Turning Failure to Success: Master the Art of Bouncing Back!
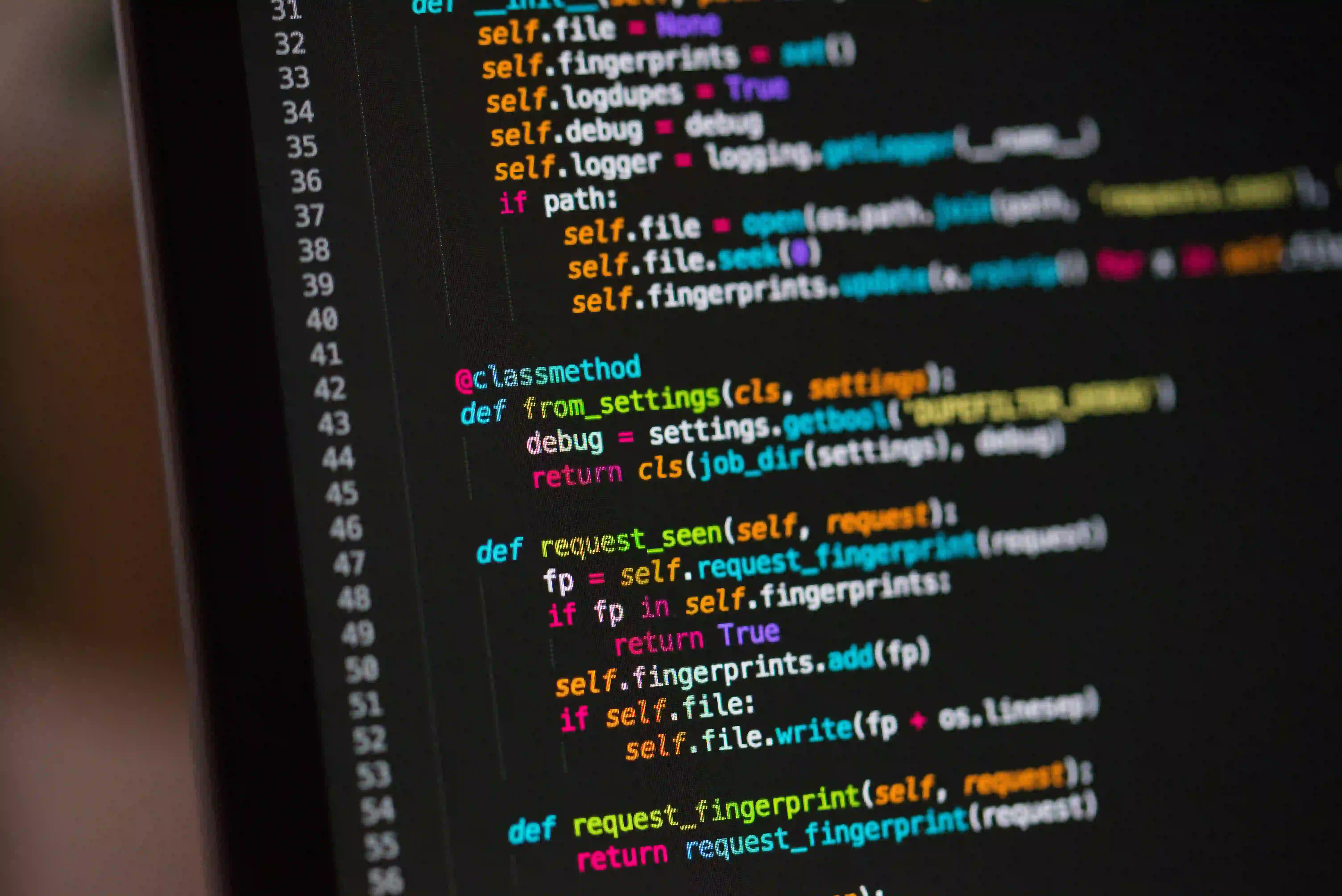
Turning Failure to Success: Master the Art of Exception Handling in Java
In the world of programming, particularly in Java, the line between success and failure can be quite thin. A minor bug or an unhandled scenario can lead to application crashes or, worse, compromised system security. Yet, within these challenges lie opportunities for us to excel—by mastering the art of bouncing back through robust exception handling mechanisms. In this post, we'll explore the intricacies of exception handling in Java, providing insights, code samples, and best practices to help you turn potential failures into successes.
Understanding Java Exceptions
Before we dive into handling exceptions, let's clarify what they are. In Java, an exception is an event that disrupts the normal flow of the program. It's Java's way of saying, "I've encountered a situation I don't know how to deal with; please take care of it."
Exceptions in Java fall into two main categories:
- Checked Exceptions: These exceptions must be either caught or declared in the method's
throws
clause. Examples includeIOException
andSQLException
. - Unchecked Exceptions: Also known as runtime exceptions, they don't need to be explicitly handled. Examples include
NullPointerException
andIndexOutOfBoundsException
.
Grasping the nature of these exceptions is crucial for writing resilient Java applications.
Exception Handling in Java: The Basics
The try-catch block is Java's primary mechanism for handling exceptions. Here's a simple example:
try {
// Code that may throw an exception
int data = 50 / 0;
} catch (ArithmeticException e) {
// Code to handle the exception
System.out.println("Cannot divide by zero!");
}
In the snippet above, we attempt to divide 50 by zero, which will throw an ArithmeticException
. The catch block catches the exception, allowing us to handle it gracefully, ensuring that our program doesn't abruptly cease.
The Why Behind Try-Catch
Why use a try-catch block? It's about control. When an exception occurs, we want the power to decide how the program should respond. Ignoring exceptions is not an option, as it leads to unstable and insecure applications. Proper exception handling provides a safety net, allowing our application to remain operational even when confronted with unexpected scenarios.
Best Practices for Exception Handling
To harness the full potential of exception handling in Java, here are some best practices:
1. Catch Specific Exceptions
Always catch the most specific exception first before moving to more general exceptions. This approach provides clarity to your error handling logic and makes your code more readable.
try {
// Code that might throw multiple exceptions
} catch (FileNotFoundException e) {
// Handle file not found case
} catch (IOException e) {
// Handle other IO exceptions
} catch (Exception e) {
// Fallback for any other Exception
}
2. Avoid Catching Throwable
Throwable
is the superclass of all errors and exceptions in Java. You should avoid catching it directly, as it encompasses both Error
(which you normally don't want to handle) and Exception
.
3. Don't Catch NullPointerException
Explicitly
A NullPointerException
often points to a bug in your code, such as not initializing an object before use. Rather than catching it, it's better to check for null references and handle them prior to getting to an operation where a NullPointerException
might occur.
if (objectReference != null) {
objectReference.someMethod();
} else {
// Handle null case
}
4. Don't Ignore Exceptions
An empty catch block is a common anti-pattern. Ignoring exceptions can lead to subtle bugs that are hard to detect.
try {
// Risky operations
} catch (IOException e) {
// At the very least, log the exception
e.printStackTrace();
}
5. Use Finally Blocks
A finally
block contains code that must be executed regardless of whether an exception occurs or not. This is particularly useful for releasing resources like file handles or database connections.
try {
// Work with resources
} catch (IOException e) {
// Handle exception
} finally {
// Release resources
}
6. Document Your Exceptions
If your method can throw a checked exception, document it with the @throws
JavaDoc tag. This is important for anyone using your method to understand what kinds of exceptions they should handle.
/**
* This method does something that can cause an IOException.
*
* @throws IOException if an I/O error occurs
*/
public void doSomething() throws IOException {
// code
}
Advanced Techniques: Custom Exceptions & More
Beyond the basics, creating your own exception classes can aid in conveying specific error conditions:
public class UserNotFoundException extends Exception {
public UserNotFoundException(String message) {
super(message);
}
}
Using these custom exceptions in your code makes it clearer what kind of error occurred, as opposed to using generic exception classes.
Wrapping Up: Turning Mistakes into Lessons
Effective exception handling is vital in Java. By capturing, logging, and providing useful feedback on exceptions, we not only make our code resilient but also turn potential pitfalls into opportunities for learning and improvement. A proper handling strategy ensures a smoother user experience and equips us with valuable insights that can feed into future development iterations.
While exceptions are generally seen as errors, in the realm of software development, they are navigational beacons that guide us towards writing more robust, user-friendly applications. Harnessing the power of Java's exception handling capabilities propels us from simply reacting to issues to proactively managing and mitigating them.
In sum, master the art of exception handling in Java, and you'll be well-equipped to turn every failure into a stepping stone towards success!