Optimizing Java JVM for High-Performance Media Processing
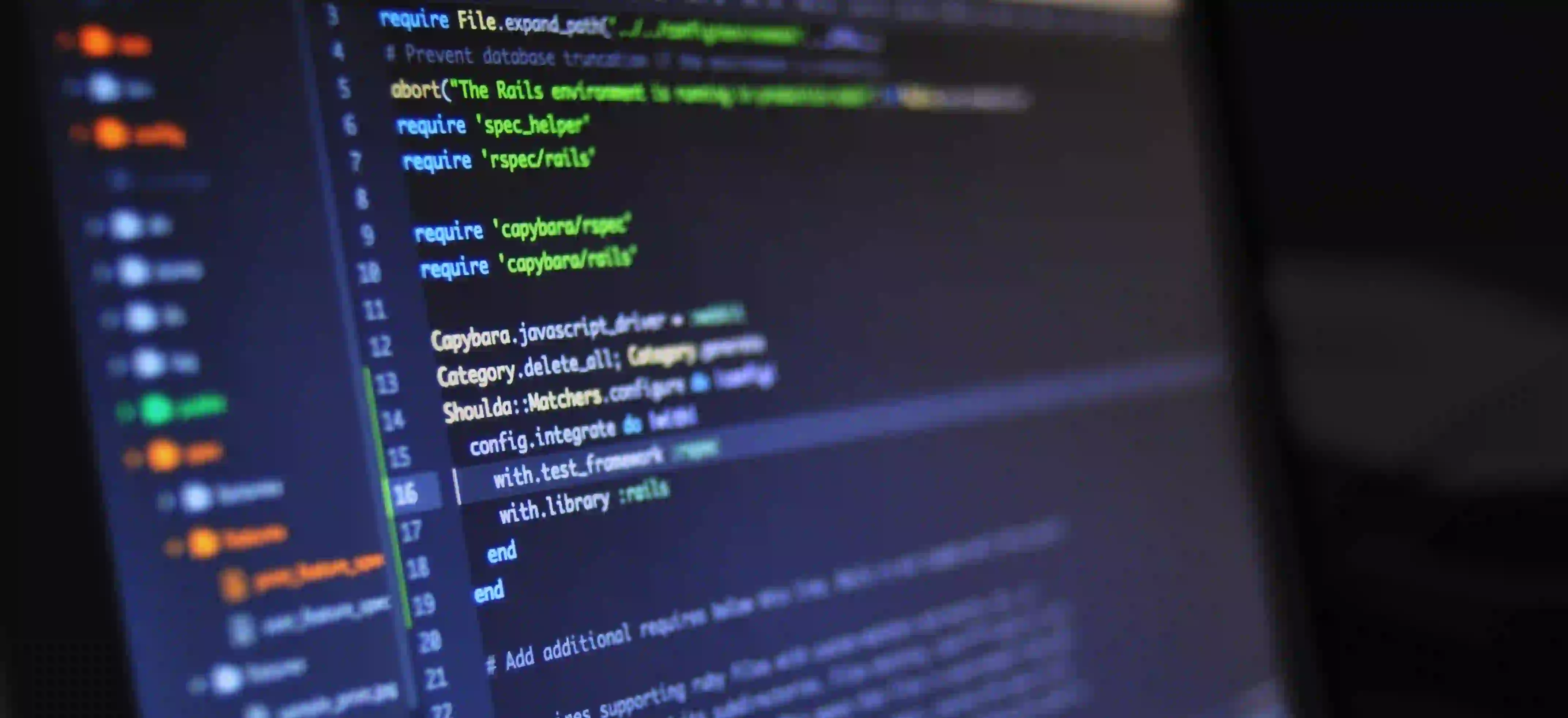
Optimizing Java JVM for High-Performance Media Processing
Java has long been a staple in large-scale enterprise environments, but with the advent of high-performing media processing demands, the spotlight has turned on Java's ability to keep up. In this digital era, where media processing is integral to various industries, optimizing the Java Virtual Machine (JVM) is paramount for efficiency and speed. Today, we're delving into the nitty-gritty of fine-tuning the JVM to handle high-performance media processing tasks.
Understanding JVM Configuration for Media Processing
Media processing applications typically require heavy I/O operations, significant memory management, and real-time data processing capabilities. The JVM, the engine powering Java applications, must therefore be configured to manage these resource-intensive processes effectively.
JVM Memory Management
One of the most critical aspects of JVM optimization is memory management. Media processing applications often require large amounts of memory, and efficient memory utilization can dramatically impact performance.
Here are the key memory areas in the JVM:
-
Heap Memory:
- Managed by the garbage collector (GC).
- Stores Java objects and is subdivided into Young Generation, Old Generation, and (optionally) the Metaspace.
-
Non-Heap Memory:
- Stores class metadata, JIT compiler code, and other artifacts.
- Metaspace/PermGen (depending on the JVM version).
-
Stack Memory:
- Stores local variables and function call stacks.
- Each thread in Java has its own stack.
Code Example: Setting the Heap Size
// This is a command-line argument and not part of the Java code.
// Set the initial and maximum heap size to 512MB and 2GB respectively.
java -Xms512m -Xmx2g MyMediaProcessingApp
Why this matters: Allocating sufficient initial heap memory (-Xms
) helps reduce the overhead caused by the JVM frequently resizing the heap. Setting an appropriate maximum heap size (-Xmx
) helps prevent out-of-memory errors during heavy processing tasks.
Garbage Collection Tuning
Garbage collection is a double-edged sword: essential for memory management but potentially disruptive when processing media in real-time. Selecting the right garbage collector and tuning its parameters can help mitigate pauses and maintain performance.
Code Example: Selecting a Garbage Collector
// This is a command-line argument and not part of the Java code.
// Use the G1 Garbage Collector.
java -XX:+UseG1GC MyMediaProcessingApp
Why this matters: The G1 (Garbage-First) GC is designed for applications with large heaps and minimizes pause times. However, other collectors like ZGC or Shenandoah may be more suitable for your specific use case.
Thread Optimization
Since media processing is often CPU-bound, creating an optimal threading strategy is vital. Java provides several concurrency frameworks to help, such as the java.util.concurrent
package, which offers a more sophisticated set of synchronization primitives than traditional synchronized
methods and blocks.
Thread Pooling
Utilizing thread pools can significantly improve performance by reusing existing threads rather than creating new ones for every task, thus reducing overhead.
Code Example: Using ExecutorService for Thread Pooling
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class MediaProcessingThreadPool {
private static final int THREAD_POOL_SIZE = 4; // Number assumes a quad-core CPU
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
for (int i = 0; i < 10; i++) {
executorService.submit(() -> processMedia());
}
// Shutdown the pool
executorService.shutdown();
}
private static void processMedia() {
// Media processing logic here
}
}
Why this matters: The ExecutorService
abstracts away the details of task execution, allowing us to focus on the media processing logic. By using a fixed thread pool, we can limit the number of concurrent threads, which is tuned here for a quad-core CPU.
I/O Strategies
Media processing often involves heavy input/output operations. By optimizing the way Java handles I/O, we can reduce bottlenecks and improve throughput.
Java NIO
The New I/O (NIO) package offers non-blocking I/O operations that can greatly improve media processing applications, especially those that require handling multiple simultaneous media streams.
Code Example: Using NIO for Scalable I/O Operations
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
public class MediaFileProcessor {
public static void main(String[] args) {
Path mediaFilePath = Path.of("mediafile.dat");
// Open a file channel
try (FileChannel fileChannel = FileChannel.open(mediaFilePath, StandardOpenOption.READ, StandardOpenOption.WRITE)) {
ByteBuffer buffer = ByteBuffer.allocateDirect(1024); // Use a direct buffer for performance
while (fileChannel.read(buffer) > 0) {
buffer.flip();
// Process the buffer
buffer.clear();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why this matters: FileChannel
with direct buffers (ByteBuffer.allocateDirect
) offers faster I/O by allowing the JVM to use native I/O operations directly. This eliminates the overhead of copying data between user and kernel space, providing a performance boost for heavy I/O processing.
Just-In-Time (JIT) Compiler Optimizations
The JVM's JIT compiler improves performance by compiling bytecode into native machine code at runtime. By leveraging profiling data, you can guide the JIT compiler to optimize critical parts of your application more effectively.
Profiling Guided Optimization
Oracle's JVM provides the ability to use profiling data to guide optimization through the -XX:+TieredCompilation
and -XX:+UseOnStackReplacement
flags, among others.
// This is a command-line argument and not part of the Java code.
// Enable Tiered Compilation
java -XX:+TieredCompilation MyMediaProcessingApp
Why this matters: Tiered compilation permits the JVM to optimize frequently called methods more aggressively, resulting in faster execution of critical sections of your media processing code.
Final Thoughts
Optimizing the Java JVM for high-performance media processing involves a holistic approach, from memory management and garbage collection to threading strategies and JIT compiler tweaks. It's crucial to understand the intricacies of your specific application and use case to tailor JVM options effectively.
Remember, while optimizing, it's easy to get lost in the myriad of options and metrics. Always benchmark and profile your application with realistic workloads to discern actual performance gains. Tools like JMH (Java Microbenchmark Harness) and profilers like VisualVM can be instrumental.
In summary, effective JVM tuning is part art, part science. By arming yourself with the knowledge and tools presented here, you're on the right path to getting the most out of Java for your high-performance media processing needs. Keep experimenting, measuring, and refining—performance optimization is an ongoing quest.