Fixing JSON-Pojo Mapping in Java WebSockets JSR 356
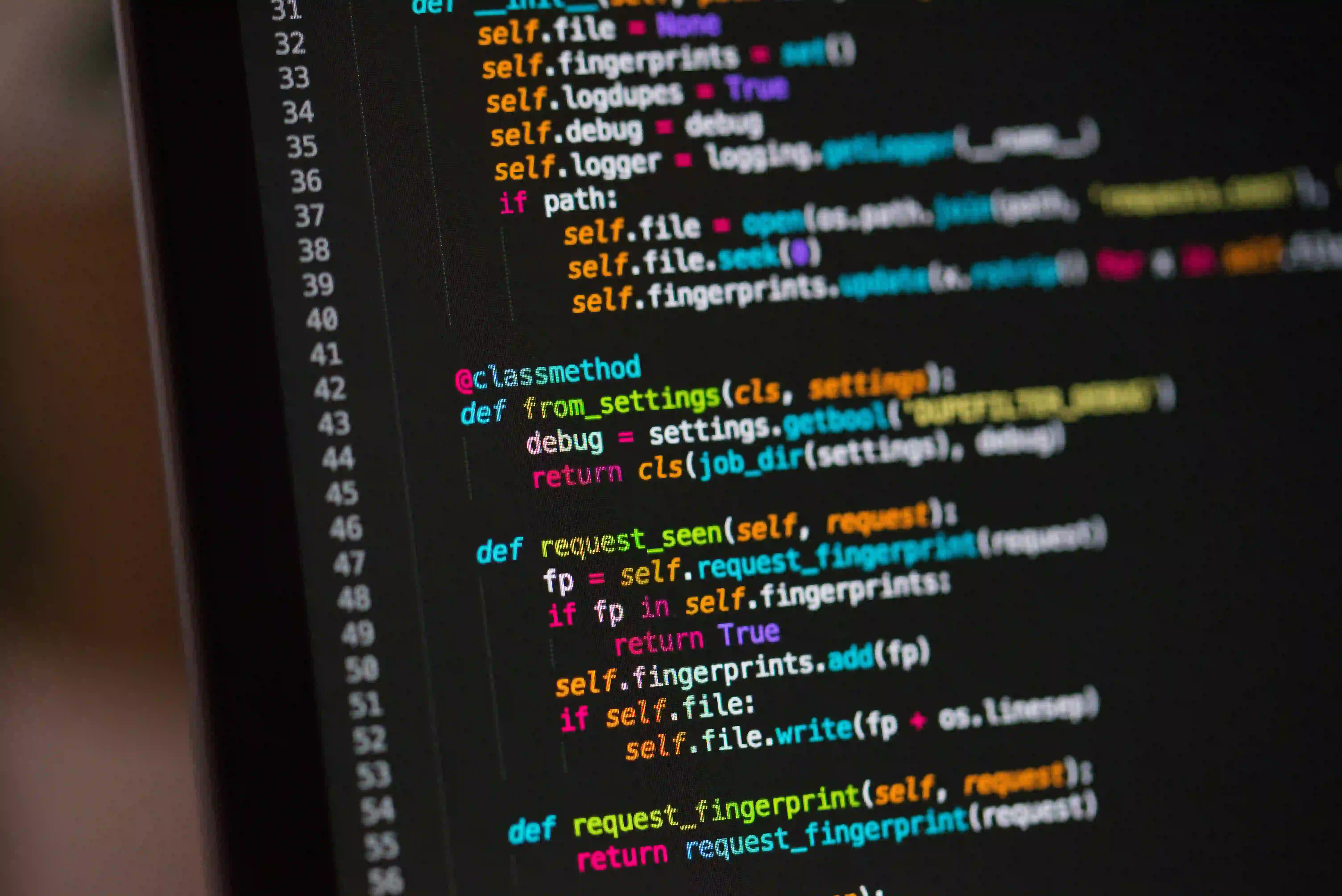
Fixing JSON-Pojo Mapping in Java WebSockets JSR 356
When building modern interactive web applications, real-time communication is often a requisite. Java provides a solid foundation for such implementations with its Java API for WebSocket (JSR 356). However, one challenge that many Java developers face when working with WebSockets is the proper mapping between JSON data and Plain Old Java Objects (POJOs). This article will provide an overview of this challenge and will guide you through a solution to seamlessly serialize and deserialize data within a WebSocket context.
Understanding the Problem
The WebSocket protocol allows two-way communication channels over a single TCP connection. In the Java landscape, the API for WebSocket (part of the specification JSR 356) typically deals with text and binary messages. JSON being a predominant format for data interchange on the web, it becomes necessary to efficiently convert JSON to Java objects (and vice versa) to streamline data handling within WebSocket endpoints.
The conversion process often involves boilerplate code, which can lead to errors and inefficiencies. Let's understand this with a simple example.
@ServerEndpoint("/websocket")
public class MyWebSocketServer {
@OnMessage
public void onMessage(String message, Session session) {
Pojo myPojo = new Gson().fromJson(message, Pojo.class);
// Handle the POJO
}
}
In this snippet, every message received needs to be manually parsed using Gson (or any other JSON processing library). This practice can clutter the onMessage
method, especially when dealing with multiple message types.
A Streamlined Approach
A cleaner approach would be to use a custom decoder that automatically handles the conversion from JSON to POJO. This allows WebSocket endpoint methods to work directly with the relevant Java objects, reducing clutter and potential mistakes.
Implementing a Custom Decoder
To begin with, you will need a POJO that represents the data structure of your JSON messages.
public class MyPojo {
// Pojo properties
// Standard getters and setters
}
Next, implement the custom decoder by extending either javax.websocket.Decoder.Text
or javax.websocket.Decoder.TextStream
. For simplicity, we'll extend Decoder.Text
.
public class MyPojoDecoder implements Decoder.Text<MyPojo> {
private static Gson gson = new Gson();
@Override
public MyPojo decode(String s) throws DecodeException {
return gson.fromJson(s, MyPojo.class);
}
@Override
public boolean willDecode(String s) {
// Implement logic to determine if the string can be decoded into MyPojo
return true; // For simplicity, we return true
}
@Override
public void init(EndpointConfig config) {
// Called when the decoder is initialized.
}
@Override
public void destroy() {
// Called when the decoder is about to be destroyed.
}
}
Here, gson
is an instance of Gson we'll use to handle JSON parsing. The willDecode
method should ideally contain logic to check if the string can be converted into MyPojo
before calling the decode
method.
You must also create an equivalent custom encoder to transform POJOs back to JSON strings:
public class MyPojoEncoder implements Encoder.Text<MyPojo> {
private static Gson gson = new Gson();
@Override
public String encode(MyPojo myPojo) throws EncodeException {
return gson.toJson(myPojo);
}
@Override
public void init(EndpointConfig config) {
// Encoder initialization logic
}
@Override
public void destroy() {
// Encoder cleanup logic
}
}
Registering the Encoder and Decoder
After implementing the encoder and decoder, you must register them with your WebSocket endpoint. Use the @ServerEndpoint
annotation's encoders
and decoders
attributes:
@ServerEndpoint(
value = "/websocket",
encoders = {MyPojoEncoder.class},
decoders = {MyPojoDecoder.class}
)
public class MyWebSocketServer {
@OnMessage
public void onMessage(MyPojo myPojo, Session session) {
// Business logic with myPojo
}
}
Now, the WebSocket server endpoint will automatically decode JSON messages into MyPojo
instances and encode MyPojo
instances to JSON strings when sending messages to the client.
Optional: Leveraging the JSON-P API
If you're running on a Java EE 7 or newer platform, you could also make use of the JSON-P (Processing) API (javax.json
package) instead of Gson for the conversion processes:
import javax.json.bind.Jsonb;
import javax.json.bind.JsonbBuilder;
public class MyPojoDecoder implements Decoder.Text<MyPojo> {
private static Jsonb jsonb = JsonbBuilder.create();
@Override
public MyPojo decode(String s) throws DecodeException {
return jsonb.fromJson(s, MyPojo.class);
}
// Other methods remain the same
}
And for the encoder:
public class MyPojoEncoder implements Encoder.Text<MyPojo> {
private static Jsonb jsonb = JsonbBuilder.create();
@Override
public String encode(MyPojo myPojo) throws EncodeException {
return jsonb.toJson(myPojo);
}
// Other methods remain the same
}
The JSON-P API is part of the Java EE standard and thus integrated into it, offering a portable and standard approach for JSON processing in a Java EE environment.
Conclusion
By implementing custom encoders and decoders to handle JSON-Pojo mapping in Java WebSocket applications (JSR 356), you reduce boilerplate code, minimize parsing errors, and streamline your data flow. While we've used Gson in our examples, you can leverage other libraries like Jackson or the JSON-P API for Java EE applications to achieve similar results.
Opting for a solution like this aids maintainability, enhances clarity, and conforms better to the separation of concerns principle. Your WebSocket servers become lighter, more focused on business logic, and agnostic to the serialization/deserialization processes. As a Java developer, these optimizations can significantly improve the quality and robustness of your real-time web application's server-side component.
For more extensive resources on Java WebSockets and JSON processing in Java, refer to the official Java EE 7 WebSocket API documentation and the Gson User Guide.