Mastering Java EE 8 Tests: Avoid the Common Pitfalls!
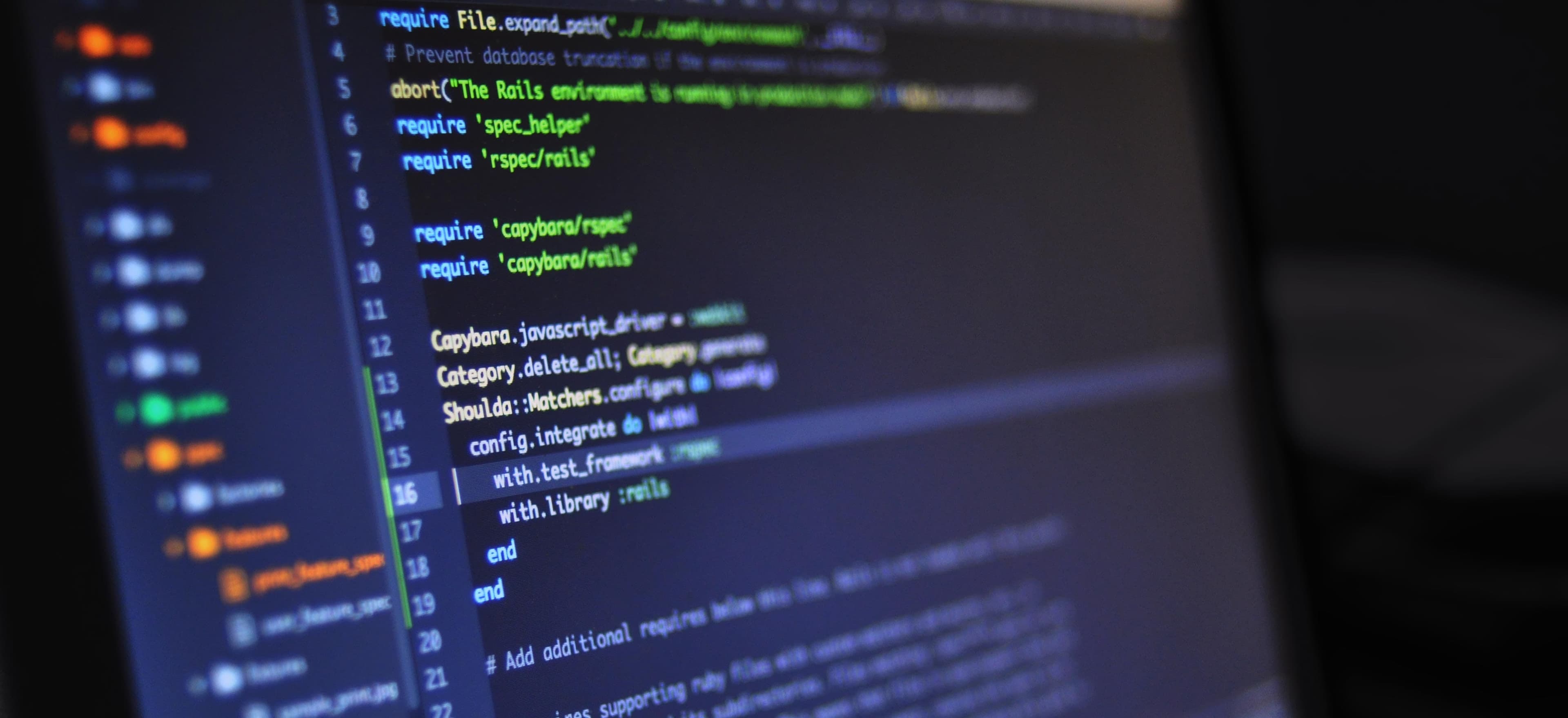
- Published on
Mastering Java EE 8 Tests: Avoid the Common Pitfalls!
Testing is an integral part of the software development process, where Java Enterprise Edition (Java EE 8) is no exception. As developers, we craft countless lines of code providing business logic and solving real-world problems. But how do we ensure that our Java EE 8 applications work as intended? Testing comes to the rescue! Here, we will delve into the common pitfalls while testing Java EE 8 applications and how to sidestep them to produce reliable, robust software.
Start With the Basics: Understanding Java EE 8 Testing Scope
Java EE 8 brings a plethora of specifications such as Contexts and Dependency Injection (CDI), Java Persistence API (JPA), Java API for RESTful Web Services (JAX-RS), and many more. Each comes with its quirks and aspects that you need to test.
Before you dive headfirst into coding, let's set a solid foundation:
Unit Testing: Tests individual components in isolation. Integration Testing: Ensures that different modules work together as intended. Functional Testing: Validates the system against functional requirements, akin to QA testing.
Understanding where each test type applies in Java EE will clear mist often clouding the testing landscape.
1. Embrace Mocks and Stubs in Unit Testing
Isolating units of code in Java EE can be tough due to dependencies on containers or resources.
Example:
Let's say we're testing a BookService
class that depends on an EntityManager
.
public class BookService {
@PersistenceContext
private EntityManager em;
public String createBook(Book book) {
em.persist(book);
return book.getId();
}
}
Testing this directly will lead to problems since EntityManager
is not available outside of a container. Enter mocks:
@Test
public void testCreateBook() {
EntityManager emMock = mock(EntityManager.class);
BookService bookService = new BookService();
setPrivateField(bookService, "em", emMock);
Book book = new Book();
book.setId("123");
String bookId = bookService.createBook(book);
verify(emMock).persist(book);
assertEquals("123", bookId);
}
Why this works:
- We're using a mocking framework (like Mockito) to mock the
EntityManager
. - The actual database is not hit during the test.
- The focus is strictly on the
createBook
method behavior.
In the code snippet, we use reflection to inject the mock into the private field, simulating the CDI that happens in the container, thus isolating the test from the container lifecycle. Make sure to check out Mockito for more advanced mocking techniques.
2. Integration Testing: The Real Deal
Java EE applications often require an actual container to manage lifecycle, transactions, and resources. Tools like Arquillian shine here, allowing you to test your application in a real container environment.
Example:
Testing an EJB service with Arquillian.
@RunWith(Arquillian.class)
public class OrderServiceTest {
@EJB
private OrderService orderService;
@Deployment
public static JavaArchive createDeployment() {
// Create deployable archive
}
@Test
public void testOrderCreation() {
Order order = new Order();
orderService.createOrder(order);
assertNotNull(order.getId());
}
}
Why this works:
- Arquillian manages the lifecycle of the container.
- Tests are executed inside a container, making sure that container-specific behaviors are taken into account.
- Dependencies such as EJBs or CDI Beans are injected as they would be in production.
Always ensure you're running integration tests in an environment close to your production setup.
3. Avoid the Overuse of Integration Tests
It's tempting to rely heavily on integration tests because they simulate the production environment more closely. However, they can be slow and resource-intensive.
Key Insight: Balance is crucial. Focus on unit tests for logic-heavy components and use integration tests sparingly for features heavily depending on container services.
4. Harness Functional Tests for User Perspective Assurance
For Java EE 8 applications, functional testing often involves UI testing or REST API testing, which can be done with tools like Selenium for web UIs or REST-assured for RESTful services.
Example:
Testing a RESTful service using REST-assured.
@Test
public void whenGetAllBooks_thenOK(){
RestAssured.get("/api/books")
.then()
.statusCode(HttpStatus.SC_OK);
}
Why this works:
- REST-assured provides a fluent interface to test HTTP-based REST services.
- It can be easily integrated with existing JUnit tests.
- The example above asserts that the response from the server is OK (HTTP 200), which simulates how the service would respond to a real API call.
Functional tests provide confidence that the application works from the user's perspective but don't replace the need for lower-level tests.
Common Pitfalls and How to Avoid Them:
- Ignoring Edge Cases: Make sure to test boundary conditions, invalid inputs, or unexpected user behaviors.
- Testing Only the Happy Path: Test how the application behaves under failure scenarios (e.g., DB connection drops).
- Not Mocking External Services: Avoid dependencies on external services in your tests. Use mocks or stubs for consistency and speed.
- Using Production Data for Testing: Use a controlled set of test data to avoid flaky tests and potential legal issues.
Conclusion: Mastering the Art of Testing in Java EE 8
By recognizing the strengths and weaknesses of different types of tests and understanding how to efficiently leverage them, you can achieve a robust and reliable Java EE 8 application. Combine unit, integration, and functional tests to cover your codebase effectively. Remember to focus on the 'why' of your tests, just as much as the 'how'.
By steering clear of common pitfalls and continually refining your testing strategies, you solidify your mastery over Java EE 8 testing. Your future self will thank you when changes can be made confidently, bugs are squashed early, and your deployment pipeline feels as smooth as silk.
Remember, testing is not just about finding bugs; it's about proving that your application does what it's supposed to do, each and every time. Happy coding and testing!
For further reading and deep dives into Java EE 8 testing strategies, refer to the official documentation and enrich your testing knowledge. Remember, a well-tested application is a reflection of its craftsman's diligence and care.