Boosting ROI: Mastering Efficient Enterprise Testing
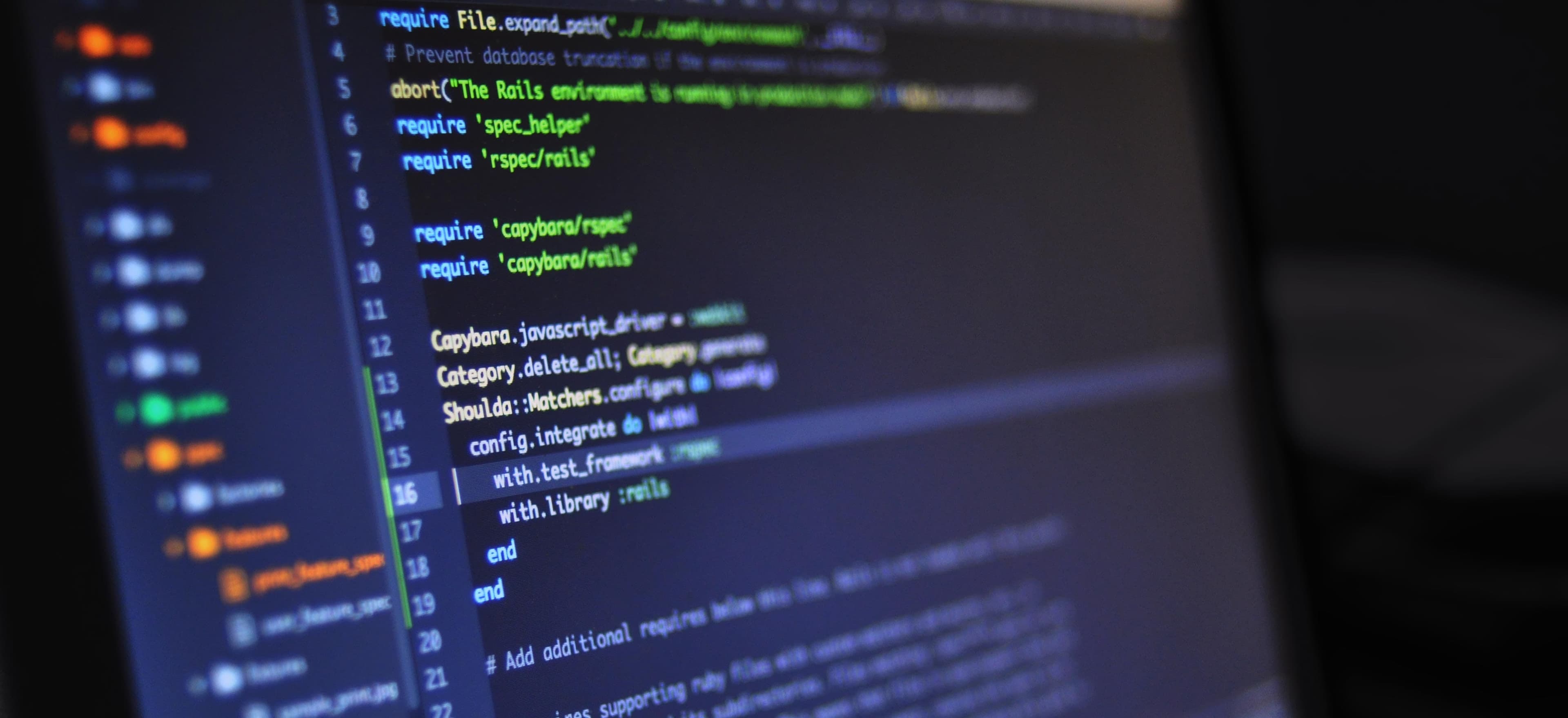
- Published on
Boosting ROI: Mastering Efficient Enterprise Testing in Java
In the quest for digital excellence, Java remains the cornerstone for building robust and scalable enterprise applications. As businesses strive for higher returns on investment (ROI), the focus often pivots to one crucial aspect: Efficient Enterprise Testing. By streamlining your Java testing strategy, you not only ensure code reliability but also substantially cut down costs and time-to-market, key ingredients for a savory ROI.
In this post, we'll delve deep into tactics and best practices that can dramatically enhance your Java testing efficiency.
Understanding the ROI of Testing
Before plunging into the technicalities, it's imperative to grasp why testing holds such a pivotal role in ROI. Testing is not merely a bug-catching phase; it's a quality assurance process that, when executed efficiently, reduces maintenance costs, increases customer satisfaction, and minimizes the risk of expensive failures post-deployment.
Automated Testing: The ROI Booster
Test automation in Java is akin to hiring an indefatigable, precision-driven army that works 24/7 without fail. Tools like JUnit, TestNG, and Selenium for web-based applications have revolutionized the testing domain.
JUnit and TestNG: The Backbone of Java Unit Testing
JUnit and TestNG are indispensable tools for Java developers. They provide a framework for writing and running repeatable tests.
@Test
public void givenNumber_whenEvenCheck_thenTrue() {
assertTrue(MyUtils.isNumberEven(22)); // Robust unit test case
}
Here, the @Test
annotation indicates a unit test method. The real power lies in understanding the "why": Why is this test case robust? It's specific, isolated, and checks a single condition - evenness of a number.
Leveraging Selenium for Web Testing
Selenium unleashes its prowess for Java-based web application testing. By automating browser actions, it simulates a real user's interaction, ensuring the application works seamlessly across different environments.
WebDriver driver = new ChromeDriver();
driver.get("https://your-application.com");
WebElement loginButton = driver.findElement(By.id("login"));
loginButton.click();
Here, you're using Selenium's WebDriver API to automatically launch a browser, navigate to a URL, find the login button by its ID, and actually click it. This is efficiency incarnate, replacing repetitive, manual testing.
Integration Testing: Ensuring All Pieces Fit Together
While unit tests focus on the smallest parts of an application, integration tests verify that the different modules or services work together as expected. Tools like Mockito help simulate the behavior of external dependencies, providing a controlled environment for your integration testing needs.
@Test
public void whenCallingExternalService_thenExpectedResultReturned() {
ExternalService mockService = Mockito.mock(ExternalService.class);
Mockito.when(mockService.retrieveData()).thenReturn("Mock Data");
String result = new BusinessLogic().handleExternalData(mockService);
assertEquals("Mock Data Processed", result); // Asserting correct data processing
}
The "why" here is pivotal; by mocking the external service, you're not testing the service itself, but rather how your application reacts to its data. You're isolating the test scope to just the integration point.
Performance Testing: The Speed Check
A high-performing application isn't just a nice-to-have, it's a must-have, and this is where performance testing enters the scene. Tools like Apache JMeter can simulate a swarm of users interacting with your application, letting you find bottlenecks and performance issues before they become real problems.
Building a Culture of Quality
Investing in testing is only half the battle. Creating a culture that prioritizes quality is just as crucial, as it empowers the entire team to strive for excellence at every development cycle stage.
Continuous Integration and Continuous Delivery (CI/CD)
CI/CD platforms such as Jenkins have become the lifeline of an agile Java development team. By automating the integration and delivery process, you can run your robust test suite regularly and get immediate feedback.
pipeline {
agent any
stages {
stage('Test') {
steps {
sh 'mvn test' // Runs the Maven test phase
}
}
}
post {
success {
echo 'Tests Passed - Ready for Deployment!'
}
}
}
In this Jenkins Pipeline script, every code commit triggers a series of actions, including running all tests. A successful test phase leads to the next deployment step, embedding quality checks into the very fabric of your development workflow.
Best Practices for Enhanced Test Efficiency
-
Write Testable Code: Apply SOLID principles and design patterns to simplify writing tests. Favor composition over inheritance, use interfaces, and dependency injection for easier mocking.
-
Test Early, Test Often: Shift-left testing by integrating testing into the initial stages of the development cycle can catch defects earlier, when they're cheaper to fix.
-
Prioritize Your Tests: Not all tests are equal; identify critical business features and apply risk-based testing to focus efforts where they matter most.
-
Maintain Your Test Code: Refactor and review test code with the same rigor as production code. This is a non-negotiable for test reliability.
-
Measure Your Success: Use coverage tools like Jacoco for Java to understand what you're testing and what you're not. However, don't fall into the trap of aiming for 100% code coverage. Aim for meaningful coverage where it counts.
-
Knowledge Sharing: Encourage knowledge transfer about effective testing within your team. Workshops and pair programming sessions can be invaluable.
Conclusion
In the realm of enterprise application development in Java, efficient enterprise testing isn't a choice; it's an imperative for boosting ROI. By embracing a strategic testing approach, leveraging powerful tools, and fostering a culture of quality, your Java projects will not only scale new heights in performance but also deliver that coveted increase in ROI.
Remember, an investment in your testing strategy is an investment in your product's future. The resources you allocate to testing today will reward you manifold tomorrow, solidifying your stance in an ever-competitive market. And with Java, you're well-equipped with an arsenal of best-in-class tools and practices to ensure that your investment yields the highest possible returns.