Unlock Auditing Woes: Mastering MongoDB in Spring Data!
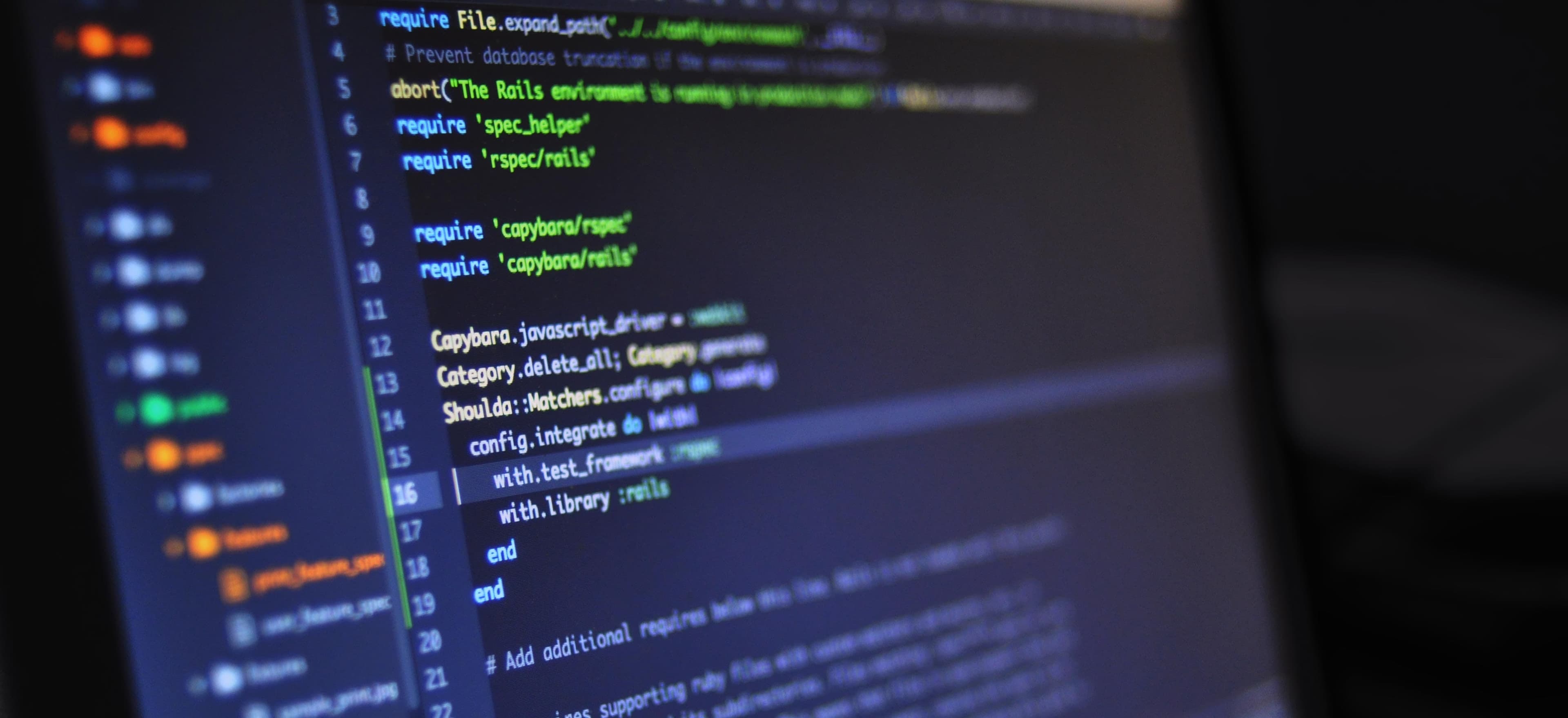
- Published on
Unlock Auditing Woes: Mastering MongoDB in Spring Data!
In the dynamic world of web development, the ability to track and audit changes in your application's data can be as crucial as the data itself. Whether it's for compliance, debugging, or just keeping a record of who did what and when, auditing is essential. If you're a Java developer using Spring Data with MongoDB, you have a powerful toolkit at your disposal to make auditing a breeze. In this post, we'll unveil how to master MongoDB auditing within the Spring Data ecosystem.
Why Auditing Matters
Imagine finding discrepancies in your data without any trail of the events that led there. Without auditing, you'd be left in the dark. Auditing allows you to:
- Keep a historical record of changes.
- Monitor user activities.
- Improve accountability and security.
- Assist in debugging and monitoring.
Spring Data's auditing feature automates the process of keeping these records, ensuring that no significant event slips through the cracks.
Enabling Auditing in Spring Data MongoDB
Spring Data MongoDB provides annotation-based support to automatically persist the audit information for any domain object. However, we'll need to take certain steps to enable and customize auditing.
Step 1: Enable Auditing
First, you need to add the @EnableMongoAuditing
annotation to your configuration class. This tells Spring Data MongoDB to look for the auditing annotations within your domain classes.
@Configuration
@EnableMongoAuditing
public class MongoConfig {}
Step 2: Annotate Domain Classes
Next, annotate the fields in your domain classes with @CreatedDate
, @LastModifiedDate
, @CreatedBy
, and @LastModifiedBy
to capture the necessary audit information.
Here is a sample MongoDB document with auditing fields:
@Document
public class Product {
@Id
private String id;
private String name;
@CreatedDate
private Instant createdDate;
@LastModifiedDate
private Instant lastModifiedDate;
@CreatedBy
private String createdBy;
@LastModifiedBy
private String lastModifiedBy;
// Standard getters and setters
}
Step 3: Capture Auditor Information
Now that the fields are ready, we need a way to capture the "current auditor," which is typically the current user. Implement AuditorAware
interface in a new class.
@Component
public class AuditorAwareImpl implements AuditorAware<String> {
@Override
public Optional<String> getCurrentAuditor() {
// Your logic to fetch the current user
String currentUser = fetchCurrentUser();
return Optional.ofNullable(currentUser);
}
}
In a real-world scenario, the fetchCurrentUser()
method would usually retrieve the authenticated user's identity from the security context.
Step 4: Use Java Configuration
To complete the setup, provide an AuditorAware
bean in your Java configuration. Spring Data will use this bean to fetch the current auditor.
@Configuration
@EnableMongoAuditing(auditorAwareRef = "auditorAware")
public class MongoConfig {
@Bean
public AuditorAware<String> auditorAware() {
return new AuditorAwareImpl();
}
}
Spring Data Auditing in Action
Now that we have set up auditing let's see how it seamlessly integrates into your everyday data operations.
When you save a domain object:
Product product = new Product();
product.setName("Green Tea");
productRepository.save(product);
Spring Data MongoDB will automatically populate the createdDate
, lastModifiedDate
, createdBy
, and lastModifiedBy
fields. When you update the same object:
product.setName("Organic Green Tea");
productRepository.save(product);
It will update the lastModifiedDate
and lastModifiedBy
fields, leaving the createdDate
and createdBy
untouched.
Best Practices for Auditing
To get the most out of auditing, there are a few best practices you should adhere to:
- Keep Audit Fields Immutable: Don't allow direct modification of auditing fields through public setters or constructors.
- Use Proper Indexing: If you frequently query by auditing fields, ensure they are properly indexed.
- Secure Auditing Information: Ensure that access to auditing information is secure and restricted to authorized users.
- Consistency Across Services: If you have a microservices architecture, keep auditing consistent across different services.
Handling Complex Auditing
While the basics of auditing involve tracking "who" and "when," you might sometimes need to record "how" and "why" changes were made. This can involve complex event sourcing or additional auditing frameworks like Javers or Envers.
However, for complex use cases, the advice is to evaluate your requirements carefully and determine whether Spring Data's auditing features suffice or if there's a need for a more comprehensive auditing approach.
Conclusion
Auditing is a powerful feature that shouldn't be an afterthought in application design. By mastering MongoDB auditing in Spring Data, you equip yourself with a set of techniques that not only bolster the integrity of your application but also provide valuable insights into its usage and evolution.
Remember to review the official Spring Data MongoDB documentation for a deep dive into its auditing capabilities. Incorporating the simple yet robust auditing mechanisms we discussed will give you a peace of mind, knowing that your application's data changes are being tracked diligently and effortlessly.
With Spring Data MongoDB, your auditing woes are now unlocked. Keep your application's data integrity in check, and effortlessly sail through debugging, compliance, and monitoring challenges.
Happy coding, and audit away!