Writing Robust Test Cases with Parameterized Tests in JUnit 5
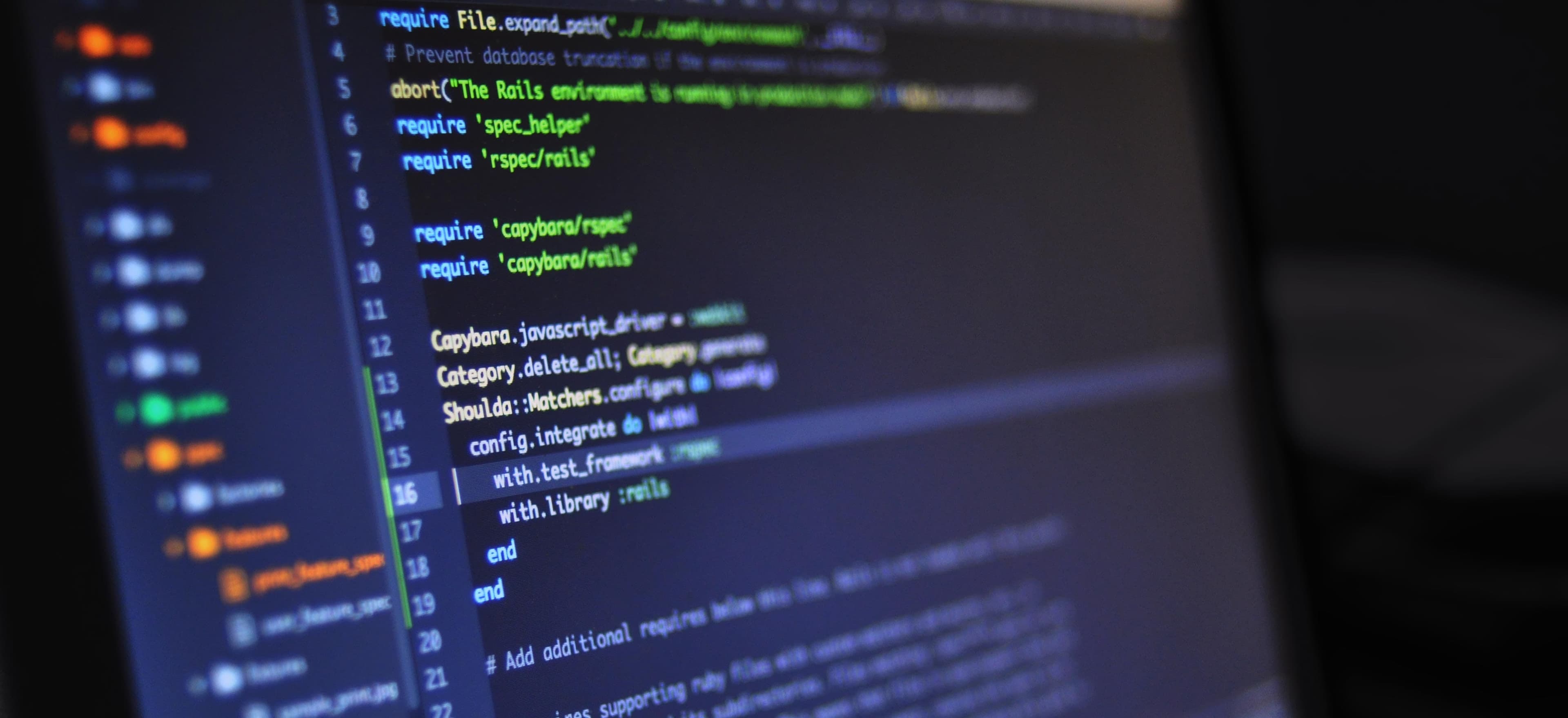
- Published on
Writing Robust Test Cases with Parameterized Tests in JUnit 5
Introduction
Writing robust test cases is essential for ensuring the quality and reliability of your Java applications. One way to improve the quality of your tests is by using parameterized tests. In this article, we will explore how to write parameterized tests in JUnit 5, the latest version of the popular testing framework for Java.
What are Parameterized Tests?
Parameterized tests allow you to run the same test logic with different input values. Instead of writing multiple test methods for different inputs, you can write a single test method that can be executed with a variety of test data.
Parameterized tests are especially useful when you want to test a specific behavior or corner cases of a method with different input values. They can help you identify and handle edge cases that might not be apparent during regular testing.
Getting Started with JUnit 5
Before we dive into parameterized tests, let's start by setting up a project with JUnit 5.
To use JUnit 5 in your Java project, you need to add the following dependencies to your build file:
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
</dependencies>
Once you have added the dependencies, you can start writing and running tests using JUnit 5.
Writing Parameterized Tests in JUnit 5
To create a parameterized test in JUnit 5, you need to follow these steps:
- Declare a test method and annotate it with
@ParameterizedTest
. - Provide a source of parameters for the test method by using the
@MethodSource
or@CsvSource
annotations. - Add parameters to the test method using the
@ValueSource
,@EnumSource
, or@CsvSource
annotations. - Run the test and observe the results.
Let's go through each step in detail.
Step 1: Declare a Test Method
To declare a parameterized test method, annotate it with the @ParameterizedTest
annotation. This annotation tells JUnit 5 that this method should be executed as a parameterized test.
Here's an example:
@ParameterizedTest
public void testCalculateSquare(int number) {
// Test logic goes here
}
Step 2: Provide a Source of Parameters
After declaring the test method, you need to provide a source of parameters for the test method. JUnit 5 provides two main annotations for this purpose: @MethodSource
and @CsvSource
.
Using @MethodSource
The @MethodSource
annotation allows you to specify a method that will provide the test parameters. This method should return a Stream
or an Iterable
of arguments.
Here's an example:
@ParameterizedTest
@MethodSource("provideNumbers")
public void testCalculateSquare(int number) {
// Test logic goes here
}
private static Stream<Integer> provideNumbers() {
return Stream.of(2, 3, 4, 5);
}
In this example, the provideNumbers
method returns a Stream
of integers, which will be used as the input for the test method. The test method will be executed four times, once for each number in the stream.
Using @CsvSource
The @CsvSource
annotation allows you to define the test parameters directly within the annotation itself. You provide a list of comma-separated values, and JUnit 5 will generate the arguments for the test method.
Here's an example:
@ParameterizedTest
@CsvSource({"2", "3", "4", "5"})
public void testCalculateSquare(int number) {
// Test logic goes here
}
In this example, the test method will be executed four times, once for each value specified in the @CsvSource
annotation.
Step 3: Add Parameters to the Test Method
Once you have provided a source of parameters, you need to add parameters to the test method itself. You can do this by annotating the test method with @ValueSource
, @EnumSource
, or @CsvSource
.
Using @ValueSource
The @ValueSource
annotation allows you to provide a single value as a parameter to the test method. You can specify multiple values by passing them as an array to the annotation.
Here's an example:
@ParameterizedTest
@ValueSource(ints = {2, 3, 4, 5})
public void testCalculateSquare(int number) {
// Test logic goes here
}
In this example, the test method will be executed four times, once for each value specified in the @ValueSource
annotation.
Using @EnumSource
The @EnumSource
annotation allows you to specify an enum type as a parameter to the test method. JUnit 5 will automatically generate the enum values as arguments for the test method.
Here's an example:
enum NumberType {
EVEN, ODD
}
@ParameterizedTest
@EnumSource(NumberType.class)
public void testIsEven(NumberType numberType) {
// Test logic goes here
}
In this example, the test method will be executed twice, once for each enum value (EVEN
and ODD
).
Using @CsvSource
As mentioned earlier, you can also use the @CsvSource
annotation to provide parameters directly within the annotation.
Here's an example:
@ParameterizedTest
@CsvSource({"2, true", "3, false"})
public void testIsPrime(int number, boolean expected) {
// Test logic goes here
}
In this example, the test method will be executed twice, once for each row specified in the @CsvSource
annotation.
Step 4: Run the Test and Observe the Results
Once you have written your parameterized test, you can run it with the JUnit 5 test runner. The test runner will execute the test method with each set of parameters and report the results.
You can use your preferred IDE's test runner or run the tests from the command line using build tools like Maven or Gradle.
Conclusion
Writing robust test cases is crucial for ensuring the quality and reliability of your Java applications. Parameterized tests in JUnit 5 allow you to write concise and reusable tests that can be executed with different inputs.
In this article, we explored how to write parameterized tests in JUnit 5, from setting up a project with JUnit 5 to running the tests with different input values. By using parameterized tests, you can improve the thoroughness of your testing and identify edge cases that might not be apparent during regular testing.
So go ahead and start writing robust test cases with parameterized tests in JUnit 5 to enhance the quality of your Java applications!
Checkout our other articles