Ensuring Uptime: Mastering High Availability for Web Apps
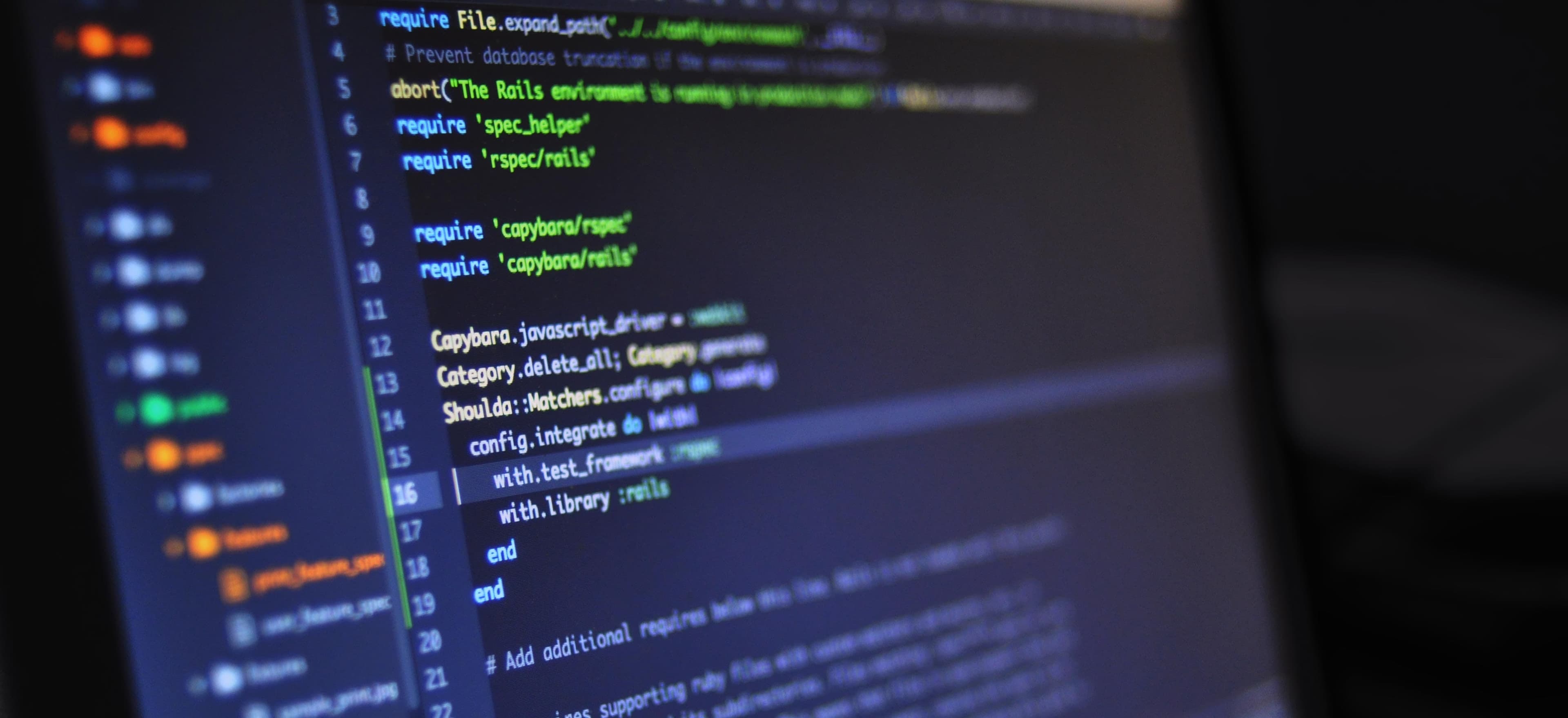
- Published on
Ensuring Uptime: Mastering High Availability for Web Apps with Java
High availability is the gold standard for web applications, especially in a world where even a minute of downtime can mean lost revenue and a tarnished reputation. By understanding and implementing high availability principles within your Java applications, you can ensure that your service remains accessible and reliable, regardless of unforeseen challenges.
What is High Availability?
High availability (HA) refers to a system’s ability to remain operational and accessible for a prolonged period of time, minimizing downtime to a bare minimum. It's not a luxury—it's a necessity in the always-on digital market. HA systems typically aim for 99.999% uptime, which translates to about 5 minutes of downtime per year.
Achieving High Availability in Java Web Applications
Architecture is Key
Before diving into code, it’s vital to design an architecture that supports HA. A typical HA setup involves:
- Redundancy: Have multiple instances of your web application running in parallel.
- Load Balancing: Distribute incoming traffic across these instances, using tools like Nginx or hardware load balancers.
- Failover Strategies: Prepare for the worst-case scenario where, if one instance fails, others can smoothly take over.
- Data Replication: Use databases that support automatic replication across multiple nodes, like MySQL with Group Replication.
Consider the Cloud
Cloud platforms like AWS, Google Cloud Platform, and Azure provide managed services that make implementing HA easier. Auto-scaling, managed databases, and global content delivery networks (CDNs) can all contribute to an HA ecosystem.
The Devil is in the Details: Java-Specific Considerations
Java applications, like their counterparts in other programming languages, rely on the infrastructure and architectural strategies mentioned above. However, there are some coding practices and frameworks in the Java ecosystem that you should use to your advantage.
public class HealthCheckServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException {
// Perform a series of quick health checks (database connection, dependent services, etc.)
boolean isHealthy = performHealthChecks();
if (isHealthy) {
// Return HTTP 200 to indicate service is healthy
resp.setStatus(HttpServletResponse.SC_OK);
resp.getWriter().write("All systems go!");
} else {
// Return HTTP 503 to indicate service is not healthy
resp.setStatus(HttpServletResponse.SC_SERVICE_UNAVAILABLE);
resp.getWriter().write("Service unavailable!");
}
}
private boolean performHealthChecks() {
// Example checks (should be replaced with real checks)
boolean dbConnected = checkDatabaseConnection();
boolean dependentServicesRunning = checkDependentServices();
return dbConnected && dependentServicesRunning;
}
// Placeholder method for checking database connections
private boolean checkDatabaseConnection() {
// Implement your database connection check logic here
return true; // assume it's always true for this example
}
// Placeholder method for checking dependent services
private boolean checkDependentServices() {
// Implement your dependent services check logic here
return true; // assume it's always true for this example
}
}
Why this works: Load balancers perform health checks on your instances. This servlet responds to these checks, which is crucial for routing traffic away from instances that are not performing optimally.
Frameworks and Libraries
Spring Boot
Spring Boot offers built-in support for building resilient applications. With annotations such as @RestController
, @RequestMapping
, and @Service
, you can declaratively set up your web services and integrate health check endpoints provided by Spring Boot Actuator.
Resilience4j
For circuit-breaking, rate limiting, and retry logic, check out Resilience4j. This lightweight fault tolerance library takes inspiration from Netflix’s Hystrix but shines with its simplicity and a Java 8 functional style of programming.
CircuitBreakerConfig circuitBreakerConfig = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.ringBufferSizeInHalfOpenState(2)
.ringBufferSizeInClosedState(2)
.build();
CircuitBreakerRegistry circuitBreakerRegistry = CircuitBreakerRegistry.of(circuitBreakerConfig);
CircuitBreaker circuitBreaker = circuitBreakerRegistry.circuitBreaker("myCircuitBreaker");
Supplier<String> decoratedSupplier = CircuitBreaker
.decorateSupplier(circuitBreaker, this::remoteCall);
String result = Try.ofSupplier(decoratedSupplier)
.recover(throwable -> "Hello from Recovery").get();
// The 'remoteCall' method should perform any remote call that needs circuit breaking
public String remoteCall() {
// Remote call logic
return "Hello from remote call";
}
Why this works: The snippet demonstrates how to set up a Circuit Breaker with Resilience4j, ensuring your application can withstand failures in external services it depends upon without cascading the issue to your entire application.
Testing for Failure
The only way to trust your HA setup is to test it thoroughly. Chaos engineering practices, championed by tools such as Chaos Monkey, advocate for deliberately introducing faults in order to test resilience.
Monitoring and Alerting
Remember, visibility is as essential as the HA features themselves. Leverage application performance monitoring (APM) tools like New Relic, Datadog, or Dynatrace that integrate well with Java applications, to stay ahead of potential issues.
Conclusion
Building highly available Java web applications may seem daunting, but with careful architecture planning, leveraging the right frameworks, and meticulous testing, it's within reach. Implement HA strategies, write resilient code with effective error handling, make judicious use of cloud resources and 3rd party tools, and monitor your application's performance relentlessly.
Uptime isn’t just about keeping the lights on—it’s about ensuring consistent, reliable service for your users. So put these principles into practice, and watch your Java web applications thrive with the robustness they—and your users—deserve.