How to seamlessly integrate MongoDB with your Spring project
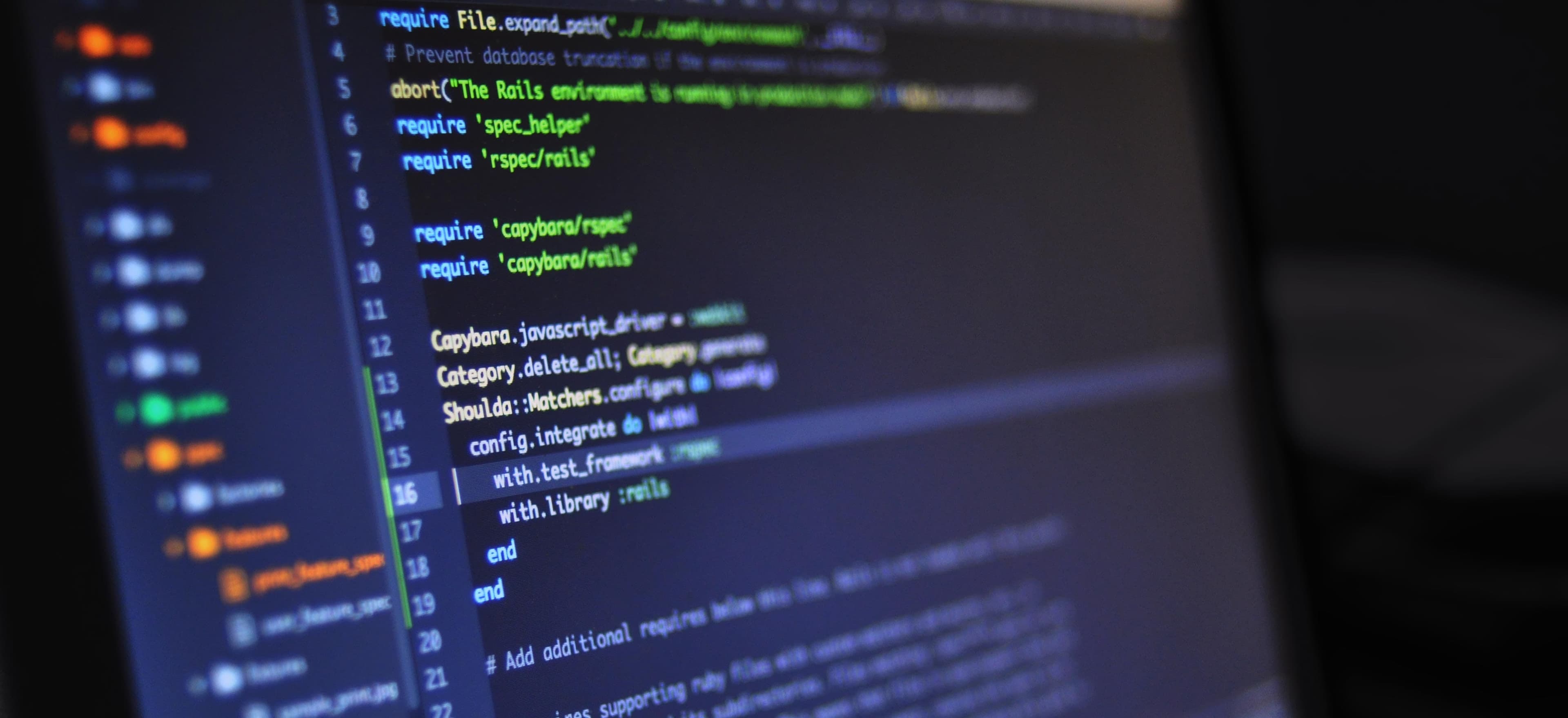
- Published on
How to Seamlessly Integrate MongoDB with Your Spring Project
In today's fast-paced and data-driven world, the need for scalable and flexible databases is more critical than ever. MongoDB, a NoSQL database, provides a flexible schema design and distributed architecture that allows developers to build modern applications with ease. When combined with the power of Spring, a popular Java framework, you can seamlessly integrate MongoDB into your Spring project and take advantage of its powerful features.
In this article, we will explore how to integrate MongoDB with your Spring project step by step. We will cover the following topics:
- Setting up a MongoDB Database
- Configuring Spring Data MongoDB
- Defining MongoDB Entities
- Performing CRUD Operations
- Querying Data with MongoDB Spring Repositories
- Implementing Advanced Queries
- Working with Aggregations
- Handling Transactions
Setting Up a MongoDB Database
Before we can integrate MongoDB with our Spring project, we need to set up a MongoDB database. MongoDB can either be installed on your local machine or hosted on a cloud service provider such as MongoDB Atlas.
To install MongoDB on your local machine, you can follow the instructions provided in the official MongoDB documentation: MongoDB Installation Guide
Alternatively, you can create a free MongoDB Atlas account and set up a cloud-hosted MongoDB cluster: MongoDB Atlas
Configuring Spring Data MongoDB
Once we have our MongoDB database up and running, we can start configuring Spring Data MongoDB in our Spring project.
First, we need to include the necessary dependencies in our project's pom.xml
file (if you are using Maven) or build.gradle
file (if you are using Gradle). Here are the dependencies:
<dependencies>
<!-- Other dependencies... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
</dependencies>
Next, we need to configure the MongoDB connection properties in our application.properties
or application.yml
file. Here is an example configuration:
spring.data.mongodb.uri=mongodb://localhost:27017/mydatabase
spring:
data:
mongodb:
uri: mongodb://localhost:27017/mydatabase
Replace mydatabase
with the name of your database.
With these configurations in place, Spring will automatically set up a connection to our MongoDB database.
Defining MongoDB Entities
In order to work with MongoDB using Spring Data MongoDB, we need to define our MongoDB entities. MongoDB entities are similar to traditional JPA entities but have some key differences.
First, MongoDB entities should be annotated with @Document
to indicate that they are MongoDB documents. Additionally, we need to specify the MongoDB collection name using the collection
attribute of the @Document
annotation.
Here's an example of a MongoDB entity representing a Person
:
@Document(collection = "persons")
public class Person {
@Id
private String id;
private String firstName;
private String lastName;
// Getters and setters...
}
In this example, the Person
entity has an id
field annotated with @Id
, which is used to uniquely identify each document. It also has firstName
and lastName
fields, which will be stored as properties within the document.
Performing CRUD Operations
With our MongoDB entities defined, we can now perform CRUD (Create, Read, Update, Delete) operations on our MongoDB database using Spring Data MongoDB.
To perform CRUD operations, we can use the MongoRepository
interface provided by Spring Data MongoDB. This interface provides methods for common database operations such as saving, finding, updating, and deleting entities.
Here's an example of a PersonRepository
interface that extends MongoRepository
:
public interface PersonRepository extends MongoRepository<Person, String> {
// Additional custom query methods...
}
By extending MongoRepository
, we inherit all the basic CRUD methods automatically. For example, we can save a Person
entity using the save()
method:
Person person = new Person();
person.setFirstName("John");
person.setLastName("Doe");
personRepository.save(person);
Similarly, we can find a Person
entity using the findById()
method:
Optional<Person> optionalPerson = personRepository.findById("12345");
optionalPerson.ifPresent(person -> {
// Perform operations on the person object...
});
Querying Data with MongoDB Spring Repositories
In addition to the basic CRUD operations, Spring Data MongoDB also provides support for querying data using its repository abstraction.
By following Spring Data's method naming conventions and using query methods, we can easily define custom queries for our MongoDB entities without writing any actual query code.
For example, let's say we want to find all persons with a specific last name. We can define a method in our PersonRepository
interface like this:
public interface PersonRepository extends MongoRepository<Person, String> {
List<Person> findByLastName(String lastName);
}
Spring Data will automatically generate the corresponding MongoDB query based on the method name, saving us from writing the query ourselves. We can then use this method to find persons with a specific last name:
List<Person> persons = personRepository.findByLastName("Doe");
Implementing Advanced Queries
While Spring Data MongoDB's query methods are convenient for simple queries, sometimes we need to perform more complex queries or have specific requirements. In such cases, we can use the @Query
annotation to define custom queries using MongoDB Query Language.
For example, let's say we want to find all persons with a specific first name and sort them by their last name. We can define a custom query method in our PersonRepository
interface using the @Query
annotation:
public interface PersonRepository extends MongoRepository<Person, String> {
@Query("{ 'firstName' : ?0 }")
List<Person> findByFirstNameAndSortByLastName(String firstName, Sort sort);
}
In this example, the @Query
annotation takes a MongoDB Query Language expression as its value. We can use the ?0
placeholder to dynamically pass in the first name parameter.
We can now use this custom query method to find persons with a specific first name and sort them by their last name:
List<Person> persons = personRepository.findByFirstNameAndSortByLastName("John", Sort.by(Sort.Order.asc("lastName")));
Working with Aggregations
MongoDB provides powerful aggregation frameworks that allow us to perform complex data manipulations and transformations. With Spring Data MongoDB, we can easily leverage these aggregation frameworks to perform aggregations on our MongoDB data.
To use aggregations in Spring Data MongoDB, we can create a custom repository method and use the Aggregation
class provided by Spring Data MongoDB to define our aggregation pipeline.
For example, let's say we want to calculate the average age of all persons in our database. We can define a custom repository method like this:
public interface PersonRepository extends MongoRepository<Person, String> {
@Aggregation("{ $group: { _id: null, averageAge: { $avg: '$age' } } }")
AverageAgeResult calculateAverageAge();
}
In this example, we define an aggregation pipeline using the MongoDB aggregation syntax within the @Aggregation
annotation. The result of the aggregation is mapped to a AverageAgeResult
class, which we need to define.
We can then use this custom repository method to calculate the average age:
AverageAgeResult result = personRepository.calculateAverageAge();
double averageAge = result.getAverageAge();
Handling Transactions
With the release of MongoDB 4.0, MongoDB has introduced support for multi-document ACID transactions. Spring Data MongoDB provides seamless integration with MongoDB transactions, allowing us to perform atomic operations on our MongoDB data.
To work with transactions in Spring Data MongoDB, we need to configure our MongoDB connection to enable transaction support. We can do this by adding the @EnableMongoTransaction
annotation to our Spring configuration class.
Here's an example of enabling transactions in a Spring Boot application:
@SpringBootApplication
@EnableMongoTransaction
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Once transactions are enabled, we can annotate our service methods with @Transactional
to indicate that they should be executed within a transactional context. Spring will handle all the transaction management behind the scenes.
For example, let's say we want to transfer money between two accounts atomically. We can define a service method like this:
@Transactional
public void transferMoney(String fromAccountId, String toAccountId, BigDecimal amount) {
// Perform atomic money transfer here...
}
With this setup, Spring will automatically manage the transaction boundary, ensuring that the transfer operation is atomic.
Conclusion
Integrating MongoDB with your Spring project can provide you with a scalable and flexible database solution. With Spring Data MongoDB, you can seamlessly work with MongoDB using a simple and intuitive API.
In this article, we explored how to set up a MongoDB database, configure Spring Data MongoDB, define MongoDB entities, perform CRUD operations, query data using MongoDB Spring repositories, implement advanced queries, work with aggregations, and handle transactions.
By following these steps, you will be able to seamlessly integrate MongoDB with your Spring project and take advantage of its powerful capabilities.
To learn more about MongoDB and Spring Data MongoDB, check out the following resources:
- Spring Data MongoDB Reference Documentation
- MongoDB Java Driver Documentation
- MongoDB University offers free online courses on MongoDB and Spring Data MongoDB.
- Official MongoDB Documentation provides comprehensive guides, tutorials, and examples on MongoDB and Spring Data MongoDB.
Happy coding!
Checkout our other articles