Unlocking Fluent APIs: Taming Lambdas for Stability!
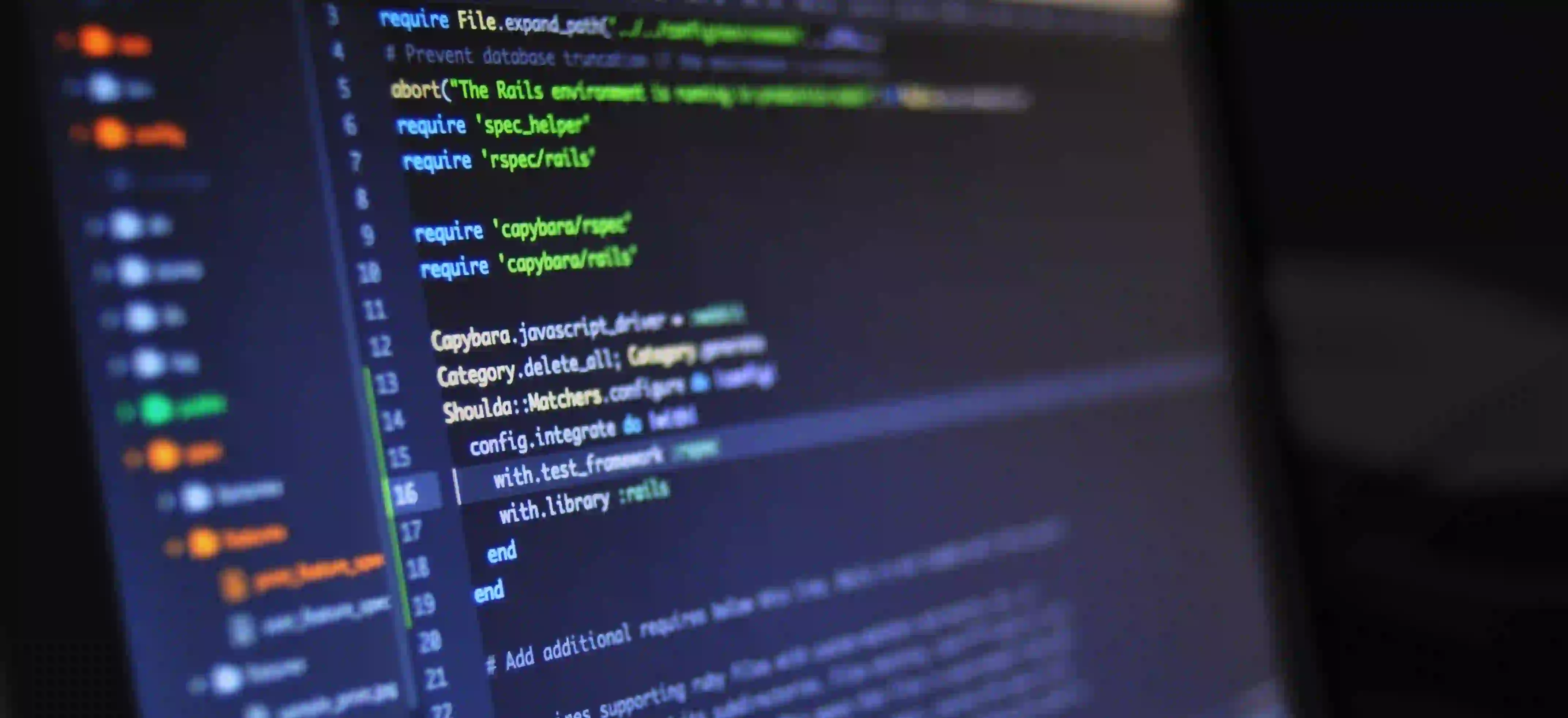
Unlocking Fluent APIs: Taming Lambdas for Stability in Java
Are you tired of clunky and inconsistent APIs that leave you scratching your head? Imagine writing code as eloquent as a Shakespearian sonnet, with method calls flowing seamlessly one after the other, crafting a story that's both logical and beautiful. That's the dream that Fluent APIs promise – and it's a dream you can turn into reality with the proper understanding of Java Lambdas. This post will demonstrate how Lambdas can be combined with Fluent APIs to produce stable, readable, and maintainable code.
What Are Fluent APIs?
Fluent APIs are a pattern of API design that aims to provide more readable code. By returning the object itself or another appropriate object in each method, they allow for method chaining, enabling you to write code that flows like a sentence.
myObject.doThis()
.thenDoThat()
.andThenDoSomethingElse();
In this code snippet, every method call is part of the same chain, which can be read almost like instructions.
The Power of Lambdas in Fluent APIs
With the introduction of Lambdas in Java 8, we brought more than just a new syntax for anonymous functions; we ushered in a potentially transformative pattern for API design. They allow for more concise and flexible code, enabling the implementation of Fluent APIs with even greater capability, such as Java's Stream API.
Let's craft a Fluent API that leverages Lambdas to configure a hypothetical EventProcessor
object:
EventProcessor processor = new EventProcessor()
.on("click", e -> handleEvent(e))
.on("hover", e -> logger.log(e))
.process();
Here, each .on()
method is part of a Fluent API. It takes an event name and a Lambda expression that defines the action to perform when that event occurs.
Stability in Fluent API Design
While Fluent APIs can clean up your code significantly, stability is a concern. A poorly designed Fluent API can lead to confusing, hard-to-change code bases. Here's how you can tame your Lambdas and ensure your Fluent API remains stable:
1. Consistent Entry and Exit Points
Ensure that your Fluent API has predictable entry and exit points for users. For example, initialization and final processing ("processing" in our example) should be clearly distinguishable and consistent.
2. Immutability for Chain Integrity
Prevent side effects that could disrupt the chain. Immutable objects ensure that the state doesn't unexpectedly change between method calls.
public class EventProcessor {
private final Map<String, Consumer<Event>> eventHandlers;
// Constructor and other methods
public EventProcessor on(String eventName, Consumer<Event> handler) {
Map<String, Consumer<Event>> newHandlers = new HashMap<>(eventHandlers);
newHandlers.put(eventName, handler);
return new EventProcessor(newHandlers);
}
// ...
}
By returning a new EventProcessor
object, rather than altering the existing one, we're guaranteeing that our Fluent API calls won't have unintended side effects.
3. Limit Lambda Complexity
Lambdas in an API should be simple and focused. Complicated Lambdas can decrease readability and make debugging difficult. If your Lambda surpasses a couple of lines, consider refactoring the logic into a separate method or class.
EventProcessor processor = new EventProcessor()
.on("click", this::handleClick) // handleClick is a method reference with clear logic.
.process();
4. Type Safety
Your API should enforce type safety by accepting Lambdas of a particular functional interface that align with the operation. Using Predicate
, Function
, Consumer
, and the like can help ensure that only Lambdas with the right signature and return type are being used.
5. Clear Lambda Context
It should always be clear to the API user what the Lambda they pass is for. Naming your methods and parameters clearly can significantly improve the readability and maintainability of your Fluent API.
public EventProcessor on(String eventName, Consumer<Event> eventAction) {
// ...
}
In the above method signature, it is evident that the second parameter is an action to be performed on an event.
6. Exception Handling
Lambdas in Java can't throw checked exceptions without some boilerplate. Your API should be designed so that lambda users don't have to clutter their code with try-catch blocks.
public EventProcessor on(String eventName, Consumer<Event> eventAction) {
return on(eventName, (Event e) -> {
try {
eventAction.accept(e);
} catch (Exception ex) {
// Handle exception or wrap it in an unchecked exception
throw new RuntimeException(ex);
}
});
}
In this example, the on
method internally handles exceptions, leading to a cleaner API surface for the client code.
Examples of Fluent APIs with Lambdas
Let's explore a practical example showing Fluent API's power combined with Lambdas. Assume we're working with a QueryBuilder
for a database:
List<Record> records = new QueryBuilder()
.select("name", "age", "email")
.from("users")
.where(user -> user.getAge() > 30)
.orderBy("age")
.limit(10)
.execute();
The QueryBuilder
class uses Lambdas in the where
method to filter records. Each method in the Fluent API returns a QueryBuilder
object, allowing for the chain to continue uninterrupted.
Conclusion
Lambdas and Fluent APIs are like bread and butter for modern Java developers. They can significantly enhance your code's readability and maintainability when used wisely. By abiding by principles like consistency, immutability, complexity management, type safety, context clarity, and proper exception handling, you'll be well on your way to designing APIs that are robust and intuitive.
Remember, the goal is not merely to create syntactically concise code but to craft an API that feels like an extension of the language itself, enabling developers to communicate their intentions effortlessly. Now that you've grasped the elements of stability in Fluent API design with Lambdas, you're set to bring clarity and power to your Java applications.
In the spirit of continuous learning and improvement, look to the Stream API and other Fluent interfaces in Java's standard library for inspiration. Dive in, experiment, and watch your Java code transform with the grace and strength of a well-designed Fluent API. Happy coding!
Note: This blog post assumes a foundational understanding of Java Lambdas and functional interfaces. For additional background, consider exploring the official Oracle Java tutorials on these topics.