Cracking Composite IDs: Master Hibernate's Complexity!
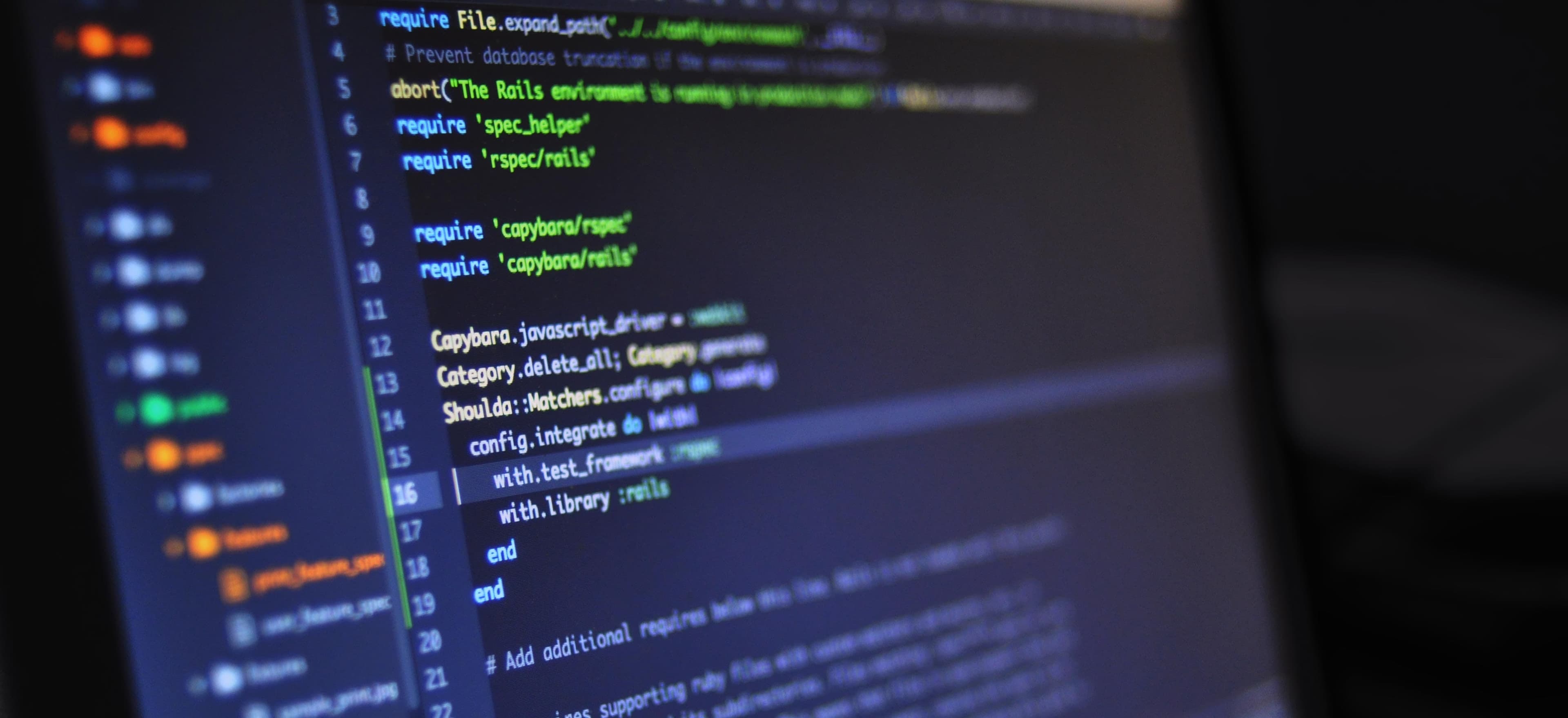
- Published on
Cracking Composite IDs: Master Hibernate's Complexity!
Persisting entities with composite primary keys in Hibernate can at times seem like a daunting task. For Java developers, it's a crucial skill to master, given the complexity and frequency of this operation in legacy systems and complex data models. This post will elucidate the strategies to efficiently map composite keys in Hibernate, ensuring your application's entities are robustly and correctly persisted.
Understanding Composite Primary Keys
In database design, a composite primary key is comprised of two or more columns which together uniquely identify a row in a table. It's often used when no single column can serve as a unique identifier or when the combination of multiple attributes is logically necessary to ensure uniqueness.
Hibernate, an Object-Relational Mapping (ORM) tool for Java, provides mechanisms to handle such complexity effortlessly, as long as you know the path to navigate its rich feature set.
Mapping Composite IDs Using @Embeddable
and @EmbeddedId
One common approach to dealing with composite keys in Hibernate is leveraging @Embeddable
and @EmbeddedId
annotations.
Consider an example where we have a Course
entity that requires a composite key made up of departmentId
and courseNumber
.
@Entity
@Table(name = "courses")
public class Course {
@EmbeddedId
private CoursePK id;
// Standard getters and setters
}
@Embeddable
public class CoursePK implements Serializable {
@Column(name = "department_id")
private Long departmentId;
@Column(name = "course_number")
private String courseNumber;
// Constructors, standard getters/setters, hashCode and equals
}
In this illustration, CoursePK
is marked with @Embeddable
, indicating that it's an embedded ID that will be used within another entity. The Course
entity utilizes the @EmbeddedId
annotation to embed the composite key.
Why Use @Embeddable
and @EmbeddedId
?
This approach provides a neat abstraction by encapsulating the composite key in its own class. It simplifies entity classes, especially when you're dealing with multiple fields contributing to the primary key.
Moreover, by implementing Serializable
, we ensure that the CoursePK
class can be serialized, which is a requirement for composite keys in Hibernate.
Harnessing the Power of @IdClass
Another mechanism for defining composite keys is through the use of the @IdClass
annotation. This approach requires defining a separate class to represent the composite key but doesn't require it to be @Embeddable
.
Here's how you'd implement our Course
entity using @IdClass
:
@Entity
@Table(name = "courses")
@IdClass(CoursePK.class)
public class Course {
@Id
@Column(name = "department_id")
private Long departmentId;
@Id
@Column(name = "course_number")
private String courseNumber;
// Other attributes, getters and setters
}
public class CoursePK implements Serializable {
private Long departmentId;
private String courseNumber;
// Constructors, standard getters/setters, equals and hashCode
}
The Reasoning Behind @IdClass
This strategy enables developers to define composite keys directly within the entity class, making it more explicit and straightforward to understand. The @IdClass
annotation serves as a pointer to Hibernate, informing it of the class that represents the composite key.
Both @Embeddable
/@EmbeddedId
and @IdClass
approaches are valid and capable in their own right, and choosing between them is a matter of preference and specific project requirements.
Edge Cases: When Composite Keys Get Tricky
Composite keys are not without their pitfalls. Relationships with other entities, for instance, can get complicated when composite keys are involved.
Consider an Enrollment
entity that references the Course
entity.
@Entity
@Table(name = "enrollments")
public class Enrollment {
@EmbeddedId
private EnrollmentPK id;
@MapsId("courseId")
@JoinColumns({
@JoinColumn(name = "department_id", referencedColumnName = "department_id"),
@JoinColumn(name = "course_number", referencedColumnName = "course_number")
})
@ManyToOne
private Course course;
// Other code omitted for brevity
}
@Embeddable
public class EnrollmentPK implements Serializable {
private CoursePK courseId; // Corresponds to the composite ID in the Course entity
private Long studentId; // Additional field specific to the Enrollment entity
// Constructors, standard getters/setters, equals and hashCode
}
Here, @MapsId
is used to indicate the attribute within the composite key that is linked to the Course
entity. The @JoinColumns
annotation specifies the mappings between the columns of the Enrollment
and Course
entities.
Navigating the Relationships
Working with entity relationships and composite keys involves careful organization of annotations and making sure the mappings correspond accurately between the entities. This ensures the integrity of the foreign key relationships and the seamless propagation of the entity state changes through Hibernate's session context.
Best Practices with Composite Keys in Hibernate
To mitigate common issues when dealing with composite keys in Hibernate, consider these best practices:
-
Keep composite keys simple: Try to limit the number of fields in a composite key. Complex keys increase the risk of errors.
-
Implement
equals()
andhashCode()
: For classes representing composite keys, it's crucial to provide proper implementations ofequals()
andhashCode()
methods to ensure Hibernate can correctly identify unique instances. -
Test rigorously: Given the nuanced nature of mapping composite keys, thoroughly test repository operations like
find
,save
, anddelete
to confirm that Hibernate handles the keys as expected. -
Clear documentation: Always document your entity mappings and explain why a composite key is used. This practice benefits maintainers and new developers who onboard to the project.
Hibernate and Composite Keys: Embrace the Detail
Mastering Hibernate's handling of composite keys requires patience and attention to detail. Understanding the purpose of @Embeddable
, @EmbeddedId
, and @IdClass
annotations, as well as the intricacies of entity relationships, is pivotal to utilizing the full potential of Hibernate.
Whether you're maintaining a legacy data model or designing a complex new system, with the insights from this post, you are better prepared to tackle the challenges of mapping composite keys in Hibernate. Remember that the key to success with composite IDs lies in appreciating the finer details of ORM persistence.
Further Reading
Delve deeper into Hibernate's features and configurations with these resources:
- Hibernate Documentation
- Java Persistence with Hibernate
- Thoughts on Object Relational Mapping
Happy coding, and may your persistence layer be as robust as it's ever been!