Overcome Java Dynamic Proxy Limitations Easily!
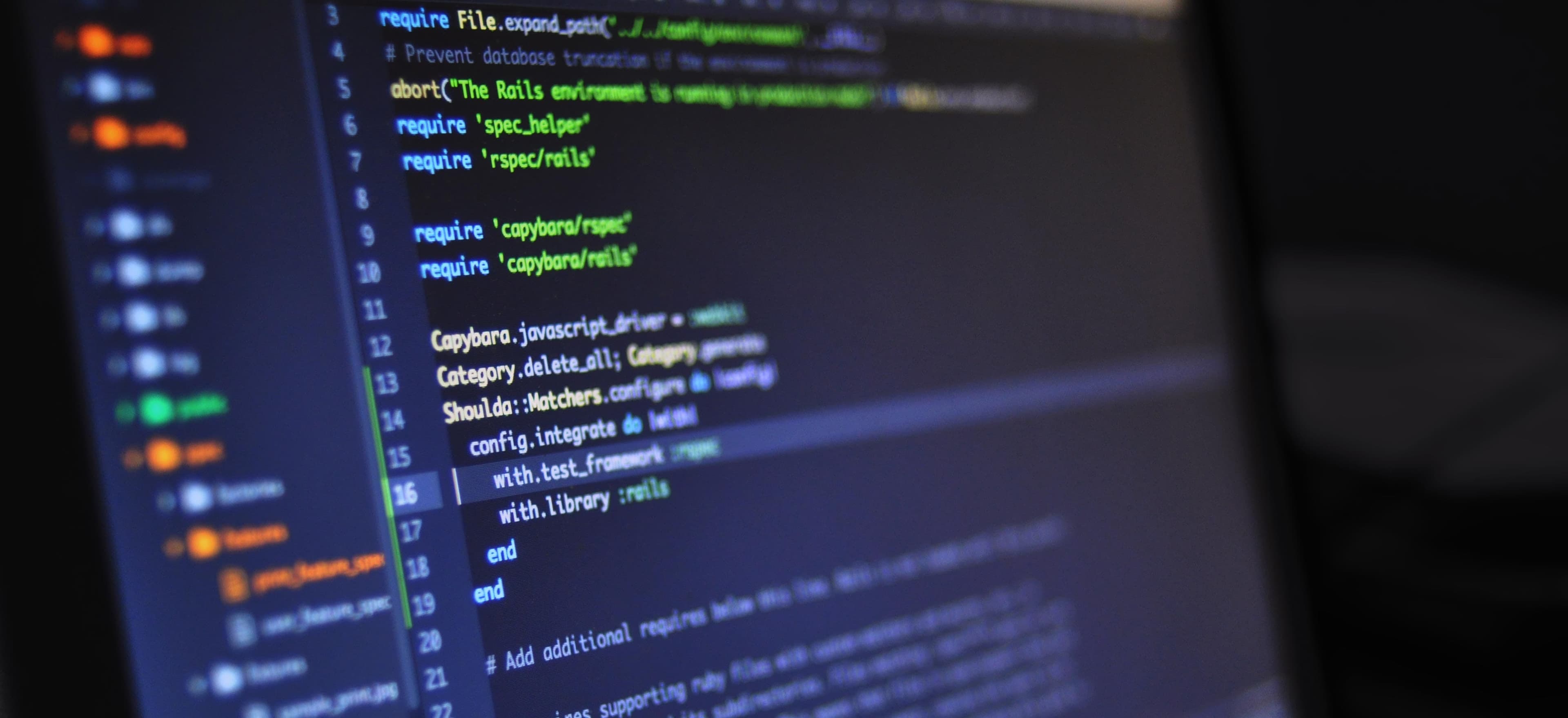
- Published on
Overcome Java Dynamic Proxy Limitations Easily!
Java's dynamic proxy is a powerful mechanism for creating lightweight and reusable code. It allows you to dynamically create proxy instances of interfaces at runtime, providing an elegant way to handle cross-cutting concerns such as logging, access control, and transaction management. However, as with any powerful tool, there are limitations you must be aware of to fully leverage its potential.
In this post, we'll dive into the strengths of Java's dynamic proxy, its inherent limitations, and strategies for overcoming those limitations to elevate your Java code.
Understanding Java Dynamic Proxy Mechanism
Before we delve into overcoming limitations, it's vital to comprehend what Java dynamic proxies are and why they are used. In Java, a Proxy
class provides static methods for creating dynamic proxy instances that implement specified interfaces. This is achieved through an InvocationHandler
, which is a piece of code that intercepts method calls made on the proxy instance.
Creating a Dynamic Proxy in Java
import java.lang.reflect.*;
public class DynamicProxyExample {
public interface HelloWorld {
void sayHello();
}
static class HelloWorldImpl implements HelloWorld {
public void sayHello() {
System.out.println("Hello World");
}
}
static class HelloWorldHandler implements InvocationHandler {
private final HelloWorld target;
public HelloWorldHandler(HelloWorld target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before method call");
Object result = method.invoke(target, args);
System.out.println("After method call");
return result;
}
}
public static void main(String[] args) {
HelloWorld helloWorld = new HelloWorldImpl();
InvocationHandler handler = new HelloWorldHandler(helloWorld);
HelloWorld proxyInstance = (HelloWorld) Proxy.newProxyInstance(
HelloWorld.class.getClassLoader(),
new Class<?>[] {HelloWorld.class},
handler
);
proxyInstance.sayHello();
}
}
In the above example, the HelloWorld
interface is implemented by the HelloWorldImpl
class. We define an InvocationHandler
(HelloWorldHandler
) to wrap around the HelloWorld
object. The proxyInstance.sayHello()
method call is intercepted by the HelloWorldHandler
, enhancing the method call with additional functionality.
Why Use Java Dynamic Proxy?
- Decoupling Code: By using dynamic proxies, you separate the cross-cutting concerns from the core business logic, making your code more maintainable and cleaner.
- Abstraction Layer: It provides an abstraction layer, allowing for transparent introduction of additional functionality without modifying the original codebase.
- Implementing AOP: It can be used to implement Aspect-Oriented Programming (AOP) in Java without the need for a full-fledged AOP framework.
The Limitations of Java Dynamic Proxy
While utilizing Java's dynamic proxy can lead to elegant designs, it does have its share of limitations:
- Interface Dependency: Java dynamic proxy only works with interfaces. If you need to proxy a concrete class, you're out of luck.
- Reflection Overhead: It uses reflection, which can introduce performance overhead and make your code a bit slower.
- Code Complexity: The proxy code can get complex and challenging to understand, especially when debugging or reading through an extensive stack trace.
Overcoming Proxy Limitations
To lift the limitations imposed by Java's dynamic proxy, let's explore some solutions:
1. Using Libraries Like CGLIB or ByteBuddy
When you want to create a proxy for classes and not just interfaces, libraries like CGLIB and ByteBuddy come to the rescue. They can create subclasses dynamically and override methods, making it possible to proxy concrete classes.
CGLIB Example
import net.sf.cglib.proxy.Enhancer;
import net.sf.cglib.proxy.MethodInterceptor;
public class CglibProxyExample {
public static class ConcreteClass {
public void concreteMethod() {
System.out.println("Concrete Method Executed");
}
}
public static void main(String[] args) {
Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(ConcreteClass.class);
enhancer.setCallback((MethodInterceptor) (obj, method, args1, proxy) -> {
System.out.println("Before concrete method call");
Object result = proxy.invokeSuper(obj, args1);
System.out.println("After concrete method call");
return result;
});
ConcreteClass proxyInstance = (ConcreteClass) enhancer.create();
proxyInstance.concreteMethod();
}
}
In this example, CGLIB is used to create a dynamic proxy for a concrete class. The Enhancer
class is responsible for generating a subclass at runtime where the concreteMethod
is overridden to include additional behavior.
2. Optimize Reflection Usage
When it comes to performance overhead due to reflection, optimize by caching Method
instances or using method handles (java.lang.invoke.MethodHandle
) available from Java 7 onwards, which provide a more performant alternative to reflection for certain use cases.
3. Simplify Debugging
To avoid getting lost in complex stack traces when debugging dynamic proxies, good logging practices are essential. Including explanatory log messages can help trace the proxy's behavior and pinpoint issues faster.
4. Assess Your Needs
Lastly, always evaluate whether you need a dynamic proxy or if there are simpler solutions that suffice. Sometimes, using a design pattern like Decorator can achieve similar results without introducing proxy complexity.
Conclusion
Java dynamic proxies are an excellent tool for abstracting cross-cutting concerns, allowing you to write cleaner and more maintainable code. However, they're not a one-size-fits-all solution. By understanding their limitations and knowing how to work around them, you can make an informed decision about when and how to use them effectively.
Using libraries like CGLIB and ByteBuddy to overcome the interface-only limitation, optimizing reflection, simplifying debugging, and critically assessing your use case are all strategies for overcoming the downsides of Java dynamic proxies. With the right approach, you can harness their full potential and write robust, flexible Java applications.
For more information on Java's dynamic proxy and related concepts, consider exploring the Java documentation on Proxies, or investigate libraries like CGLIB and ByteBuddy to take your proxy implementation to the next level.