Mastering Spring Boot: Fixing Web Slice Test Flaws
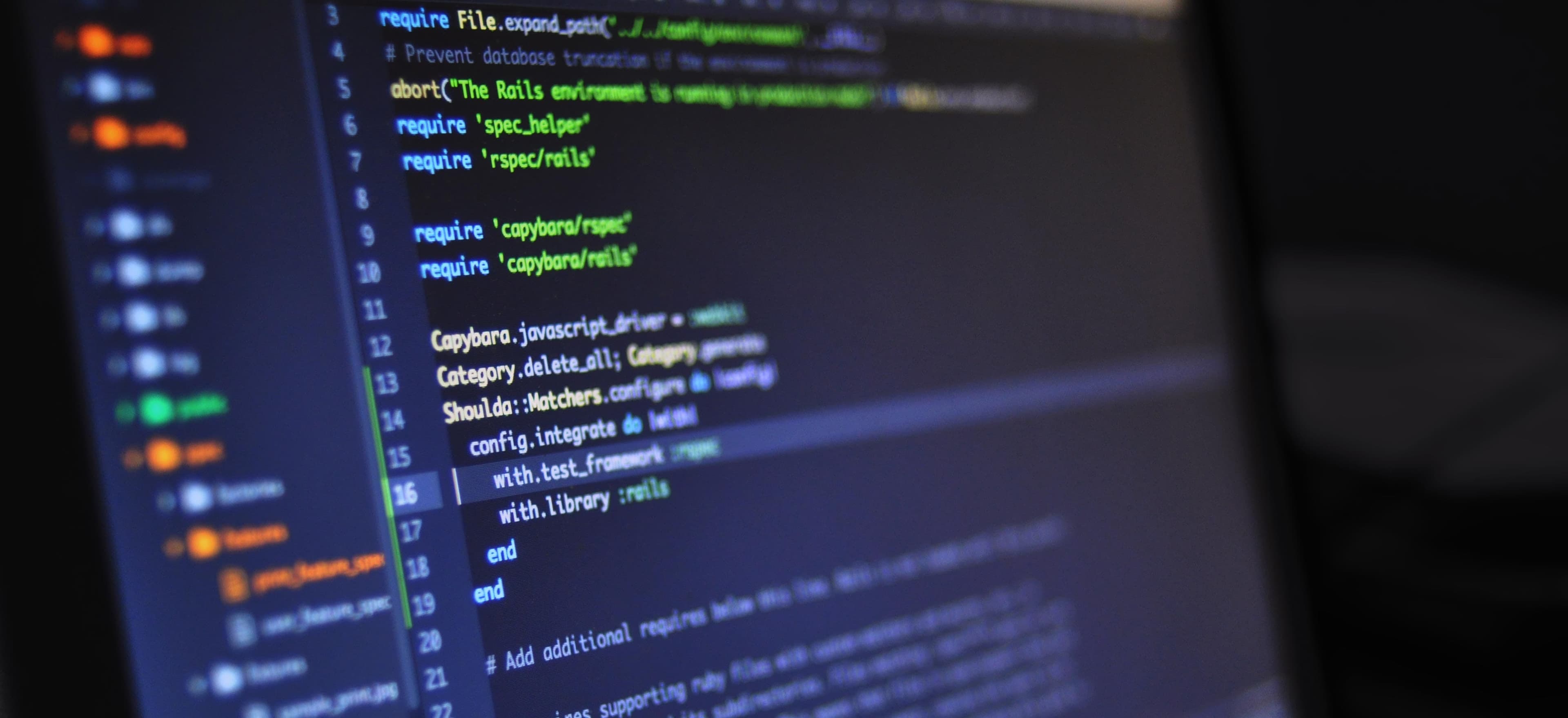
- Published on
Mastering Spring Boot: Fixing Web Slice Test Flaws
In the world of Java development, Spring Boot has emerged as the go-to framework for creating stand-alone, production-grade Spring-based Applications with ease. However, even with Spring Boot streamlining application development, writing comprehensive and effective tests remains a critical task for developers. In this article, we'll explore the common pitfalls of Spring Boot's Web Slice tests and provide you with strategies to fix them, ensuring your web applications are as robust as possible.
Understanding Spring Boot Test Slices
Before diving into the details of improving Web Slice tests, it's important to appreciate what test slices are. In essence, the concept of "slices" is introduced by Spring Boot to provide a way to test specific layers of your application in isolation. Among them, Web Slice tests are designed to focus on the web layer, validating the behavior of controllers and associated web infrastructure without starting the entire context.
By default, the Web Slice available in Spring Boot is @WebMvcTest
. This slice auto-configures the Spring MVC infrastructure for your tests and limits the application context to only the necessary beans for MVC testing, leading to faster test execution.
However, Web Slice tests can sometimes give a false sense of security if not set up correctly. Issues such as context misconfiguration or ignoring certain bean dependencies can lead to an application that passes tests but fails in production.
Common Flaws in Web Slice Tests
Here are a few flaws that can occur when using @WebMvcTest
:
- Limited context: While isolating the web layer speeds up tests, it can also hide issues that may arise when the full application is running.
- Bean dependencies: If your controller depends on certain beans that are not part of the MVC layer, these may be left out of the test configuration, leading to unexpected behavior.
- Configuration properties: Any configuration properties used in the controller or its dependencies may need to be included in the test setup to ensure accurate testing.
Here are examples of these issues and how you can fix them.
Example 1: Ensuring Complete Controller Dependencies
Imagine a controller that depends on a service class for some functionality. If the service class is not a part of the MVC layer, it won't be included in the @WebMvcTest
context by default.
@RestController
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/user/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
The Flaw
The UserController
needs an instance of UserService
. But if we only use @WebMvcTest(UserController.class)
, the test won't know about UserService
, and our test will fail to start the application context.
The Fix
We can fix this by providing a mock implementation of UserService
in our test.
@WebMvcTest(UserController.class)
class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
public void shouldReturnUserForGivenId() throws Exception {
when(userService.findById(1L)).thenReturn(new User("Alice"));
mockMvc.perform(get("/user/1")
.contentType(MediaType.APPLICATION_JSON))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("Alice"));
}
}
Why It Works
The @MockBean
annotation adds a mock version of UserService
to the Spring application context. This allows the UserController
to be autowired with a dummy implementation, ensuring that the MVC layer can be tested in isolation while still simulating its external dependencies.
Example 2: Incorporating Configuration Properties
If your controller relies on configuration properties to function, yet they remain unaccounted for in your tests, it can lead to failed tests or undetected bugs.
The Flaw
Suppose UserController
utilizes a configuration property to determine a feature toggle, like so:
@RestController
public class UserController {
@Value("${user.newFeatureEnabled}")
private boolean newFeatureEnabled;
// Other methods...
@GetMapping("/new-feature")
public ResponseEntity<String> getNewFeatureStatus() {
return ResponseEntity.ok(newFeatureEnabled ? "Enabled" : "Disabled");
}
}
The Fix
To test this controller accurately, we need to configure the test to include the relevant property.
@WebMvcTest(UserController.class)
@Import(UserController.class)
@PropertySource("classpath:application-test.properties")
class UserControllerTest {
// Existing test code...
}
Why It Works
The @PropertySource
annotation tells Spring to load the specified properties file when the test context is initialized. This allows us to set properties like user.newFeatureEnabled
which the controller needs to function correctly. Including the application-test.properties
file in the src/test/resources
directory can specify these properties with test-specific values.
user.newFeatureEnabled=true
With this setup, when the test context loads the controller, the correct properties will be used, increasing the test's reliability and relevance.
Conclusion
Mastering Web Slice tests in Spring Boot can be a challenge, but it's a task well worth undertaking. By recognizing common pitfalls and applying the fixes we've discussed, you can ensure that your tests remain reliable indicators of your application's health.
These strategies—populating the appropriate beans with @MockBean
, integrating property files with @PropertySource
, and other context configuration techniques—allow for granular validation of your web layer, leading to resilient, production-ready applications.
Moreover, good testing practices, such as the ones highlighted, are integral to successful continuous integration and deployment pipelines, which rely heavily on automated testing to maintain code quality.
Spring Boot is a powerful platform that, when correctly harnessed, can greatly increase your efficiency as a Java developer. By investing time into perfecting your testing strategy, especially around web slice tests, you can avoid costly mistakes and deliver high-quality software with confidence. Happy coding!
Want to dig deeper into Spring Boot testing? Refer to the official Spring Boot testing documentation for more in-depth insights and advanced techniques.