Groovy Magic: Simplify Scripting in Your Next Project!
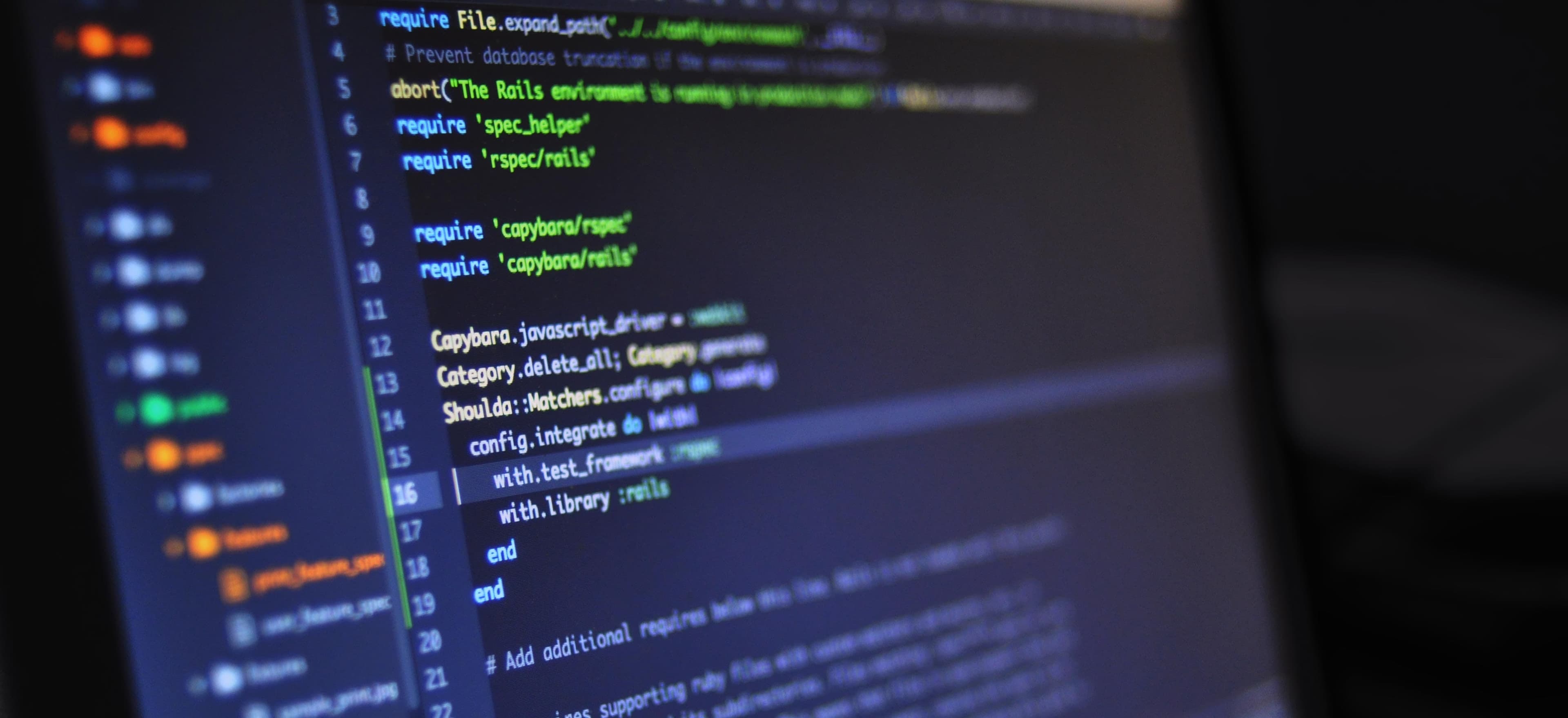
- Published on
Groovy Magic: Simplify Scripting in Your Next Project!
When working on complex Java projects, it might often feel like you're getting bogged down with boilerplate code and lengthy configuration files. Wouldn't it be great if there was a way to handle some of this more efficiently, perhaps with a scripting touch? Enter Groovy - a language that offers the dynamism of a scripting language with the power of the Java platform. In this blog post, we'll explore the magic of Groovy and how it can simplify scripting in your next project.
The Groovy Language Basics
Groovy is an object-oriented language for the JVM that offers a level of simplicity and flexibility you don't always find in Java. It's fully integrated with Java, and its syntax is incredibly similar, which means that Java developers can usually pick it up very quickly.
The real power of Groovy comes from its ability to cut down on Java's verbosity, offering features such as closures, builders, and runtime metaprogramming, which can make scripts much briefer and more expressive. Let's take a look at some basic Groovy features that demonstrate its elegance.
The Basics with Examples
Consider the traditional Hello World in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
And now, the Groovy version:
println "Hello, World!"
Notice the lack of a class or method definition in Groovy? This simple example already shows the reduced boilerplate code. You can execute Groovy scripts, which are compiled to Java bytecode and run on the JVM just as efficiently as Java programs.
Groovy’s Closures
Closures are a first-class citizen in Groovy. They can be passed around like objects and have their own methods and variables. Consider a list iteration in Java:
List<String> items = Arrays.asList("Apple", "Banana", "Cherry");
for (String item : items) {
System.out.println(item);
}
And in Groovy:
def items = ["Apple", "Banana", "Cherry"]
items.each { println it }
Groovy introduces the it
variable that represents the current item in the context of the closure, simplifying the iteration process.
Operator Overloading
Groovy also supports operator overloading, which can make arithmetic manipulations read more naturally.
println 10 + 5 // Addition
println 10 - 5 // Subtraction
println [1, 2] + [3, 4] // Concatenating lists
Accessing and Modifying Members
In Groovy, you often don't need getters and setters as you would in Java; properties can be accessed directly.
class Fruit {
String name
}
def apple = new Fruit()
apple.name = 'Apple' // Sets the name property
println apple.name // Gets the name property
Integrating Groovy Into a Java Project
Integrating Groovy with Java is straightforward, thanks to the Groovy-Eclipse compiler plugin, which allows for joint compilation of both Java and Groovy code. This means you can use Groovy to write tests for your Java code or even script parts of your application logic where brevity and flexibility are more important than static typing.
Joint Compilation
Ensure that your project is set up to handle both Java and Groovy. You can do this by adding the necessary plugins and dependencies to your Maven or Gradle build files.
Here's an example of using Groovy with Maven:
<dependencies>
<!-- Include Groovy -->
<dependency>
<groupId>org.codehaus.groovy</groupId>
<artifactId>groovy-all</artifactId>
<version>3.0.7</version>
</dependency>
</dependencies>
<plugins>
<!-- Add support for Groovy -->
<plugin>
<artifactId>groovy-eclipse-compiler</artifactId>
<groupId>org.codehaus.groovy</groupId>
<version>3.0.7-01</version>
<extensions>true</extensions>
</plugin>
</plugins>
Pragmatic Example: Using Groovy for Configuration
XML and JSON are often used for configuration in Java projects, but these can be verbose and cumbersome. Groovy provides a much more concise way to handle such configurations using its builders.
Here's a XML configuration in Java:
// Typical XML configuration strings or file manipulation
String xmlConfig = "<config><name>MyApp</name><version>1.0</version></config>";
Now, Groovy offers a DSL-like approach to handle this:
def config = new MarkupBuilder().bind {
config {
name 'MyApp'
version '1.0'
}
}
println config.toString()
Groovy's MarkupBuilder
allows for creating XML structures programmatically using a more natural syntax.
The Ecosystem of Groovy
Groovy is not just a language, it's an ecosystem. There are frameworks like Grails, which is to Groovy what Spring Boot is to Java, allowing you to create web applications rapidly. Tools like Gradle, which have taken the automation world by storm, are Groovy-based DSLs for describing build and deployment scripts.
With Spock, Groovy further simplifies testing by providing an expressive and powerful syntax for writing tests, which can target both Java and Groovy code.
Best Practices for Adopting Groovy
When integrating Groovy into a Java project, it's important to recognize the differences between the languages and take advantage of each where appropriate.
- Start Small: Use Groovy for small scripting tasks or for writing tests.
- Use the Right Tool: Choose Groovy for tasks that require more flexibility and dynamic scripting.
- Maintainability: Ensure that code is kept understandable to developers who might not be familiar with Groovy.
- Performance Considerations: Although Groovy is fast, Java is usually faster for strictly typed applications. Use Groovy wisely to avoid a performance hit.
Conclusion
Groovy's magic lies in its seamless integration with Java and its ability to simplify scripting tasks within a Java project. It empowers developers to write concise and readable code, freeing them from the Java boilerplate straitjacket while still leveraging the Java ecosystem's robustness.
If you'd like to give Groovy a try in your next project, you'll find an abundance of resources and a supportive community to help you on your journey. The official Groovy website is a great place to start, offering documentation, tutorials, and more.
Remember, the goal of using Groovy isn't to replace Java, but to complement it, making your life as a developer a little bit easier—and perhaps even a little more magical.
Checkout our other articles