Losing Data in Java: The Long to Int Conversion Trap
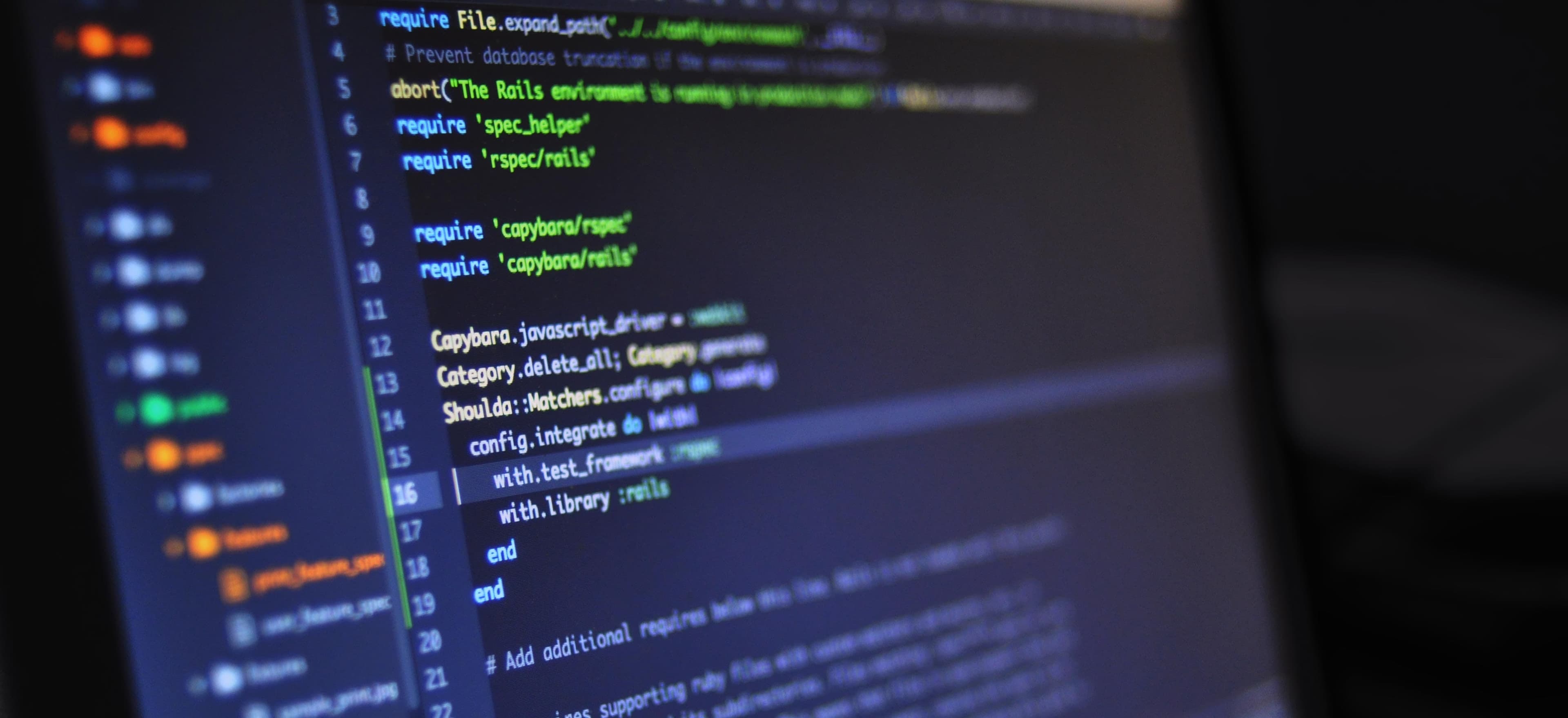
- Published on
Losing Data in Java: The Long to Int Conversion Trap
In Java, type casting is a common practice that allows us to convert a variable from one data type to another. However, when it comes to converting from a larger-capacity type to a smaller one, such as from long
to int
, you might fall into a trap: the risk of data loss. This post will discuss this potential pitfall, how to avoid it, and best practices for type conversion in Java.
Understanding Primitive Types
Before we delve into the specifics of type conversion, let's briefly revisit the primitive data types in Java. Java provides several primitive data types including int
, long
, float
, double
, and more. An int
is a 32-bit signed integer, while a long
is a 64-bit signed integer. This means a long
can hold a much larger range of values than an int
.
int maxInt = Integer.MAX_VALUE; // 2^31 - 1
long maxLong = Long.MAX_VALUE; // 2^63 - 1
Here, maxInt
can store a maximum value of 2,147,483,647, while maxLong
can go up to 9,223,372,036,854,775,807.
The Conversion Process
Type casting from long
to int
is straightforward -- you can use a simple cast:
long myLong = 12345678901L;
int myInt = (int) myLong;
This code compiles, but therein lies the trap. If myLong
contains a value that fits within the range of int
, the conversion works fine. But if the value of myLong
exceeds Integer.MAX_VALUE
, the result is a truncated value that won't accurately represent the original long
.
Why Is This a Problem?
In programming, data integrity is paramount. If you're expecting a faithful representation of your numerical data after conversion, and instead you get a corrupted value, this can lead to incorrect calculations, logic errors, unseen bugs, and a whole range of potential issues in your application that can be hard to diagnose.
Detecting the Trap
To safely convert from long
to int
, you must ensure that the long
value is within the range of an int
. Here is one way to perform this check:
if (myLong > Integer.MAX_VALUE || myLong < Integer.MIN_VALUE) {
throw new ArithmeticException("Value out of int range!");
}
int safeInt = (int) myLong; // Now it's safe to cast
This check prevents the data loss by throwing an exception if an overflow or underflow would occur.
Using Java 8's Math.toIntExact()
Java 8 introduced Math.toIntExact(long value)
, which does the check for you and throws an ArithmeticException
if the long
value does not fit into an int
.
try {
int exactInt = Math.toIntExact(myLong);
} catch (ArithmeticException e) {
System.err.println("Cannot convert to int safely: " + e.getMessage());
}
By using Math.toIntExact
, your intent is clear and your code is protected from inadvertent data corruption.
Real-world Implications
Consider a situation where an application is tracking the population of a city. You might start with an int
but as the city grows, you decide to switch to a long
to anticipate larger numbers. At some point, you might need to interface with legacy code or APIs that still use int
. Converting back from long
to int
without due diligence could distort your population data, leading to incorrect statistics or resource allocations.
Best Practices for Type Conversion
When performing type conversions, follow these guidelines to avoid data loss:
- Understand the Types: Be aware of the value ranges different types can hold.
- Check Before You Cast: Always check if the value fits within the target type range before casting.
- Use Java's Built-in Methods: When available, use built-in methods like
Math.toIntExact()
. - Handle Exceptions Gracefully: Implement proper exception handling to catch any overflows.
- Use Larger Types When Necessary: If there's potential for a variable to exceed
int
range, uselong
from the start. - Perform Thorough Testing: Include edge cases for out-of-bounds values when unit testing.
- Code Review: Have another set of eyes review the code, as they may catch potential casting issues you overlooked.
- Avoid Unnecessary Casting: Only perform casts when absolutely necessary.
Conclusion
Type conversion in Java, particularly from long
to int
, is a process fraught with potential for data loss if not handled with care. By understanding the implications and ensuring the safe handling of conversion, you can avoid introducing subtle bugs that might only surface under certain circumstances. Using the available built-in methods like Math.toIntExact()
and adhering to best practices will protect the integrity of your data and the robustness of your Java applications.
Remember, coding defensively when it comes to data types is not about anticipating every single failure but about implementing sensible checks and balances to maintain data integrity at all times. Keep this in mind, and you'll steer clear of the long to int conversion trap in Java.
For further reading, check out the official Java documentation on primitive data types: Primitive Data Types and learn more about exception handling in Java at The Java™ Tutorials.
Happy coding, and stay vigilant against data loss!