Mastering Ignite & Spring on K8s: Deployment Woes
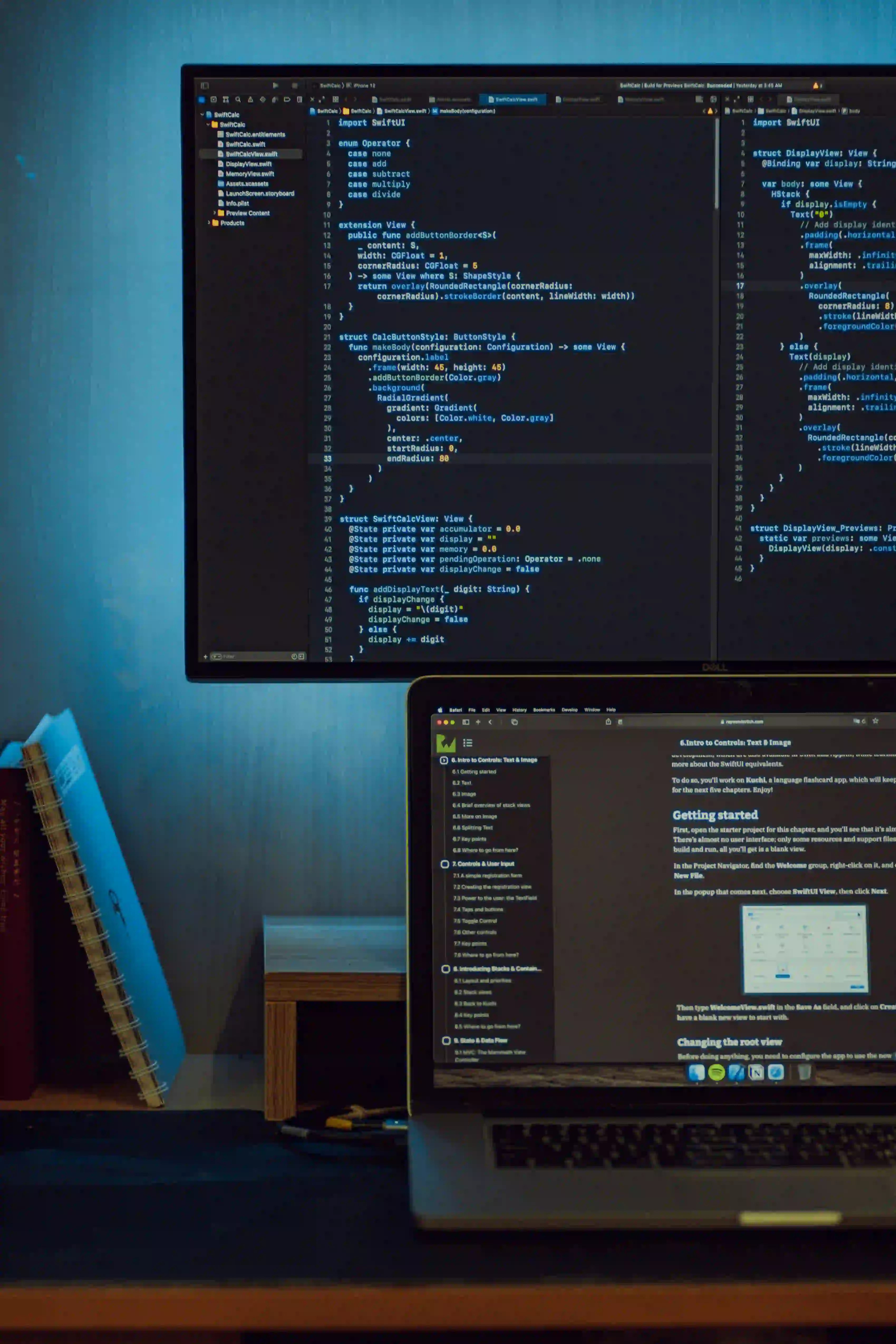
Mastering Ignite & Spring on Kubernetes: Navigating Deployment Challenges
When you blend the resilience of Apache Ignite with the versatility of Spring Framework and deploy it on the juggernaut of container orchestration, Kubernetes (K8s), you've got a recipe for an enterprise-level powerplant. However, achieving a smooth deployment of Ignite and Spring on Kubernetes can present some unique challenges. In this blog post, we'll explore strategies to navigate potential pitfalls and ensure your deployment is rock-solid.
Understanding the Components
First, let's break down the elements of our topic:
- Apache Ignite: A distributed database, caching, and processing platform designed for high-performance and scalability.
- Spring Framework: An application framework that provides comprehensive programming and configuration models for modern Java-based enterprise applications.
- Kubernetes (K8s): An open-source system for automating deployment, scaling, and management of containerized applications.
Combining these technologies allows developers to build high-availability systems that are both scalable and resilient. But as with any complex system, there are hurdles to overcome.
Containerizing Your Application
Before you can deploy anything to Kubernetes, you need to containerize your application. For Java applications built with Spring and Ignite, this typically involves creating a Dockerfile.
Here’s a simple Dockerfile to get you started:
FROM openjdk:11-jdk-slim as build
WORKDIR /workspace/app
COPY mvnw .
COPY .mvn .mvn
COPY pom.xml .
COPY src src
RUN ./mvnw install -DskipTests
FROM openjdk:11-jdk-slim
VOLUME /tmp
COPY --from=build /workspace/app/target/spring-ignite-app-0.0.1-SNAPSHOT.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
Why this Dockerfile works:
- We're using a multi-stage build to keep our final image lean.
- Using
openjdk:11-jdk-slim
takes advantage of a lightweight Java 11 runtime. - We're copying only the necessary files for the build, which keeps the context sent to the Docker daemon small.
Deployment Manifest for Kubernetes
Once your image is built and pushed to a container registry, you need a deployment manifest for K8s. The manifest tells Kubernetes how to run your application. Here’s a basic example to deploy a Spring Boot application with an Ignite node:
apiVersion: apps/v1
kind: Deployment
metadata:
name: spring-ignite-deployment
spec:
replicas: 3
selector:
matchLabels:
app: spring-ignite
template:
metadata:
labels:
app: spring-ignite
spec:
containers:
- name: spring-ignite
image: your-registry/spring-ignite-app:latest
ports:
- containerPort: 8080
env:
- name: SPRING_PROFILES_ACTIVE
value: "k8s"
Why this manifest works:
- It sets the deployment with three replicas, ensuring high availability.
- The
matchLabels
section helps Kubernetes identify which pods are part of this deployment. - The
containerPort
matches the port your Spring Boot application is set to run on.
Communication Between Ignite Nodes in Kubernetes
Apache Ignite relies on effective communication between its nodes to maintain a cluster. Kubernetes' dynamic nature makes static IP assignments impractical, so you'll want to use a service discovery mechanism that Ignite understands. One option is to use Kubernetes IP finder for this task.
Below is an example of how you can configure Ignite to use Kubernetes IP finder:
@Bean
public IgniteConfiguration igniteConfiguration() {
IgniteConfiguration cfg = new IgniteConfiguration();
TcpDiscoveryKubernetesIpFinder ipFinder = new TcpDiscoveryKubernetesIpFinder();
ipFinder.setNamespace("default");
ipFinder.setServiceName("ignite-service");
cfg.setDiscoverySpi(new TcpDiscoverySpi().setIpFinder(ipFinder));
return cfg;
}
Why this code works:
- It dynamically discovers Ignite cluster members within a Kubernetes namespace.
- You no longer have to hardcode IPs or deal with nodes dropping out-of-sync when pods are rescheduled.
Exposing Ignite Services
Your Ignite nodes are up, but you likely need to expose some services to other applications. Kubernetes services can help here. An example Kubernetes Service to expose Ignite might look like this:
apiVersion: v1
kind: Service
metadata:
name: ignite-service
spec:
type: ClusterIP
selector:
app: spring-ignite
ports:
- port: 47500
name: communication
- port: 47100
name: discovery
Why this service works:
- It exposes the necessary Ignite ports using the
ClusterIP
service type, which is accessible within the cluster. - It selects pods based on labels, ensuring traffic is routed to the appropriate pods.
Persistent Volumes
In a distributed database like Ignite, data persistence is often necessary. Kubernetes Persistent Volumes (PV) can be used to provide a piece of consistent and stable storage despite the ephemeral nature of pods.
Here’s a snippet that includes a Persistent Volume Claim (PVC) in the application’s deployment manifest:
apiVersion: v1
kind: PersistentVolumeClaim
metadata:
name: ignite-pvc
spec:
accessModes:
- ReadWriteOnce
resources:
requests:
storage: 10Gi
---
# Plus the earlier Deployment manifest for brevity
Why using PVs is essential:
- It ensures that your Ignite state is not lost when a pod dies.
- The PVC will dynamically provision PVs as needed.
Monitoring and Health
Finally, don't forget monitoring and health checks. Kubernetes can use liveness and readiness probes to know when to restart a container (liveness) and when a container is ready to serve traffic (readiness).
Here’s how to add a health check to your deployment manifest:
spec:
containers:
- name: spring-ignite
image: your-registry/spring-ignite-app:latest
livenessProbe:
httpGet:
path: /actuator/health/liveness
port: 8080
readinessProbe:
httpGet:
path: /actuator/health/readiness
port: 8080
# ... other settings
Why liveness and readiness probes are important:
- They help Kubernetes manage your applications’ lifecycle more intelligently.
- They prevent traffic from being routed to pods that are not ready to handle it.
Conclusion
Deploying a Spring Boot application with Apache Ignite on Kubernetes can be complex, but with the right approach and understanding of the underlying technologies, it becomes a structured process. Remember to containerize efficiently, adapt your application configuration for Kubernetes environments, carefully expose your services, persist your data appropriately, and implement robust monitoring. Facing these deployment woes head-on will undoubtedly lead to a more stable, scalable, and high-availability system.
While this post covers the essentials for getting started, always consult the official Kubernetes documentation and the Apache Ignite documentation for more complex scenarios and best practices. Happy deploying!