Handling Expected Exceptions in JUnit Tests: Best Practices
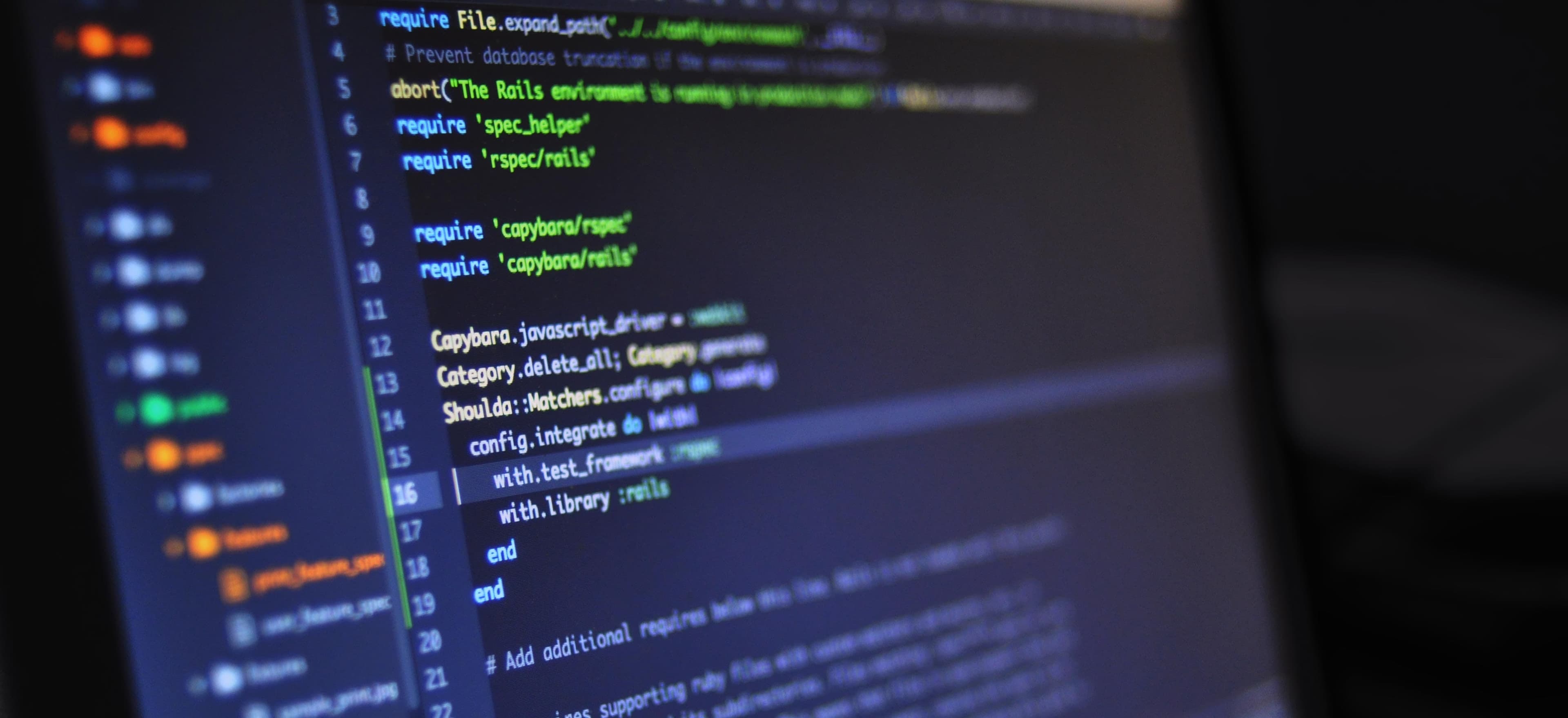
- Published on
In the world of software development, testing is an essential part of the process. It helps ensure the quality and reliability of the code we write. When it comes to testing in Java, JUnit is the go-to framework for many developers. It provides a robust and flexible way to write unit tests.
One common scenario in testing is handling expected exceptions. Sometimes, we expect a certain piece of code to throw an exception under specific circumstances, and we want to assert that the exception is indeed thrown. In this blog post, we will explore some best practices for handling expected exceptions in JUnit tests.
- Use the
@Test
annotation
In JUnit, we use the @Test
annotation to mark a method as a test case. By default, JUnit expects the test method to complete successfully without throwing any exceptions. However, we can use the expected
attribute of the @Test
annotation to indicate that the test is expected to throw a specific exception. For example:
@Test(expected = NullPointerException.class)
public void testNullPointerException() {
String str = null;
str.length();
}
In the above example, we are expecting a NullPointerException
to be thrown when calling the length()
method on a null
string. If the exception is not thrown, the test will fail.
- Use the
assertThrows
method
Starting from JUnit 4.13, a new utility method called assertThrows
has been introduced. This method provides a more flexible and expressive way to handle expected exceptions. Instead of using the expected
attribute of the @Test
annotation, we can use assertThrows
within the test method itself. For example:
@Test
public void testNullPointerException() {
String str = null;
assertThrows(NullPointerException.class, () -> str.length());
}
In this example, the assertThrows
method is used to assert that a NullPointerException
is thrown when calling the length()
method on a null
string. If the exception is not thrown, the test will fail.
- Use the
expectException
method (only for JUnit 4)
If you are using an older version of JUnit (before 4.13) and cannot upgrade to the latest version, you can use the expectException
method from the ExpectedException
rule. This method allows you to specify the expected exception. For example:
@Rule
public ExpectedException exceptionRule = ExpectedException.none();
@Test
public void testNullPointerException() {
exceptionRule.expect(NullPointerException.class);
String str = null;
str.length();
}
In this example, the expectException
method is used to assert that a NullPointerException
is thrown when calling the length()
method on a null
string. If the exception is not thrown, the test will fail.
- Provide a descriptive error message
When writing test cases, it is important to provide descriptive error messages to aid debugging in case of test failures. This is especially important when handling expected exceptions. The error message should clearly indicate that the exception was expected and provide any relevant information for understanding the test failure. For example:
@Test
public void testNullPointerException() {
String str = null;
try {
str.length();
fail("Expected NullPointerException, but no exception was thrown.");
} catch (NullPointerException e) {
assertEquals("Cannot call length() on a null object.", e.getMessage());
}
}
In this example, we use the fail
method to indicate that we were expecting a NullPointerException
, but no exception was thrown. This will produce a failure message that clearly indicates the expected exception was not thrown.
- Test for specific exception messages
Sometimes, we want to ensure that a specific exception message is thrown along with the expected exception. This can be useful when the exception message contains important information or error codes. To test for a specific exception message, we can use the getMessage()
method of the caught exception and assert against the expected message. For example:
@Test
public void testIllegalArgumentException() {
int[] array = {1, 2, 3};
try {
int value = array[4];
fail("Expected ArrayIndexOutOfBoundsException, but no exception was thrown.");
} catch (ArrayIndexOutOfBoundsException e) {
assertEquals("Index 4 out of bounds for length 3", e.getMessage());
}
}
In this example, we are expecting an ArrayIndexOutOfBoundsException
to be thrown when accessing an array element outside its bounds. We assert that the exception message matches the expected message.
By following these best practices, you can ensure that your JUnit tests effectively handle expected exceptions. This will make your tests more robust, reliable, and easier to maintain. It's important to note that handling expected exceptions is just one aspect of testing, and there are many other best practices to consider. For more information on JUnit and testing in Java, check out the following resources:
- JUnit 5 User Guide
- Testing in Java
- Effective Unit Testing
- JUnit Best Practices
Checkout our other articles