5 Tips for Simplifying Complex Event Processing
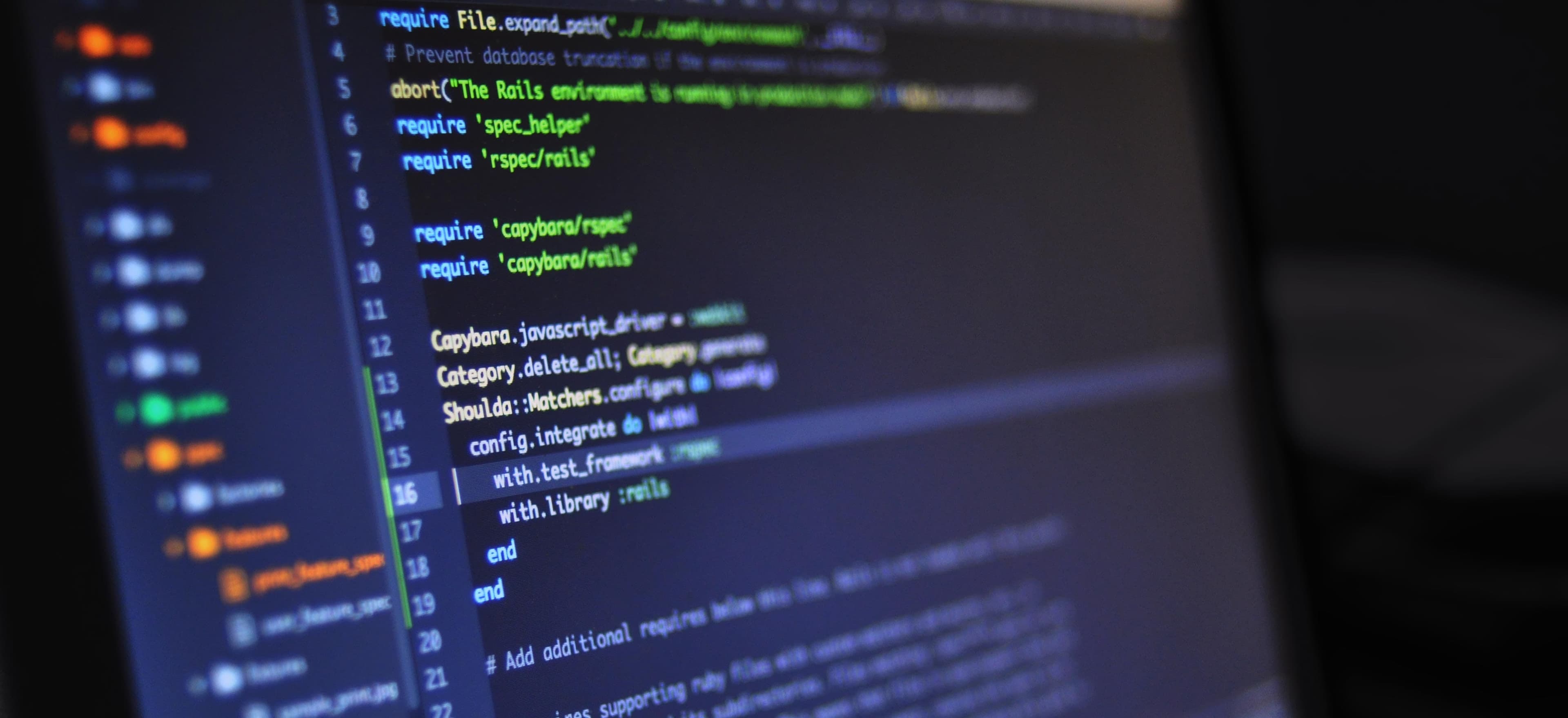
- Published on
Complex Event Processing (CEP) is a powerful technology that allows organizations to analyze and process large volumes of data in real-time. It enables them to identify meaningful patterns and correlations in the data stream, which can then be used for a variety of purposes, such as fraud detection, risk assessment, and real-time decision-making.
However, working with CEP can be challenging, especially when dealing with large and complex data sets. In this article, we will discuss five tips for simplifying complex event processing, making it easier to work with and extract valuable insights from the data.
- Clearly Define Event Types and Event Schemas: When dealing with complex data and event streams, it is essential to have a clear understanding of the types of events being processed and their underlying structure. By clearly defining event types and event schemas, you can ensure that the data being processed is accurate and consistent, which is crucial for accurate analysis and decision-making.
One way to achieve this is by using Java classes to represent event types and define their schema. By using classes, you can enforce data consistency, add relevant metadata, and easily validate the data against the defined schema. This approach also makes it easier to work with the data in a typed manner, improving code readability and maintainability.
Here's an example of how you can define an event schema using a Java class:
public class OrderEvent {
private int orderId;
private String customerId;
private double amount;
private LocalDateTime timestamp;
// Constructors, getters, and setters go here...
}
- Use a Stream Processing Framework: A stream processing framework provides a high-level abstraction for working with event streams, making it easier to process and analyze data. These frameworks typically provide built-in abstractions for handling time-based computations, windowing, joining, and aggregations, which are commonly used in complex event processing.
There are several stream processing frameworks available for Java, such as Apache Kafka Streams, Apache Flink, and Apache Samza. These frameworks offer a wide range of features and integrations, allowing you to build scalable and fault-tolerant CEP applications.
Using a stream processing framework can simplify complex event processing by abstracting away low-level details and providing a more intuitive and declarative programming model. It also enables you to focus on the business logic of your application, rather than dealing with the intricacies of event processing.
- Embrace Event-Driven Architecture: Event-driven architecture (EDA) is an architectural pattern that promotes the production, detection, consumption, and reaction to events. It is a natural fit for complex event processing applications, as it allows you to decouple the components of your system and enable asynchronous communication between them.
By embracing EDA, you can simplify complex event processing by breaking down your application into smaller, modular components that are responsible for specific tasks. Each component can independently process events and emit new events, allowing for scalability and flexibility.
In an event-driven architecture, you can use message brokers such as Apache Kafka or RabbitMQ to handle the streaming of events between components. This approach decouples the producer and consumer of events and provides fault-tolerance and durability, making it easier to build robust and scalable CEP applications.
- Utilize Pattern Matching and Rule Engines: Pattern matching and rule engines are powerful tools for simplifying complex event processing. They allow you to define rules or patterns that describe specific conditions or behavior in the event stream, and then automatically detect and react to these patterns.
There are several Java libraries available for pattern matching and rule-based processing, such as Drools and Esper. These libraries provide a declarative and expressive language for defining rules and patterns, making it easier to express complex conditions and behaviors in a concise and readable manner.
By utilizing pattern matching and rule engines, you can simplify the logic of your CEP application by externalizing complex rules and conditions. This approach makes your code more maintainable and flexible, as you can easily modify or add new rules without changing the core logic of your application.
- Optimize Performance and Scalability: Complex event processing often involves processing large volumes of data in real-time, which can be challenging from a performance and scalability perspective. To simplify CEP and ensure efficient processing, it is essential to optimize your application for performance and scalability.
One way to optimize performance is by leveraging parallel processing and distributed computing techniques. Stream processing frameworks like Apache Flink and Apache Samza provide built-in support for parallel processing and distributed execution, allowing you to scale your CEP application horizontally across multiple machines or clusters.
Additionally, you can optimize performance by carefully designing your event schemas and data structures. Using appropriate data structures, indexing techniques, and caching mechanisms can significantly improve the efficiency of data processing and reduce latency.
Furthermore, monitoring and profiling your CEP application can help identify performance bottlenecks and optimize resource utilization. Tools like Java Flight Recorder and Java Mission Control can provide valuable insights into the runtime behavior and performance characteristics of your application.
In conclusion, by following these five tips, you can simplify complex event processing and make it more manageable and efficient. Clearly defining event types and schemas, using a stream processing framework, embracing event-driven architecture, utilizing pattern matching and rule engines, and optimizing performance and scalability are essential strategies for successful complex event processing. By applying these techniques, you can extract valuable insights from your data streams and make real-time decisions that drive business success.
Sources:
- Apache Kafka Streams
- Apache Flink
- Apache Samza
- Drools
- Esper
- Java Flight Recorder and Java Mission Control
Checkout our other articles