Building Blocks: Crafting a Strong Microservice System
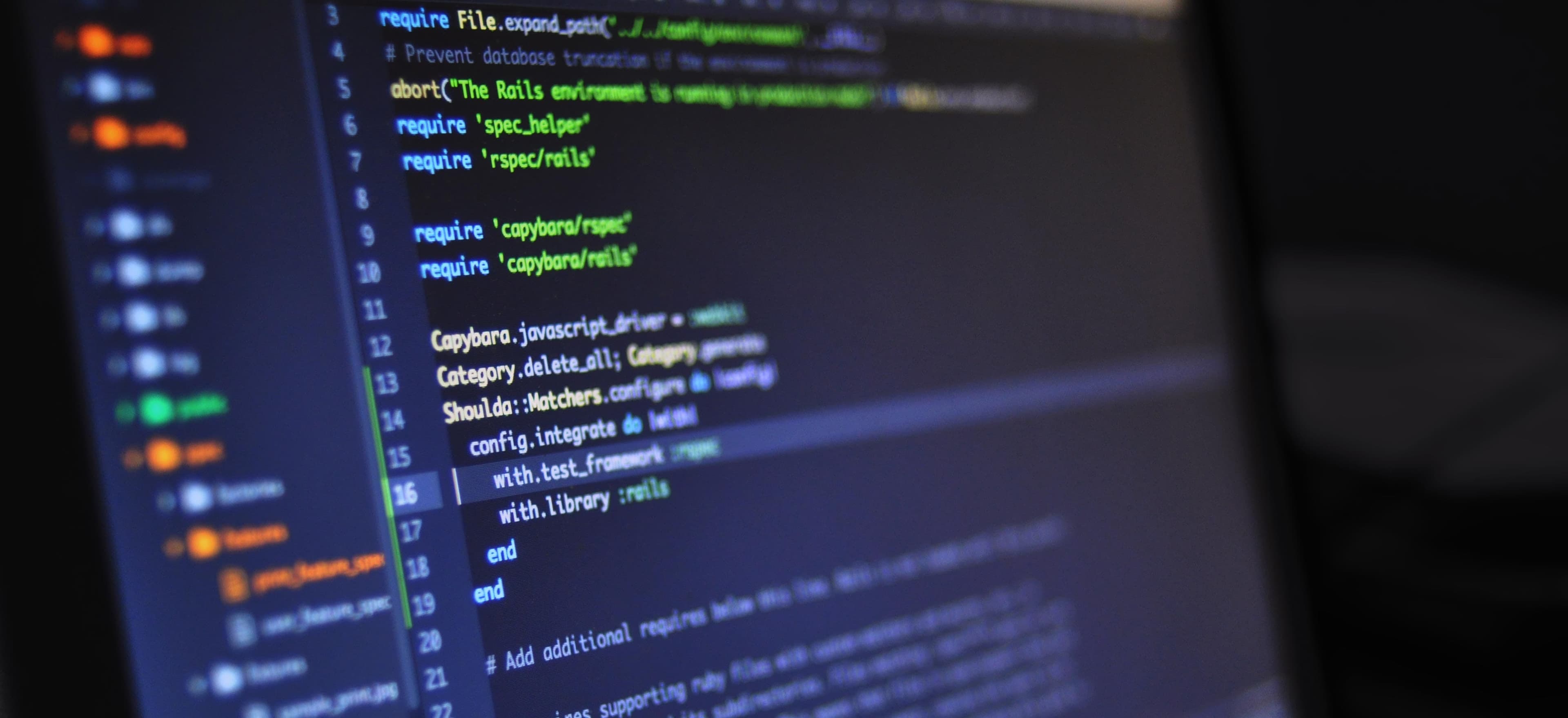
- Published on
Building Blocks: Crafting a Strong Microservice System in Java
In the digital landscape, where scalability, agility, and speed are non-negotiable, microservices architecture has become the cornerstone of modern application development. It diverts from the monolithic approach, offering a suite of independent services that are easier to maintain, scale, and update. Java, known for its robustness, has established itself as one of the preferred languages for building microservices due to its vast ecosystem and mature frameworks.
In this blog post, we'll discuss the key components and best practices to consider when crafting a strong microservice system in Java. We'll also look at some code snippets to help you kickstart your microservice journey.
Understanding Microservices
Microservices are a design approach where applications are built as a collection of loosely coupled services. Each service is responsible for a specific business capability and can be developed, deployed, and scaled independently.
Why go for microservices?
- Scalability: Microservices can be scaled horizontally, allowing you to handle more traffic by adding more service instances.
- Flexibility: They allow teams to work independently on different services using different technologies suited to their service needs.
- Resilience: Failure in one service doesn't bring down your entire application.
- Faster Deployments: Smaller codebases and independent services make it easier to deploy updates faster.
Here's a deeper dive into microservices architecture.
Choosing the Right Java Framework
Java offers several frameworks for building microservices; some of the most popular include Spring Boot, Micronaut, and Quarkus. These frameworks provide the essentials such as inversion of control, dependency injection, and configuration management, making them ideal for microservice development.
@SpringBootApplication
public class ProductServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ProductServiceApplication.class, args);
}
}
Why this code? The above snippet is a Spring Boot application boilerplate. It's annotated with @SpringBootApplication
which denotes it as a configuration class that declares one or more @Bean
methods.
Containerization: Docker and Java
Containerization is a key element of a reliable microservice system. Docker helps package your Java application with all its dependencies into a container, ensuring consistency across multiple development and deployment cycles.
Let's Dockerize a simple Java application:
FROM openjdk:11-jre-slim
COPY ./target/myapp-1.0.jar /usr/app/
WORKDIR /usr/app
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "myapp-1.0.jar"]
Why this code? This Dockerfile
starts with a base Java image, copies your built jar file into the container, sets the working directory, exposes a port for the web server, and defines the entry point as running the jar file.
Service Discovery in a Distributed World
In a microservice architecture, services need to find and communicate with each other. Service discovery mechanisms are pivotal, with options like Netflix’s Eureka or HashiCorp's Consul offering dynamic service registration and discovery.
@EnableEurekaClient
@SpringBootApplication
public class InventoryServiceApplication {
public static void main(String[] args) {
SpringApplication.run(InventoryServiceApplication.class, args);
}
}
Why this code? By annotating our Spring Boot application with @EnableEurekaClient
, you automatically register the service with Eureka, allowing other services to discover it.
Inter-Service Communication: Sync vs Async
Microservices communicate with each other using either synchronous protocols like HTTP/REST, or asynchronous messaging systems like Apache Kafka or RabbitMQ. Synchronous communication is straightforward but can lead to tight coupling, while asynchronous methods can decouple services but add complexity.
Here's how you might use a REST client in Spring Boot:
@RestController
public class ProductController {
private final RestTemplate restTemplate;
public ProductController(RestTemplateBuilder restTemplateBuilder) {
this.restTemplate = restTemplateBuilder.build();
}
@GetMapping("/products/{id}")
public Product getProduct(@PathVariable String id) {
ResponseEntity<Product> response = restTemplate.getForEntity(
"http://inventory-service/inventory/" + id,
Product.class
);
return response.getBody();
}
}
Why this code? The RestTemplate
is a synchronous client that makes HTTP requests to other services. In this snippet, we use it to call the inventory service and retrieve product details.
API Gateway: The Entrance to Your Microservices
An API Gateway acts as the single entry point for all clients. It can handle cross-cutting concerns such as authentication, SSL termination, and request routing. Zuul and Spring Cloud Gateway are popular choices in the Java ecosystem.
@Bean
public RouteLocator myRoutes(RouteLocatorBuilder builder) {
return builder.routes()
.route(p -> p
.path("/product/**")
.uri("http://product-service"))
.route(p -> p
.path("/inventory/**")
.uri("http://inventory-service"))
.build();
}
Why this code? Here, we define routes for a Spring Cloud Gateway instance. This way, incoming requests for products or inventory are forwarded to the appropriate microservices.
Data Management for Microservices
Each microservice should own its data model and database to ensure loose coupling. Choose your data store based on the service's needs—SQL for transactional data, NoSQL for hierarchical, or event sourcing for audit logging and replay.
For example, you might use JPA to map your service's data:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
// getters and setters omitted for brevity
}
Why this code? The @Entity
annotation marks this class as an object that needs to be persisted in the database, and @Id
signifies the primary key of an entity.
Observability and Monitoring
You need to monitor your microservices to ensure they are performing as expected. Use tools like Prometheus for monitoring and Grafana for visualization to keep an eye on metrics, logs, and traces across all your services.
Integrate with Spring Boot Actuator to expose metrics:
management:
endpoints:
web:
exposure:
include: "*"
Why this code? This YAML configuration snippet is for a Spring Boot application's application.properties
file, telling Spring Boot Actuator to expose all its built-in endpoints for monitoring purposes.
Resilience: Handling Failures Gracefully
Expect failures in a microservices environment. Implement patterns like circuit breakers, bulkheads, and retries. Libraries like Resilience4j provide these patterns specifically tailored for Java applications.
@Retry(name = "productService", fallbackMethod = "fallback")
public Product findProductById(String id) {
// ...
}
public Product fallback(String id, Throwable e) {
return new Product(id, "Default Product");
}
Why this code? This uses Resilience4j's retry mechanism with a fallback method to ensure the application can handle failures by retrying or returning a default value.
Conclusion
Crafting a strong microservice system in Java requires a deep understanding of its building blocks and best practices. From choosing the right framework and containerization to mastering service discovery, communication, and resilience, there's no one-size-fits-all approach. The key is to understand the strengths and limitations of the Java ecosystem and apply them to construct a system that meets your needs.
By following these guidelines and leveraging the power of Java, developers can design systems that are not only efficient and robust but are also capable of evolving with changing business requirements.
Do remember that every decision you make in your architecture can have far-reaching effects on your application’s performance, scalability, and maintainability. Happy coding!
Note: The code snippets provided are simplified examples to demonstrate specific concepts.
For more comprehensive tutorials and documentation, explore the following resources:
- Spring Boot Microservices Guide
- Building Microservices with Micronaut
- Creating Supersonic Java Applications with Quarkus