Identifying the Appropriate Design Pattern: A Key Challenge
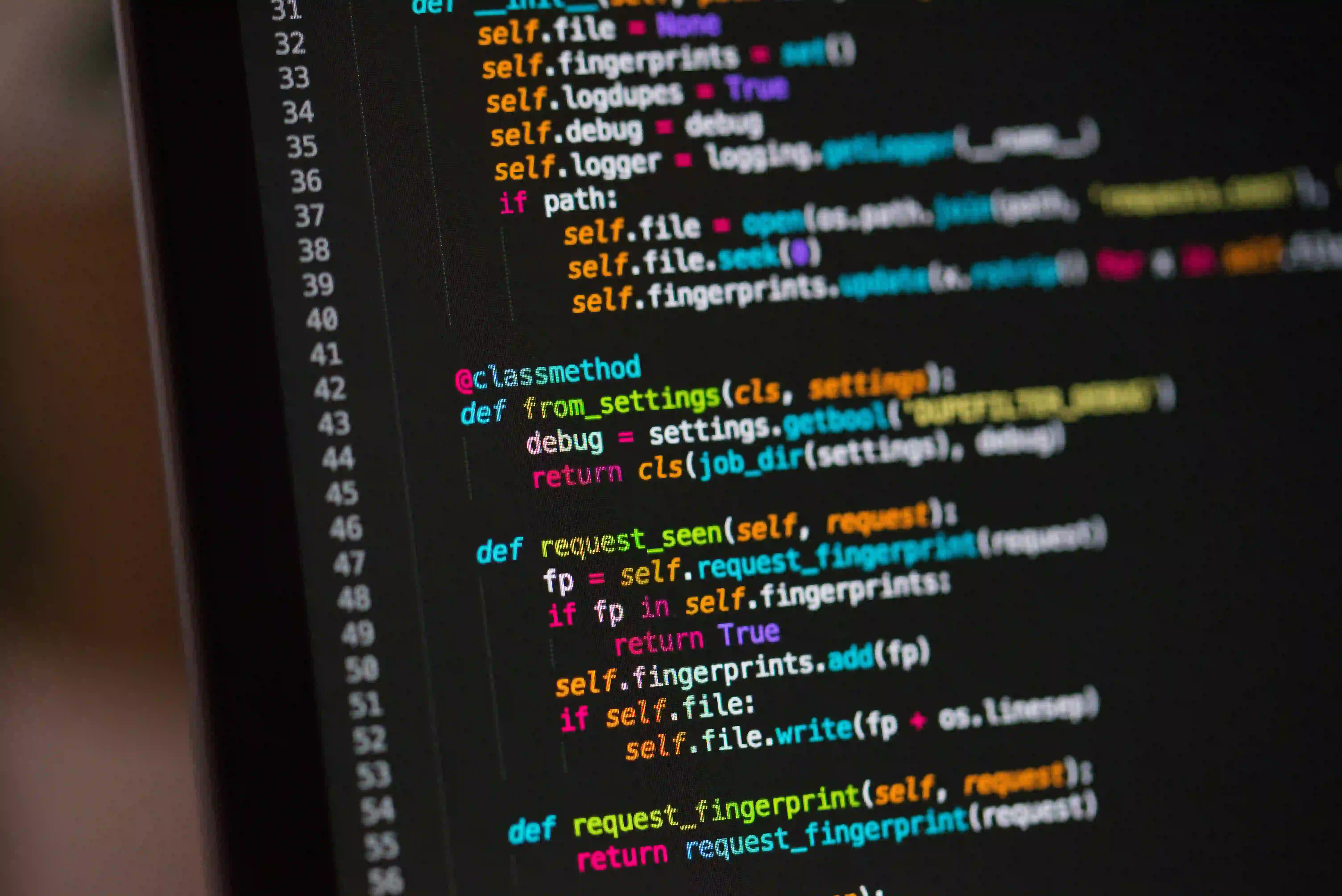
Design patterns play a crucial role in software development. They provide proven solutions to common problems and promote code reuse and maintainability. However, choosing the right design pattern can be challenging. With so many options available, developers often find themselves overwhelmed and unsure of which pattern to apply.
In this article, we will explore the process of identifying the appropriate design pattern for your project. We will discuss the key factors to consider, examine popular design patterns, and provide guidance on making an informed decision.
Understanding the Problem
The first step in identifying the appropriate design pattern is to thoroughly understand the problem you are trying to solve. This involves analyzing the requirements, identifying the key stakeholders, and determining the overall goals of the project. By clearly defining the problem, you can better evaluate which design pattern will best address the specific needs.
Examining Popular Design Patterns
To make an informed decision, it's important to have a solid understanding of the most common design patterns. Here, we will briefly explore some popular design patterns and their typical use cases:
1. Singleton Pattern
The Singleton pattern ensures that only one instance of a class is created and provides a global point of access to it. This pattern is often used for resources that need to be shared across different parts of an application, such as configuration settings or database connections.
public class Singleton {
private static Singleton instance;
private Singleton() { }
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
2. Observer Pattern
The Observer pattern establishes a one-to-many relationship between objects. When one object changes state, all dependent objects are notified automatically. This pattern is commonly used in event-driven systems or when building user interface components.
public interface Observer {
void update();
}
public interface Subject {
void attach(Observer observer);
void detach(Observer observer);
void notifyObservers();
}
public class ConcreteSubject implements Subject {
private List<Observer> observers = new ArrayList<>();
public void attach(Observer observer) {
observers.add(observer);
}
public void detach(Observer observer) {
observers.remove(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update();
}
}
}
3. Factory Method Pattern
The Factory Method pattern provides an interface for creating objects, but leaves the subclasses to decide which class to instantiate. This pattern is useful when you want to decouple the client code from the actual instantiation logic, enabling easier maintenance and future extendability.
public interface Animal {
void makeSound();
}
public class Dog implements Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
public class Cat implements Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
public interface AnimalFactory {
Animal createAnimal();
}
public class DogFactory implements AnimalFactory {
public Animal createAnimal() {
return new Dog();
}
}
public class CatFactory implements AnimalFactory {
public Animal createAnimal() {
return new Cat();
}
}
Evaluating the Factors
After understanding the problem and exploring potential design patterns, it's crucial to evaluate various factors to determine the most appropriate pattern.
1. Flexibility and Future Changes
Consider how the design pattern will accommodate potential future changes in requirements. A pattern that allows for easy modification and extension is often more suitable.
2. Complexity and Learning Curve
Evaluate the complexity of the design pattern and whether it aligns with the skill set and expertise of the development team. If the team is unfamiliar with a particular pattern, the learning curve might impact productivity and overall project timeline.
3. Performance Trade-offs
Different design patterns have varying performance implications. Consider the specific performance requirements of your application and select a pattern that aligns with those needs.
4. Code Reusability and Maintainability
Examine the potential for code reuse and the ease of maintenance. A pattern that promotes code reusability and simplifies maintenance tasks is often a good choice.
Making the Decision
By thoroughly evaluating the problem, examining popular design patterns, and considering the above factors, you are equipped to make an informed decision. Remember that there is usually no one-size-fits-all solution, and the appropriateness of a design pattern may vary depending on the specific project requirements.
Conclusion
Choosing the appropriate design pattern is a crucial step in software development. It not only ensures the efficient and effective implementation of the solution but also helps maintain code quality, reusability, and scalability. By understanding the problem, exploring popular patterns, and evaluating various factors, you can confidently select the best design pattern that aligns with your project requirements.