Java 7: Scaling Up with ConcurrentHashMap vs HashMap
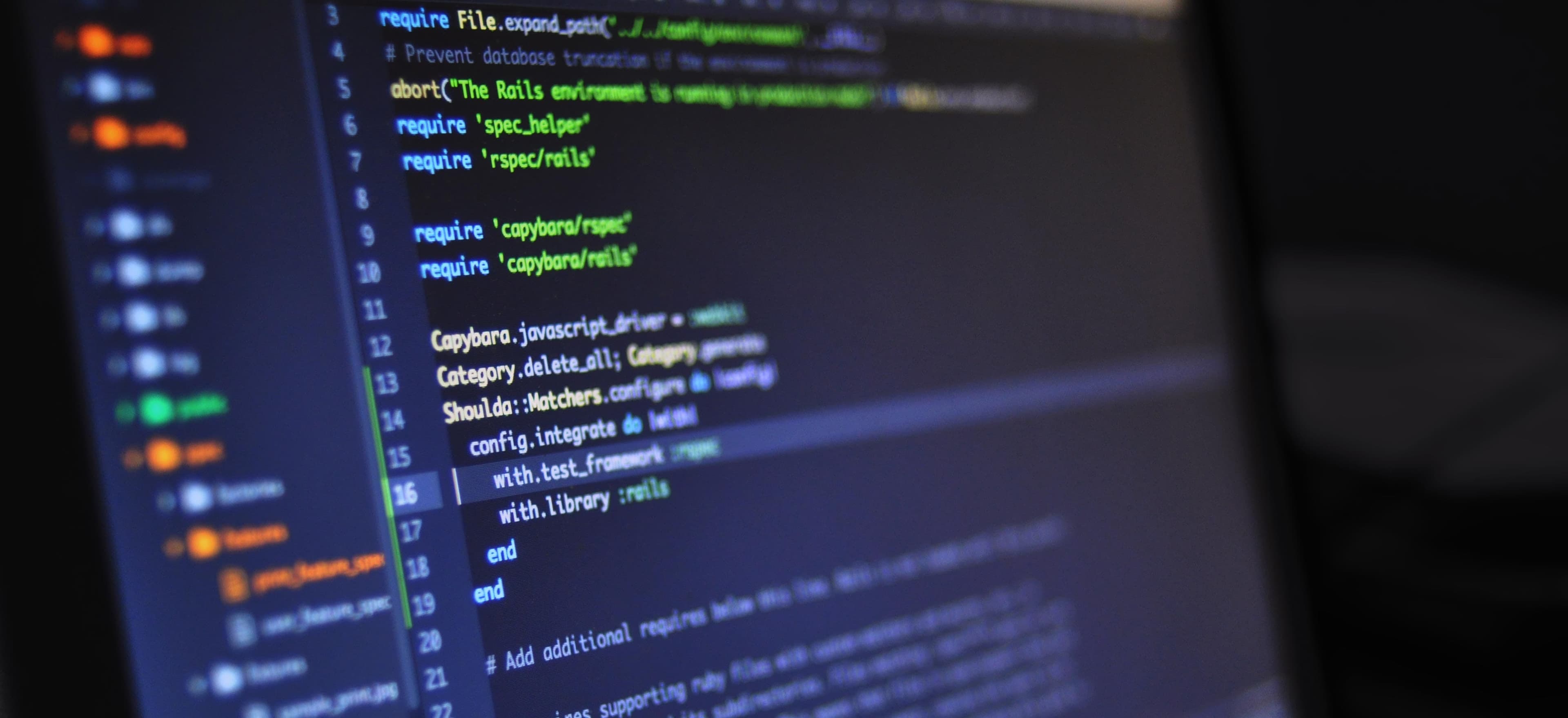
- Published on
Java 7: Scaling Up with ConcurrentHashMap vs. HashMap
In the realm of Java programming, the choice between ConcurrentHashMap
and HashMap
can be pivotal for applications requiring map data structures. With Java 7 and its ongoing revisions, understanding these two implementations becomes crucial, especially when concurrent operations and performance are at stake.
Understanding HashMap
Before delving into the muscle power of concurrency, let's first take a glimpse at the traditional HashMap
. A part of the Java Collections Framework, HashMap
is a map based on the hash table data structure. It allows us to store key-value pairs, where each key is unique.
Here's a basic example of HashMap
usage:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
// Creating a HashMap
HashMap<Integer, String> map = new HashMap<>();
// Adding key-value pairs
map.put(1, "Java");
map.put(2, "Python");
map.put(3, "C++");
// Displaying the content
System.out.println(map);
}
}
In the snippet above, we create a simple HashMap
and populate it with programming language names associated with an integer key. This is a straightforward way to maintain a collection of objects.
However, HashMap
falls short in one significant area - it is not thread-safe. In a concurrent environment where multiple threads may modify the map simultaneously, HashMap
can lead to data corruption. This is where ConcurrentHashMap
comes into play.
Scaling Up with ConcurrentHashMap
ConcurrentHashMap
is part of the java.util.concurrent
package and is designed to handle concurrency. It allows multiple readers without any synchronization and a restricted number of writers to modify the map safely.
Let's explore a ConcurrentHashMap
example:
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
// Creating a ConcurrentHashMap
ConcurrentHashMap<Integer, String> concurrentMap = new ConcurrentHashMap<>();
// Adding key-value pairs
concurrentMap.put(1, "Java");
concurrentMap.put(2, "Python");
concurrentMap.put(3, "C++");
// Displaying the content
System.out.println(concurrentMap);
}
}
At first glance, the code looks very similar to our HashMap
example. However, the magic happens behind the scenes. ConcurrentHashMap
employs several segments (controlled by the concurrency level) to store the data, which means it locks the segment to which the key belongs rather than the entire map.
Why Use ConcurrentHashMap?
- Thread-Safety: As discussed, this is the clear winner for concurrent applications.
- Performance: By allowing concurrent reads and limiting writes to a small section of the map,
ConcurrentHashMap
offers higher throughputs. - Fail-Safe Iterators: The iterators in
ConcurrentHashMap
do not throwConcurrentModificationException
if the map is structurally modified during iteration.
ConcurrentHashMap vs. HashMap Performance
In a single-threaded environment, HashMap
might perform slightly better due to its non-concurrent nature, which results in lower overhead. However, once you introduce concurrency, ConcurrentHashMap
will typically outperform HashMap
due to better scalability.
When to Pick ConcurrentHashMap over HashMap?
- When the application has multiple threads modifying or reading the map concurrently.
- When thread-safety guarantees are paramount for the application's integrity and correctness.
- In high-throughput scenarios where read operations far outnumber write operations.
Practical Considerations
Let's address practical considerations regarding HashMap
and ConcurrentHashMap
. Should you just replace all your HashMap
instances with ConcurrentHashMap
? Not really.
- If your application is single-threaded or if the map is confined within a thread,
HashMap
is the ideal choice due to its simplicity. - If you have a read-intensive application where the map is initialized once but read many times (e.g., a configuration map), you might want to use
Collections.unmodifiableMap()
over aHashMap
, for a read-only, thread-safe collection. - When using
ConcurrentHashMap
, tune your concurrency level. The default is 16, but this may not be optimal for all use cases.
For those interested in the granular details, here are some resourceful Java documentation links:
Conclusion
In the world of Java 7, understanding the distinction between ConcurrentHashMap
and HashMap
is vital for developers who value performance and thread-safety. The choice between the two should be dictated by the specific needs of the application. Don't just follow the concurrency trend blindly; know why you're choosing one over the other based on the nature of your tasks at hand.
While HashMap
continues to be a quick and easy data structure for key-value storage in non-concurrent environments, ConcurrentHashMap
emerges as a stalwart companion in applications prone to the simultaneous footsteps of multiple threads. Equip yourself with both, understand their strengths and limits, and let your application scale new heights in performance and reliability.
Happy coding, Java enthusiasts!