Cracking Java Exception Handling with AspectJ!
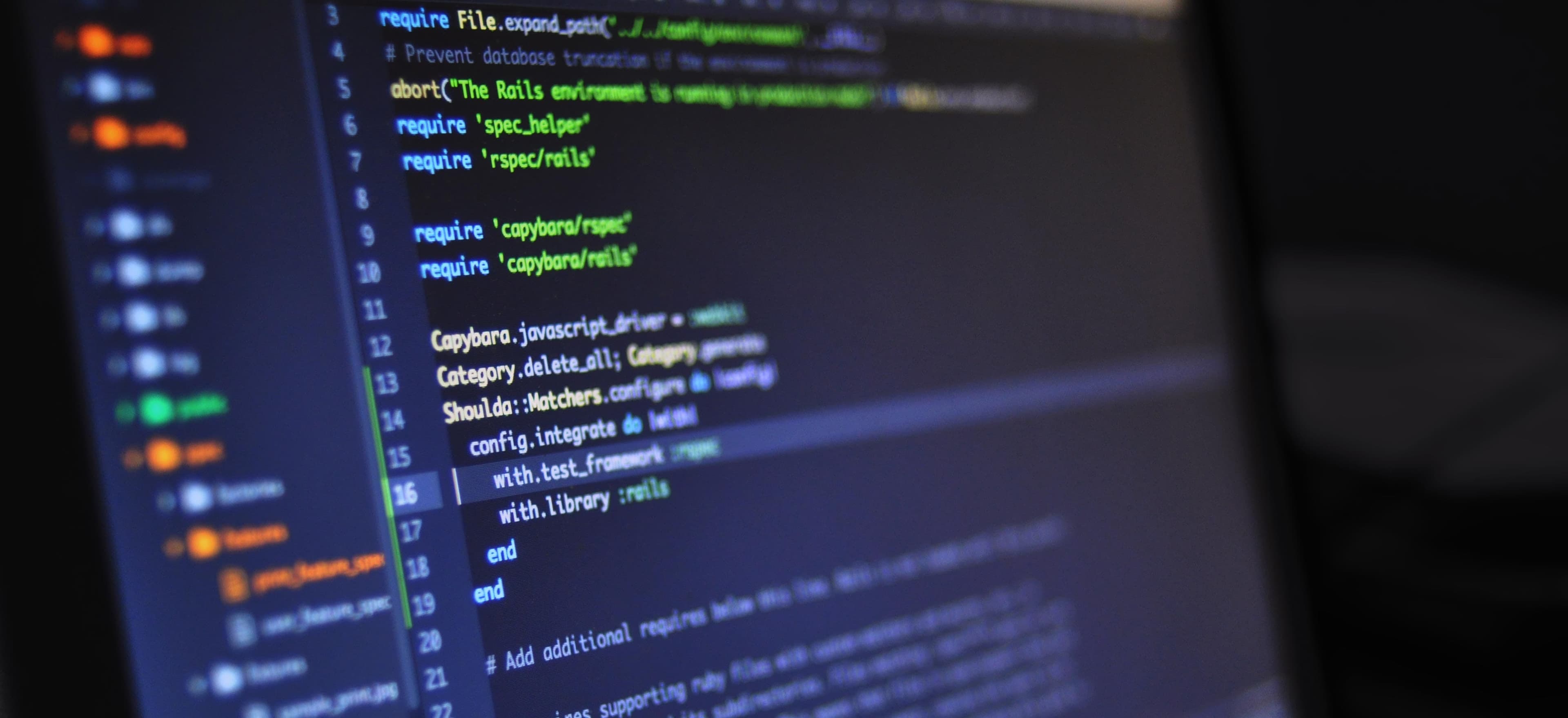
- Published on
Cracking Java Exception Handling with Aspect-Oriented Programming Using AspectJ
Exception handling is a vital part of software development, especially in Java, where the robust exception mechanism is a cornerstone of writing resilient, fault-tolerant applications. However, sometimes handling exceptions can become repetitive and scattered across the codebase. This is where Aspect-Oriented Programming (AOP) and AspectJ come to the rescue, helping streamline exception handling. Let's break down how you can leverage AspectJ to enhance your Java exception handling practices.
Understanding AspectJ and AOP
Before we dive into the intricacies of exception management, let's quickly recap what AOP is. Aspect-Oriented Programming is a programming paradigm that complements Object-Oriented Programming (OOP) by allowing cross-cutting concerns to be modularized into special classes called aspects. These concerns include logging, security, and, of course, exception handling.
AspectJ is the de-facto standard for AOP in Java. It extends Java with new constructs for writing aspects. Integrating AspectJ into your Java project can be straightforward, and it typically involves adding the AspectJ compiler and possibly an AOP framework like Spring AOP.
Prerequisites
To follow along, you need a basic understanding of Java and familiarity with its exception handling mechanisms. Also, you should have AspectJ set up in your development environment. You can find resources on how to do that here.
Installing AspectJ
For a Maven-based project, the following dependency in your pom.xml
will do:
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>LATEST_VERSION</version>
</dependency>
Replace LATEST_VERSION
with the current version of AspectJ, which you can find on the Maven Central Repository.
Writing an Aspect for Exception Handling
Assume you have various methods scattered across services that throw an IOException
, and you want to handle it in a standard manner. Classical Java would have you wrap each method call with a try-catch block, which quickly turns verbose. With AspectJ, you create an aspect.
import java.io.IOException;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.AfterThrowing;
@Aspect
public class IOExceptionHandlerAspect {
@AfterThrowing(
pointcut = "execution(* com.example.service..*(..))",
throwing = "ex"
)
public void handleIOException(IOException ex) {
// Standard exception handling code
System.err.println("An IO exception occurred: " + ex.getMessage());
// You might implement notification logic or error reporting here...
}
}
What's happening in the code above?
- The
@Aspect
annotation marks the class as an aspect. @AfterThrowing
is an advice, a special type of method that runs at certain points in the program execution. In this case, the advice runs after an exception is thrown (AfterThrowing
advice).- The
pointcut
expression,execution(* com.example.service..*(..))
, tells AspectJ to target all methods in thecom.example.service
package (and any subpackages). You can customize this to pinpoint exactly where you want your exception handling to apply. throwing = "ex"
binds the thrown exception to theex
parameter.
This aspect will catch any IOException
thrown by any method in specified packages and handle it with the logic you provide. It's modular and easily reusable.
Reacting to Different Exception Types
Let's say you want distinct handling for IOExceptions
, SQLExceptions
, and other unchecked exceptions. AspectJ can cater to that too!
@Aspect
public class GeneralExceptionHandlerAspect {
@AfterThrowing(
pointcut = "execution(* com.example..*(..)) && args(..,ex)",
throwing = "ex"
)
public void handleSqlException(SQLException ex) {
// Handle SQL exceptions specifically
}
@AfterThrowing(
pointcut = "execution(* com.example..*(..)) && args(..,ex)",
throwing = "ex"
)
public void handleIOException(IOException ex) {
// Handle IO exceptions separately
}
@AfterThrowing(
pointcut = "execution(* com.example..*(..)) && args(..,ex)",
throwing = "ex"
)
public void handleOtherExceptions(Exception ex) {
// A generic exception handler
if (!(ex instanceof IOException) && !(ex instanceof SQLException)) {
// handle unchecked exceptions
}
}
}
In this example, we have three advice methods tailored to the exception type. They all use the same pointcut but are differentiated by the parameter type they bind the exception to.
Advantages of Using AspectJ for Exception Handling
- Reduced Boilerplate: AspectJ significantly decreases the boilerplate related to exception handling in your services.
- Consistency: Ensures that exception handling is consistent across the application.
- Separation of Concerns: Decouples the business logic from exception handling code, leading to cleaner and more maintainable code.
- Centralized Management: Allows for centralized management of cross-cutting concerns such as logging and transaction management in addition to exception handling.
Testing Your Aspect
To verify that your aspect is working as intended, you can write unit tests that simulate methods throwing the exceptions your aspect is supposed to handle. Ensure that your test methods align with the pointcut expressions in your aspect:
class IOExceptionService {
public void methodThrowingIOException() throws IOException {
throw new IOException("This is a test exception.");
}
}
class IOExceptionHandlerAspectTest {
private final IOExceptionHandlerAspect aspect = new IOExceptionHandlerAspect();
@Test(expected = IOException.class)
public void shouldHandleIOException() throws IOException {
IOExceptionService service = new IOExceptionService();
// Simulate method that would throw an IOException
try {
service.methodThrowingIOException();
} catch (IOException e) {
// Handle exception using the aspect
aspect.handleIOException(e);
throw e; // Rethrow to verify if the exception was handled or not
}
}
}
In a real scenario, you might also want to mock other components that your aspect interacts with, such as logging or monitoring services.
Conclusion
Exception handling is an essential aspect of robust Java applications, but it can become cumbersome and repetitive. AspectJ provides a powerful tool to address these pain points, encouraging cleaner, more maintainable code by extracting exception handling into reusable aspects. The examples above illustrate just a fragment of what's possible with AspectJ when it comes to managing exceptions or any cross-cutting concerns your applications may have.
By implementing these strategies, you can ensure that your application's exception handling is not only consistent but also easier to understand and modify. This aligns with modern best practices that favor separation of concerns and modular design.
Start applying AspectJ to your Java projects today, and you might find that exception handling is no longer the tedious task it once was. Happy coding!
Resources:
- AspectJ Documentation: https://www.eclipse.org/aspectj/docs.php
- Maven Central Repository for AspectJ: https://search.maven.org/search?q=g:org.aspectj
- AspectJ in Action by Ramnivas Laddad (for an in-depth understanding of AspectJ in real-world applications)