Cracking Geometric Brownian Motion in Java: A Deep Dive
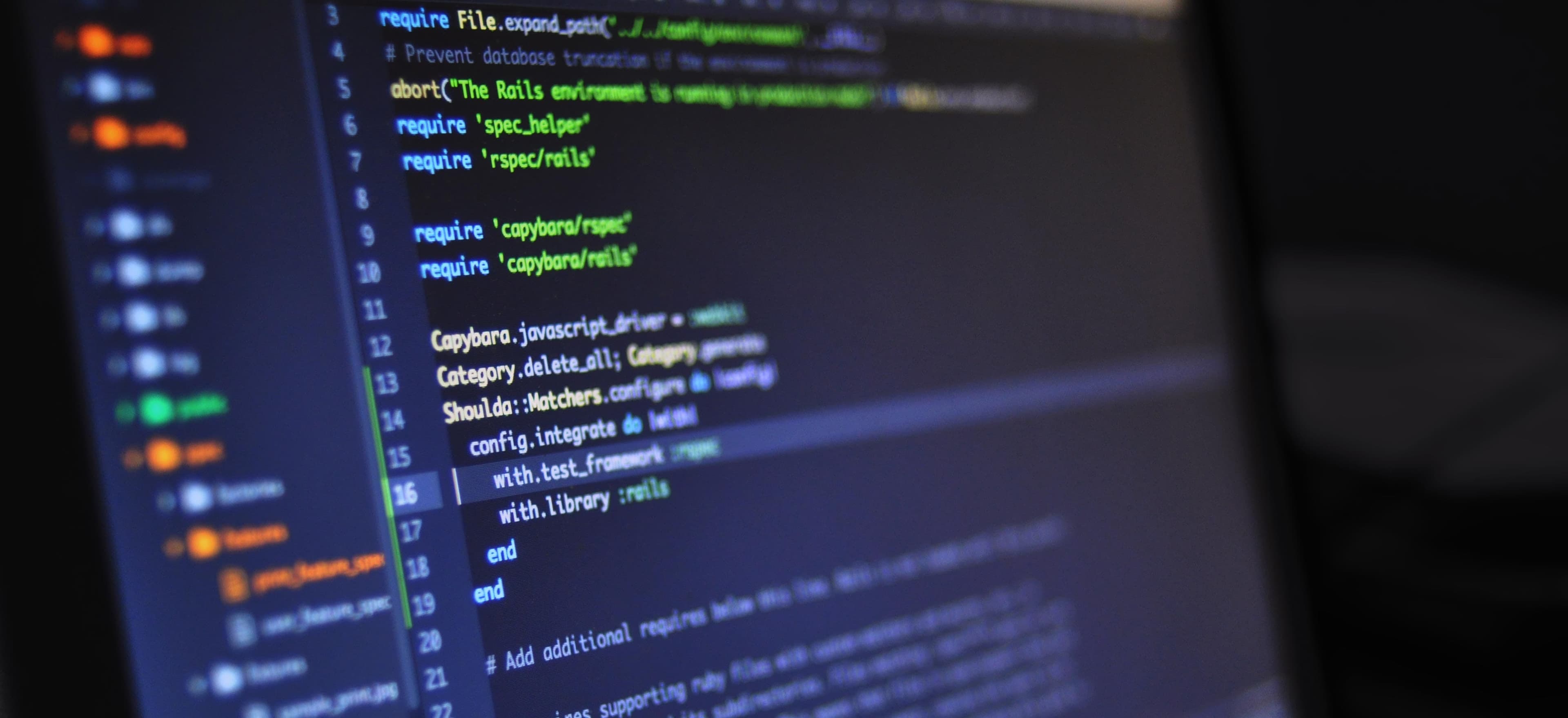
- Published on
Cracking Geometric Brownian Motion in Java: A Deep Dive
Geometric Brownian Motion (GBM) is a mathematical model that is widely used to forecast the price paths of stocks, commodities, or indices in the finance world. Understanding and implementing GBM can seem intimidating, but Java, with its robust mathematical libraries and straightforward syntax, makes it remarkably approachable. In this deep dive, we'll peel back the layers of Geometric Brownian Motion and reveal how to implement it proficiently in Java.
What is Geometric Brownian Motion?
Before diving into code, let’s understand the concept. GBM combines the principles of Brownian Motion (also known as Wiener Process) with geometric (exponential) scaling. It’s mathematically expressed as follows:
dS = μSdt + σSdB
where:
S
is the stock priceμ
is the expected returnσ
is the standard deviation of returns (volatility)dB
is the Brownian motion
GBM assumes that a stock's log returns follow a normal distribution, meaning that prices can be modeled to drift upwards or downwards (captured by μ
) while also incorporating random 'shocks' (captured by σSdB).
Implementing Geometric Brownian Motion in Java
Implementing GBM in Java involves using the formula to simulate future stock prices using random draws from a standard normal distribution. We will use Java’s Random
class for this purpose. Let's begin by implementing a simple but efficient GBM simulator.
Step 1: Import the Necessary Libraries
We start by importing Java's math and utility libraries; they include necessary classes like Random
for generating random numbers and Math
for exponential and logarithmic functions.
import java.util.Random;
import java.util.stream.DoubleStream;
Step 2: Define the GBM Function
We define a method simulateGBM
which will perform the simulation of GBM. This method will take the parameters necessary for the GBM (initial stock price S0
, drift mu
, volatility sigma
, time frame T
, and number of steps n
) as inputs.
public class GeometricBrownianMotion {
private static final Random random = new Random();
public static DoubleStream simulateGBM(double S0, double mu, double sigma, double T, int n) {
double dt = T/n;
double drift = (mu - 0.5 * Math.pow(sigma, 2)) * dt;
double volatility = sigma * Math.sqrt(dt);
// We are going to use Java 8 streams to generate our GBM path
return DoubleStream.iterate(S0, S -> S * Math.exp(drift + volatility * random.nextGaussian()));
}
// Other methods and the main program will go here
}
Why this code works:
iterate
is a method from theDoubleStream
class that allows us to create a sequence of doubles where each subsequent number is generated using the previous one - exactly what we need for stock price simulation over time.- We use
Math.exp
to simulate the exponential growth factor, which is part of the GBM model. random.nextGaussian()
returns a random number drawn from a normal distribution with a mean of 0 and a standard deviation of 1, which is multiplied by ourvolatility
factor to simulate the Brownian motion part of the GBM.
Step 3: Running the Simulation
Now, let’s use the method simulateGBM
and see how a hypothetical stock price behaves over time. We will assume some average values for illustration purposes.
public static void main(String[] args) {
final double S0 = 100; // Initial stock price
final double mu = 0.05; // Expected return
final double sigma = 0.2; // Volatility
final double T = 1; // Time period of 1 year
final int n = 250; // Number of steps (trading days in a year)
DoubleStream stockPrices = simulateGBM(S0, mu, sigma, T, n)
.limit(n); // This will limit our stream to `n` elements
stockPrices.forEach(price -> {
System.out.printf("%.2f%n", price);
});
}
When we run the main method, we will see a sequence of price outputs printed onto the console window that represent our stock's daily closing price over one year.
Why this part of the code is effective:
- It brings our
simulateGBM
function to life, allowing us to visualize how the simulated stock price changes over time. - By limiting the size of the stream to
n
, we ensure that our simulation runs for the intended number of steps.
Step 4: Analysis and Visualization (Optional)
While the console output gives us an idea about the behavior of the Geometric Brownian Motion, it's often more useful to analyze these results visually. Plotting these simulated price paths using tools such as JFreeChart or integrating with a front-end JavaScript charting library can provide better insights into the price dynamics.
// Code for visualization would go here - this is optional and depending on the tools and libraries you are comfortable with.
Note: Visualization is outside the scope of this article, so we won’t go into the details here.
Conclusion
Implementing the Geometric Brownian Motion model in Java is straightforward and efficient. With basic Java knowledge and a fundamental understanding of GBM, one can model future stock prices and conduct financial analysis or risk management tasks.
Java's power for mathematical computation combined with its easy-to-understand syntax makes it an ideal choice for tackling complex problems such as GBM simulations. Whether you're a finance professional, a software developer, or a student, mastering GBM in Java can elevate your analytical capabilities and open doors to sophisticated financial modeling.
Always remember to assess the assumptions of the model and to consider the practical limitations and potential improvements, such as incorporating jumps or mean-reversion, which can make the model more representative of reality.
If you’re eager to explore more about the capabilities of Java in financial engineering, you might find resources on stochastic calculus and the applications of Monte Carlo simulations in finance to be similarly engaging and fruitful.
Happy coding, and may your simulations forecast prosperity!
(Please note that this blog post is intended for educational purposes and is not financial advice. The Geometric Brownian Motion model is a simplified representation of stock price dynamics and does not consider many factors that can influence financial markets.)
Checkout our other articles