Streamline Your Release: Auto-Promote Artifacts to Maven!
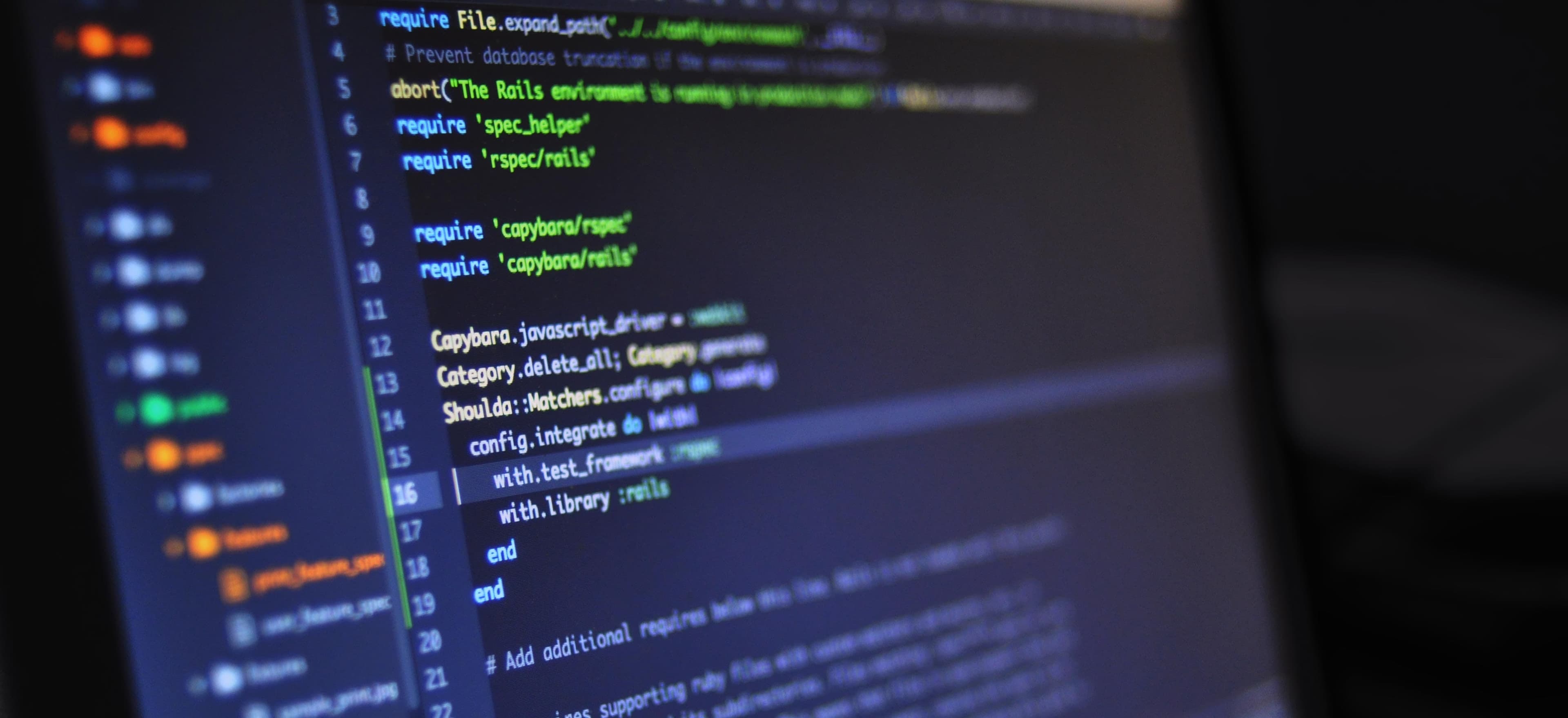
- Published on
Streamline Your Release: Auto-Promote Artifacts to Maven Central
Developing Java applications can be as exhilarating as it is intricate, with Maven Central standing as the lighthouse for dependency management. Every Java developer negotiates with Maven Central's repositories, whether to fetch the finest libraries for a project or to contribute one's own artifact. Have you ever stumbled upon the question of how to automate the promotion of your artifacts to Maven Central? It's a game-changer for efficiency, and this post will elaborate on mastering this craft.
Maven Central - The Hub of Java Libraries
Before we delve deeper, let’s understand what Maven Central is. Owned by Sonatype, Maven Central is the default repository for Apache Maven to locate Java libraries and various project dependencies. It hosts a colossal array of artifacts that makes it the go-to source for developers.
The Manual Struggle
Typically, releasing a new version of an artifact to Maven Central can be quite a task. It involves several manual steps:
- Generating the artifacts.
- Signing them with GPG.
- Uploading them to the Sonatype OSSRH (OSS Repository Hosting).
- Staging the repository.
- Promoting or releasing the staged repository.
For a one-time affair, these steps may not seem burdensome. However, frequent releases can turn this process into a recurring nightmare.
Automation To The Rescue
Let's automate this grind. By the end of this process not only will your releases be quicker, but you'll also minimize the risk of human error.
Prerequisites
Before diving in, ensure you have the following:
- Apache Maven installed and configured.
- An account on Sonatype OSSRH.
- GPG set up with a key pair generated for signing artifacts.
- Maven settings configured with OSSRH credentials and GPG passphrase.
Setting Up Your Pom.xml
Your pom.xml
is the backbone of Maven automation. It dictates how Maven should package, test, and deploy your application. Let's set it up for auto-promotion.
<project>
...
<distributionManagement>
<snapshotRepository>
<id>ossrh</id>
<url>https://s01.oss.sonatype.org/content/repositories/snapshots/</url>
</snapshotRepository>
<repository>
<id>ossrh</id>
<url>https://s01.oss.sonatype.org/service/local/staging/deploy/maven2/</url>
</repository>
</distributionManagement>
<profiles>
<profile>
<id>release</id>
<build>
<plugins>
<plugin>
<groupId>org.sonatype.plugins</groupId>
<artifactId>nexus-staging-maven-plugin</artifactId>
<version>1.6.8</version>
<extensions>true</extensions>
<configuration>
<serverId>ossrh</serverId>
<nexusUrl>https://s01.oss.sonatype.org/</nexusUrl>
<autoReleaseAfterClose>true</autoReleaseAfterClose>
</configuration>
</plugin>
</plugins>
</build>
</profile>
</profiles>
...
</project>
In the above snippet, we're setting the URLs for snapshot and release repositories. Moreover, we're adding the nexus-staging-maven-plugin
under the release
profile, configuring it to auto-release after the repository is closed.
Releasing with Maven
Once your pom.xml
is prepared, invoke the release process with Maven goals:
mvn clean deploy -P release
This command will compile, test, package, sign and deploy your code. The -P release
option activates the release
profile, which in turn triggers the nexus-staging-maven-plugin
to do its magic.
Why Automate With the Nexus Staging Maven Plugin?
You may wonder why we use the nexus-staging-maven-plugin
. This plugin interacts with Nexus OSSRH directly, handling the staging and promotion in one sweep, controlled by the autoReleaseAfterClose
configuration setting. It spares you the manual intervention of promoting the staging repository.
Essential Tips for Reliable Auto-Promotion
- Automation is a Double-Edged Sword: Automation speeds up repetitive tasks but be wary of overreliance. Always ensure to have proper checks like test coverage to catch any issues early.
- Back Up Your GPG Keys: A lost GPG key can be detrimental. Keep it secure and backed up.
- Keep Your OSSRH Credentials Safe: Your Sonatype credentials are vital. Store them in your Maven’s
settings.xml
and guard that file. - Tag Your Releases: Maintain a git tag for each release. It provides a clear history and makes debugging of version-specific issues less vexing.
Conclusion
Automating artifact promotion to Maven Central clears a pathway for streamlined development and deployment processes. It reflects professionalism and attention to efficiency in your development cycle.
In this post, we’ve traversed the manual struggle of artifact release and the subsequent relief brought by automation using the nexus-staging-maven-plugin
. We’ve peeked at pom.xml
configurations and delved into Maven goals that tie together a seamless release process, reinforcing it with robust tips for an unfailing auto-promotion strategy.
For those keen to delve deeper, the documentation on Maven, Nexus Repository Manager, and GPG offer valuable resources. Remember, the investment in setting up this automation pays dividends in the long run, freeing up precious time for creative coding endeavors and innovation.
Happy coding and auto-promoting!