Mastering TestNG & Maven Setup: A Step-by-Step Guide
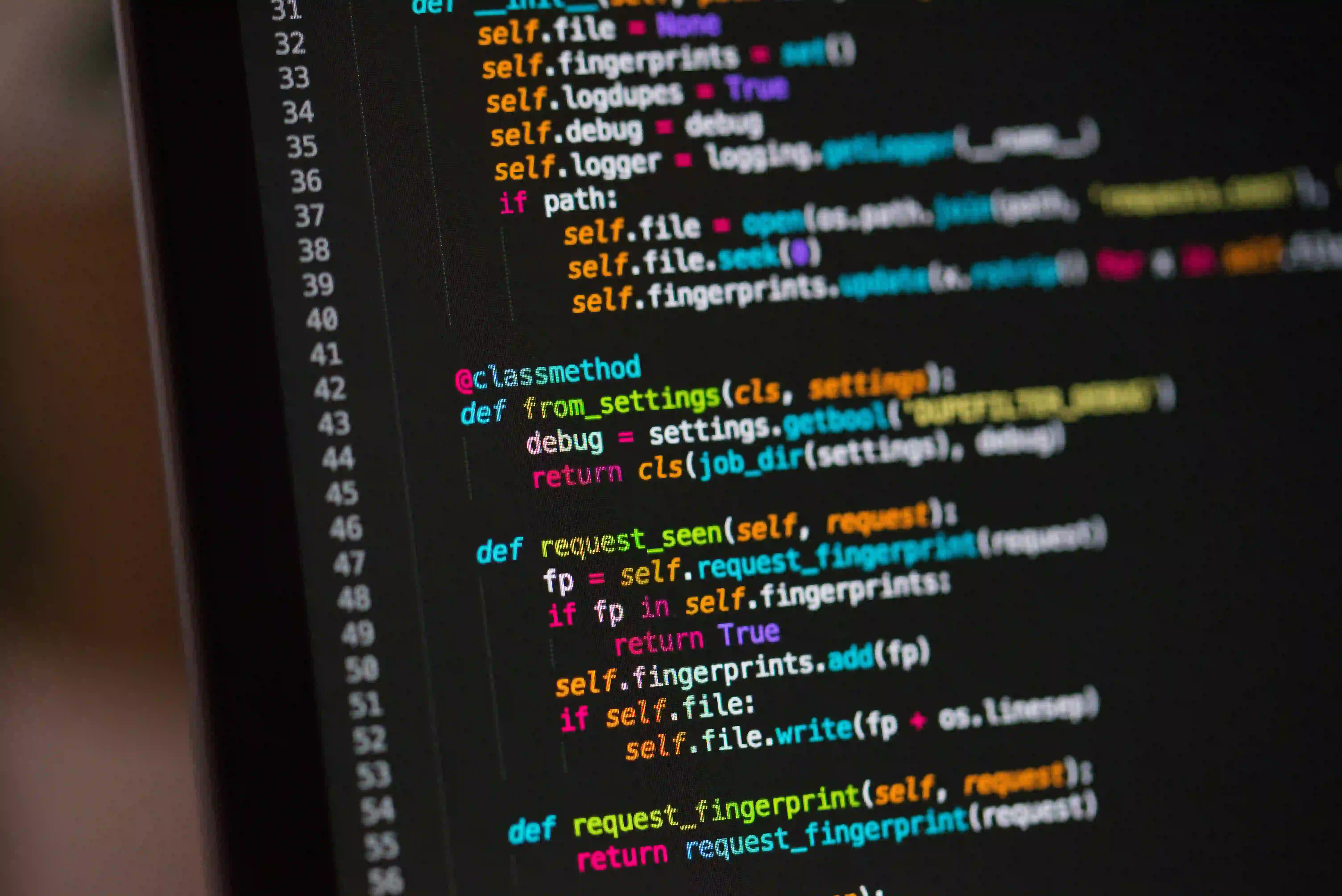
title: "Mastering TestNG & Maven Setup: A Step-by-Step Guide" description: "Learn how to integrate TestNG with Maven to streamline your testing process. This guide provides a walk-through to setup TestNG & Maven with examples & best practices." author: "[Your Name]" date: "[Today's Date]" keywords: "TestNG, Maven, Java, Testing, Setup guide, Tutorial, Integration, Test automation"
Mastering TestNG & Maven Setup: A Step-by-Step Guide
Test automation is a critical aspect of any successful software development project. Among the plethora of tools available for Java developers, TestNG and Maven stand out for their power and flexibility. In this guide, we'll dive into how to integrate TestNG with Maven to create a robust testing framework that can accelerate your development workflow.
What is TestNG?
TestNG is a testing framework inspired by JUnit and NUnit, but introducing new functionalities that make it more powerful and easier to use. It offers advanced features like annotations, grouping, sequencing, and parametrization of tests.
What is Maven?
Maven is a build automation tool used primarily for Java projects. It addresses build lifecycle management, dependency resolution, and project building. Its use of conventions and XML-based project configuration (Project Object Model or POM) simplifies the setup and management of complex projects.
Integrating TestNG with Maven can greatly improve the efficiency of compiling, running, and reporting on your test suites. So, let's get started.
Prerequisites
To get the most out of this guide, you should be familiar with basic Java programming and understand the fundamentals of automated testing and build processes.
Setting Up Maven with TestNG: The Essentials
To kick off our integration, we need to set up a basic Maven project and configure TestNG.
Initializing a New Maven Project
Start by creating a new directory for your project and initialize a Maven project with the following command:
mvn archetype:generate -DgroupId=com.yourcompany.app -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Replace com.yourcompany.app
with your group ID and my-app
with your artifact ID. This generates a project structure with a pom.xml
file that we will customize in the next step.
Edit the pom.xml
To integrate TestNG, add the TestNG dependency within the <dependencies>
section of your pom.xml
file.
<dependencies>
<!-- TestNG Dependency -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.5</version>
<scope>test</scope>
</dependency>
</dependencies>
Make sure to use the latest version of TestNG, which at the time of this writing is 7.5, by checking the TestNG Maven repository.
The <scope>test</scope>
tag is critical here - it tells Maven that this dependency is only for test compilation and execution phases.
Directory Structure
Next, ensure your project adheres to Maven's standard directory layout:
my-app/
|-- src/
|-- main/
|-- java/
|-- resources/
|-- test/
|-- java/
|-- resources/
The src/main/java
directory should contain your application code, while src/test/java
will house your TestNG test classes.
Creating a TestNG Test Case
Now, let's write a simple test case. Under src/test/java/com/yourcompany/app
, create a file named AppTest.java
.
package com.yourcompany.app;
import org.testng.Assert;
import org.testng.annotations.Test;
public class AppTest {
@Test
public void exampleTest() {
int a = 1;
int b = 2;
Assert.assertEquals(a + b, 3, "The addition result should be 3");
}
}
The @Test
annotation from TestNG marks the exampleTest()
method as a test method.
Running Tests with Maven
To run your tests, you can use the following command:
mvn test
Maven will compile your application and test code, then TestNG will execute all methods annotated with @Test
.
TestNG Suite Configuration
For complex scenarios, TestNG allows you to define suites in an XML file. You can specify which classes, methods, and groups to include or exclude from a test run.
Create a file named testng.xml
at the root of your project:
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd">
<suite name="MySuite">
<test name="MyTest">
<classes>
<class name="com.yourcompany.app.AppTest"/>
</classes>
</test>
</suite>
You can then tell Maven to use this suite file by adding a build plugin configuration in your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<suiteXmlFiles>
<suiteXmlFile>testng.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
Make sure the maven-surefire-plugin version is compatible with your TestNG version.
Running the Test Suite
To execute the newly configured test suite:
mvn test
Maven will consider the testng.xml
configuration and run your tests accordingly.
Advanced TestNG Features
TestNG's real power shines when you start using its advanced features like:
- Annotations: Provide metadata and test management capabilities (e.g.,
@BeforeSuite
,@AfterMethod
). - Parameterized tests: Allow tests to run with different sets of data.
- Grouping: Define groups of tests to run or exclude from a run.
- Dependent methods: Specify test methods that depend on others, controlling the order of execution.
Let’s implement a dependent test method to illustrate the power of annotations.
package com.yourcompany.app;
import org.testng.Assert;
import org.testng.annotations.Test;
public class MoreAppTests {
@Test(groups = {"init"})
public void setupEnvironmentTest() {
// Set up your test environment here
Assert.assertTrue(true); // Assume the environment is set up correctly
}
@Test(dependsOnGroups = {"init"})
public void coreFunctionalityTest() {
// This test method depends on the 'init' group
// It will only run if setupEnvironmentTest passes
Assert.assertEquals("A" + "B", "AB"); // Simple concatenation test
}
}
Using groups and dependent methods ensures that tests are run in a specific order and that core functionality tests are only run if the setup is successful.
Conclusion
In this guide, we've covered the basics of setting up TestNG with Maven for Java test automation. The combination of Maven's build capabilities and TestNG's testing power can significantly streamline the software development process. Remember to keep your pom.xml
updated with the latest versions of your dependencies, and take full advantage of TestNG’s features to write comprehensive tests. With practice, these tools will become an indispensable part of your Java development toolkit.
Further Reading
For those who want to delve deeper, here are some resources:
- TestNG Official Documentation: Learn all about TestNG's features and best practices.
- Maven Getting Started Guide: Understand the fundamentals of Maven project setup and configuration.
- Maven Central Repository: Search for the latest libraries and their Maven dependencies.
Testing is a vast topic, and mastery comes with experience and continuous learning. Happy testing, and may your builds always be green!