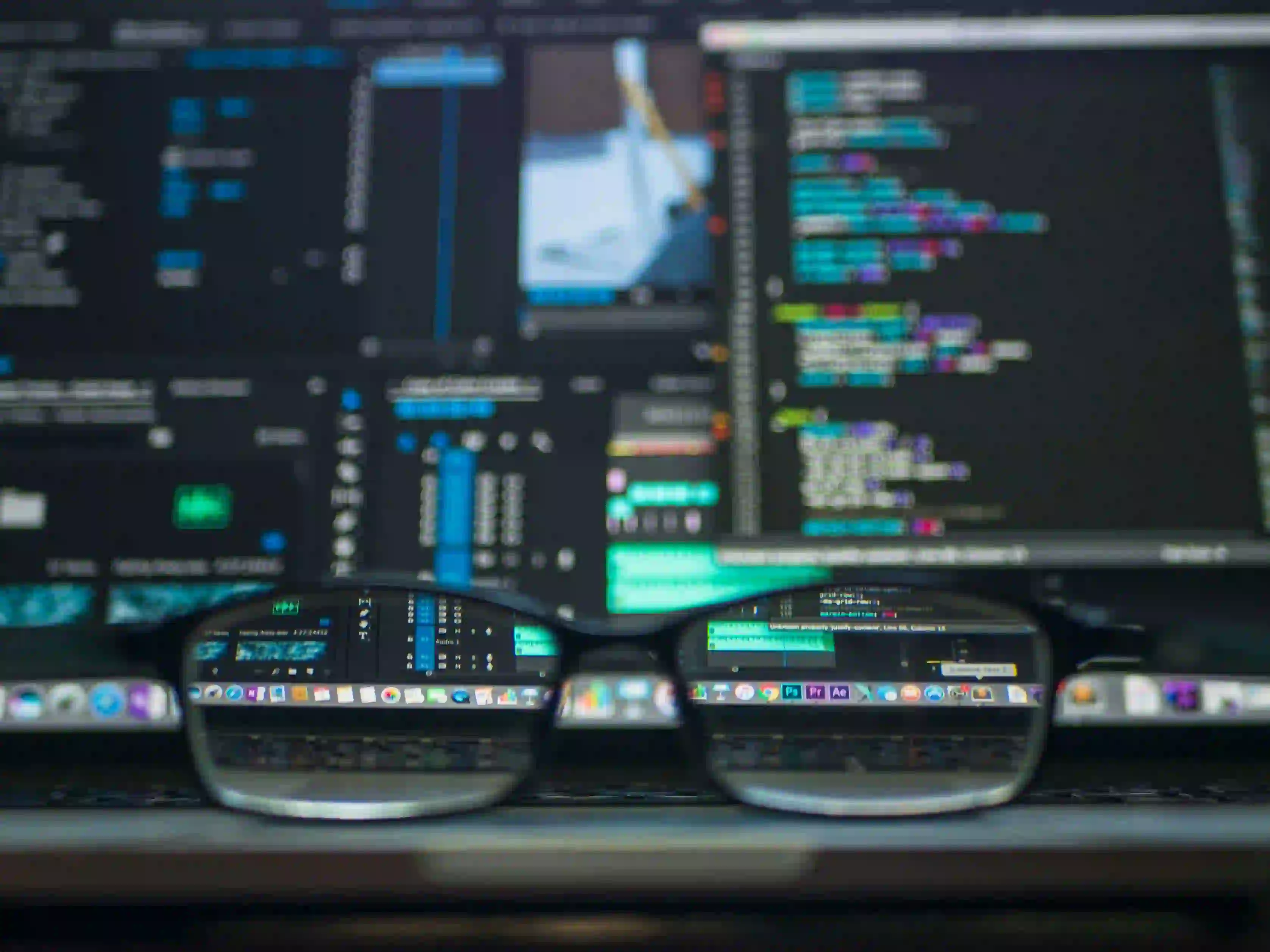
Optimizing Performance in Spring RESTful APIs with Spring Data JPA and H2
Introduction
In today's highly competitive world, performance is a crucial aspect of any software application. When it comes to building RESTful APIs using Spring, optimizing the performance becomes even more important, as these APIs are often used by a large number of users and need to handle high traffic loads efficiently.
In this blog post, we will explore various techniques and best practices for optimizing performance in Spring RESTful APIs using Spring Data JPA and H2, a lightweight in-memory database. We will discuss how to improve the performance of database queries, caching, and handling large data sets efficiently.
Understanding Performance Bottlenecks
Before we jump into optimization techniques, it's essential to identify the performance bottlenecks in your application. Profiling tools like JProfiler or Java Mission Control can be used to identify areas of your code that are taking up more CPU or memory resources. Additionally, you can use tools like Apache JMeter to simulate high traffic loads and measure the response times of your APIs.
Some common performance bottlenecks in RESTful APIs include:
-
Inefficient Database Queries: Poorly optimized database queries can lead to slow API responses. Spring Data JPA provides several options for optimizing queries, such as using query hints, indexing, and pagination.
-
Unoptimized Data Fetching: Loading excessive data from the database can impact the performance of your APIs. Use lazy loading, projections, and pagination to fetch only the required data.
-
Lack of Caching: Not caching frequently accessed data can result in additional database queries and slow down API response times. Spring provides support for various caching mechanisms, including in-memory caching using frameworks like EhCache or Caffeine.
-
Overloading the API: Poorly designed APIs that perform too many operations in a single request can impact performance. Consider breaking down complex operations into smaller, more focused requests.
Optimizing Database Queries with Spring Data JPA
Spring Data JPA simplifies working with databases by providing a set of convenient abstractions and utilities. Here are some tips for optimizing database queries using Spring Data JPA:
1. Use Query Hints
Query hints allow you to provide performance-related instructions to the underlying database. For example, you can use the @QueryHint
annotation to specify a specific index to be used for a particular query, or to enable specific optimizations provided by the database.
@QueryHints(@QueryHint(name = "org.hibernate.readOnly", value = "true"))
List<User> findAllReadOnly();
2. Indexing
Properly indexing your database tables can significantly improve the performance of your queries. Use the @Index
annotation provided by Spring Data JPA to create indexes on specific table columns.
@Entity
@Table(name = "users")
@Index(columnList = "email")
public class User {
// ...
}
3. Pagination
When dealing with large data sets, it's crucial to implement pagination to limit the number of records returned in a single query. Spring Data JPA provides built-in support for pagination using the Pageable
interface.
Page<User> findAll(Pageable pageable);
Caching with Spring
Caching can dramatically improve the performance of your RESTful APIs by reducing the number of database queries. Spring provides support for various caching mechanisms, including in-memory caching using frameworks like EhCache or Caffeine. Here's how you can use caching in Spring:
1. Enable Caching
To enable caching in your Spring application, annotate your service methods or repository methods with the @Cacheable
annotation.
@Service
public class UserService {
@Cacheable("users")
public User getUserById(Long id) {
// ...
}
}
2. Configure Cache Providers
Spring supports different cache providers, such as EhCache, Caffeine, or Redis. You can configure the cache provider using the @EnableCaching
annotation and providing a cache manager bean.
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
// Configure your cache provider here
}
}
3. Evict Cache Entries
Sometimes, you may need to evict specific cache entries when data changes. Spring provides the @CacheEvict
annotation to remove entries from the cache.
@Service
public class UserService {
@CacheEvict("users")
public void updateUser(User user) {
// ...
}
}
Handling Large Data Sets Efficiently
When dealing with large data sets, it's essential to consider techniques for efficient data handling and processing. Here are a few tips for handling large data sets efficiently in Spring RESTful APIs:
1. Use Lazy Loading
Lazy loading is a technique where data is loaded on-demand when accessed by the application. This can help minimize the initial data load and improve API response times. To implement lazy loading with Spring Data JPA, use the @OneToMany
or @ManyToMany
annotations with the fetch = FetchType.LAZY
attribute.
@Entity
public class Post {
// ...
@OneToMany(fetch = FetchType.LAZY, mappedBy = "post")
private List<Comment> comments;
// ...
}
2. Projections
Projections allow you to define custom views of your entity objects, exposing only the required fields. This can help reduce the amount of data transferred over the network and improve performance. Spring Data JPA supports projections using interfaces or DTOs.
public interface PostSummary {
String getTitle();
String getAuthor();
}
public interface PostRepository extends JpaRepository<Post, Long> {
List<PostSummary> findByTitleContaining(String keyword);
}
3. Batch Processing
When dealing with large data sets, processing data in smaller batches rather than all at once can help reduce memory usage and improve performance. Spring provides support for batch processing using the ItemReader
, ItemProcessor
, and ItemWriter
interfaces from the Spring Batch framework.
@Configuration
@EnableBatchProcessing
public class BatchConfig {
@Bean
public ItemReader<User> userItemReader() {
// Configure your user item reader here
}
@Bean
public ItemProcessor<User, User> userItemProcessor() {
// Configure your user item processor here
}
@Bean
public ItemWriter<User> userItemWriter() {
// Configure your user item writer here
}
// ...
}
Conclusion
Optimizing performance is crucial for building high-performance Spring RESTful APIs. In this blog post, we explored various techniques and best practices for optimizing performance in Spring RESTful APIs using Spring Data JPA and H2.
We discussed optimizing database queries with query hints, indexing, and pagination. We also explored caching techniques with Spring, enabling caching, configuring cache providers, and evicting cache entries. Finally, we looked at handling large data sets efficiently using lazy loading, projections, and batch processing.
By following these best practices and techniques, you can significantly improve the performance of your Spring RESTful APIs and provide a better user experience to your application's users.