Unlocking the Potential of JavaFX 20 Charts
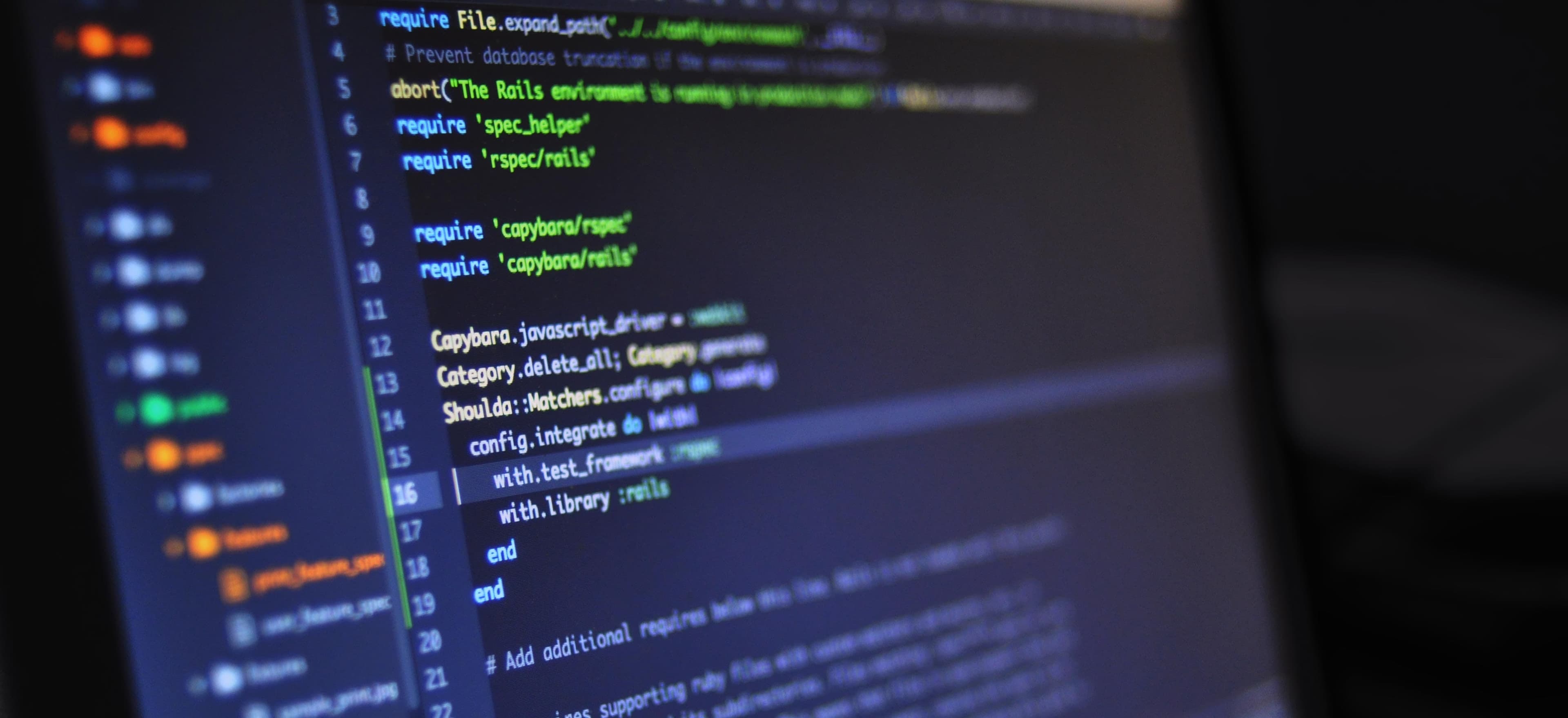
- Published on
Unlocking the Potential of JavaFX 20 Charts: A Guide for Developers
JavaFX 20, the latest iteration of Oracle's rich client platform for building cross-platform applications, comes with an enhanced suite of features designed to give developers unparalleled power to create visually compelling and responsive UIs. Among these features, JavaFX Charts offer a potent way to represent data graphically, enhancing comprehension and user engagement. In this blog post, we will delve into the capabilities of JavaFX 20 charts, covering how to harness their potential in your Java applications.
Understanding JavaFX Charts
Charts are pivotal in conveying complex data in a digestible format. JavaFX provides a collection of pre-built chart classes, such as line charts, bar charts, area charts, pie charts, and scatter charts, each designed for specific data visualization needs.
Why choose JavaFX for charts, you might ask? JavaFX charts boast an intuitive API, are easily customizable, and integrate seamlessly with other JavaFX components, offering a consistent and aesthetically pleasing UX design.
To get started with JavaFX charts, ensure you are using JDK with JavaFX 20 support. If your setup requires a standalone JavaFX SDK, you can download it from Gluon's JavaFX page.
A Dive into Chart Creation
Creating a chart in JavaFX is straightforward. Let's go through an example using a Line Chart, one of the most versatile and widely used chart types.
Step 1: Setting Up Your Project
To initialize a JavaFX project, you can use an IDE like Eclipse or IntelliJ IDEA with JavaFX support or configure your build tools like Maven or Gradle to manage dependencies.
Here's a simple Maven dependency for JavaFX:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>20</version>
</dependency>
Step 2: Creating a Line Chart
JavaFX charts reside within the javafx.scene.chart
package. The following example creates a simple Line Chart illustrating hypothetical sales data over a year.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.chart.LineChart;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.XYChart;
import javafx.stage.Stage;
public class LineChartExample extends Application {
@Override
public void start(Stage stage) {
stage.setTitle("Sales Data Visualization");
// Defining the axes
NumberAxis xAxis = new NumberAxis();
NumberAxis yAxis = new NumberAxis();
xAxis.setLabel("Month");
yAxis.setLabel("Sales");
// Creating the Line Chart
LineChart<Number, Number> lineChart = new LineChart<>(xAxis, yAxis);
lineChart.setTitle("Monthly Sales Overview");
// Prepare the series of data
XYChart.Series<Number, Number> series = new XYChart.Series<>();
series.setName("Sales in 2023");
// Populating the series with data
series.getData().add(new XYChart.Data<>(1, 230));
series.getData().add(new XYChart.Data<>(2, 197));
series.getData().add(new XYChart.Data<>(3, 260));
// ... Continue adding data for remaining months
// Adding series to the chart
lineChart.getData().add(series);
// Setting the scene
Scene scene = new Scene(lineChart,800,600);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Step 3: Running Your Application
Execute the main
method, and you should see a window rendering a Line Chart with the provided data.
A few points to note in the code:
- The axes are instances of
NumberAxis
, which can be replaced withCategoryAxis
if your x-axis represents non-numeric categories. - The
XYChart.Series
object encapsulates all data points for a particular series — in our case, the sales data for 2023. - By adding the series to the
LineChart
instance, the chart becomes aware of the data it needs to plot.
Step 4: Customizing Your Chart
JavaFX charts offer numerous customization options. For instance, let's make the data points stand out using markers.
lineChart.setCreateSymbols(true); // Enables the display of symbols on each data point
To style your charts, you can apply CSS. JavaFX charts are stylable via CSS just like other JavaFX components, which provides powerful customizability without altering your Java code.
Example CSS for a Line Chart:
.chart-line-symbol {
-fx-background-color: #ff0000, white;
-fx-background-insets: 0, 2;
-fx-background-radius: 5px;
-fx-padding: 5px;
}
.line-chart {
-fx-background-color: linear-gradient(to bottom, #61a2b1, #2A5058);
}
Save this CSS as chart-style.css
and load it in your JavaFX scene like so:
scene.getStylesheets().add(getClass().getResource("chart-style.css").toExternalForm());
Expanding Your Chart Knowledge
While the above steps give you a basic line chart, JavaFX provides more elaborate features to add to your charts like event handling, animation, and customization via skins.
Event Handling
Interactive charts can provide additional context to the user when they hover over or click on data points. You can utilize JavaFX's event handling to make your charts respond to user actions:
for (XYChart.Data<Number, Number> data : series.getData()) {
data.getNode().setOnMouseEntered(event -> {
// display pop-up, highlight data point, etc.
});
}
Animation
JavaFX charts have built-in animation which you can easily enable or disable:
lineChart.setAnimated(false); // Turn off animation
Skins
Skins in JavaFX allow you to define the visual structure of controls. Custom chart skins can govern the rendering and interaction of your chart in a more fundamental way. Skins can be applied by extending base chart skins like ChartSkin
or AreaChartSkin
.
Conclusion
Charts are an indispensable tool in the modern application developer's repertoire, and JavaFX 20 brings an impressive array of capabilities into your hands. By following the steps outlined in this post, you're now equipped to implement and customize JavaFX charts effectively, thereby unlocking their full potential.
Effective data visualization is key in turning raw data into actionable insights. JavaFX charts, with their rich feature set, provide a powerful avenue to achieve just this within Java applications, creating visual narratives that can inform and engage users.
For more extensive charting needs or complex datasets, third-party libraries like JFreeChart or commercial options like TeeChart for Java also offer integration with JavaFX.
Dive into the JavaFX Documentation to explore the full capabilities of JavaFX charts, and start incorporating these visually engaging elements into your Java applications today.